Question
HeapSort C++ Problem Description Implementing Heaps using an array Write a HeapSort. It will require the use of a reHeapDown function. Your heap sort must
HeapSort C++
Problem Description
Implementing Heaps using an array
Write a HeapSort. It will require the use of a reHeapDown function. Your heap sort must follow the algorithm shown.
the reHeapDown function will be supplied
The code given also requires a list function for the array, and a swap function to be written. You MUST USE the provided function headers, so that the parameter list does not change.
You need to show the HeapSort works with an array.
Here is the algorithm for reHeapDown:
reHeapDown(heap, root, bottom)
if heap element[root] is not a leaf
Set maxChild to index of child with larger value
if heap element[root] < heal element[maxChild]
Swap(heap elements[root], heap lements[maxChild]
reHeapDown(heap, maxChild, bottom
Here is the algorithm for HeapSort
for index going from last node up to next to root node.
Swap data in root node with values[index]
reHeapDown(values, start, end-1)
modify all the funtions to use a template.
Heres what a Swap header looks like that uses a template:
template
void Swap(T * elements, int x, int y)
Demonstrate that the template works with something other than an int array.
Hint:
Get ReHeapDown to work by writing the list and swap functions. Test it!!
Now write a HeapSort function and Test!
code given below
// ReHeapDown.cpp : Defines the entry point for the console application.
//
#include "stdafx.h"
#include
#include
using namespace std;
void listArr(int* arr, int start, int end);
void reHeapDown(int * elements, int root, int bottom);
void Swap(int * elements, int x, int y);
int main()
{
/* consider the situation where the top of the heap was removed
and the right most child on the lowest leveel replaced it */
int heap[6] = {2, 8, 9, 5, 3, 4 };
cout << "Listing semi-heap ";
listArr(heap, 0, 5);
cout << "Try reHeap ";
reHeapDown(heap, 0, 5);
listArr(heap, 0, 5);
return 0;
}
/**
@pre int array
@post array displayed
@param arr address of an int array
@param start int starting point of array
@param end int ending point of array
*/
void listArr(int* arr, int start, int end)
{
// Please write your code. for the display
}
/**
@pre integer array
@post array displayed
@param arr address of an int array
@param start int starting point of array
@param end int ending point of array
reHeapDown(heap, root, bottom)
if heap element[root] is not a leaf
Set maxChild to index of child with larger value
if heap element[root] < heal element[maxChild]
Swap(heap elements[root], heap lements[maxChild]
reHeapDown(heap, maxChild, bottom
*/
void reHeapDown(int * elements, int root, int bottom)
{
int maxC, rightC, leftC; // maxChild, rightChild, leftChild
leftC = root * 2 + 1;
rightC = root * 2 + 2;
if (leftC <= bottom)
{
if (leftC == bottom)
maxC = leftC;
else
{
if (elements[leftC] <= elements[rightC])
maxC = rightC;
else
maxC = leftC;
}
if (elements[root] < elements[maxC])
{
Swap(elements, root, maxC);
reHeapDown(elements, maxC, bottom);
}
}
}
/**
@pre int array
@post array two items swapped
@param arr address of array
@param x int subscript to be swapped
@param y int subscript to be swapped
swap the contents of two elements of an array
*/
void Swap(int * elements, int x, int y)
{
// Please write your code. for the swap
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
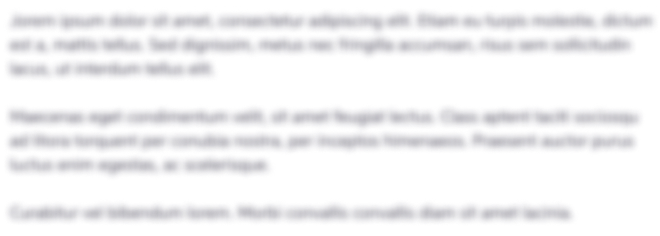
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started