Question
Hello, Can someone help me to solve this project. Please, see the bottom for projcet #1 codes. Your goal for this project is take the
Hello, Can someone help me to solve this project. Please, see the bottom for projcet #1 codes.
Your goal for this project is take the provided code and improve upon it. The code provided is Mr. Kerlin's solution to the Project #1 problem. Start by loading up this code and running it. Read through the input and the java code. Trace out parts of the code you aren't clear on. Try to figure out what each part of the code is doing, how and why. Note that there are no Java Doc comments on the methods. This is because in the real world, you will likely get some undocumented code to read through and try to understand, so it makes sense for you to get some practice with this skill now.
When you feel like you have a good grasp at what Mr. Kerlin's code is doing and how it works, it is time to take this project to the next step and update this code to do a better job of simulating an Olympics.
DRIVER CHANGES:
Read event data from a file (Events.txt is included below as a sample...The version used for testing will have between 3 and 300 events) -- Format of the file is:
Event Name%Venue%EventType
For every event in the event file:
Create a new event
Create 8 to 32 (use a Random object!) athletes who can compete in the event. Each Athlete should be generated by:
Selecting a random name from the provided file of names (Provided by an old US Census)
Randomly select country (use the enum's method)
Randomly set skill (1 to 10 inclusive)
Randomly set any subclass specific skills based on the ranges listed in the subclass's section of this specification
Load event with an array of the Athletes you just created
Run the Event's compete() method and print the Event name and winner of the event
ATHLETE CHANGES:
Get ride of specialty variable. Instead create sub-classes for each type of Athlete. See below for the unique characteristics for each Athlete sub-class. (NOTE that we won't be implementing ALL types of Athlete, just the selected ones described below)
AlpineSkier Object:
Has attribute for risk taking (number between 0 and 100)
getSkill() should return (skill + ((random number from 1 to 1000) % (risk taking)))
Needs a toString() method which should return a String containing all Athlete data and AlpineSkier data
CrossCountrySkier Object:
Has attribute for endurance (number between 0.0 and 1.0 (note this is a decimal!))
getSkill() should return (skill + (100 * endurance))
Needs a toString() method which should return a String containing all Athlete data and CrossCountrySkier data
FreeStyleSkier Object:
Has attribute for style (number between 0.0 and 10.0 (note this is a decimal!))
getSkill() should return (skill + (1 * style))
Needs a toString() method which should return a String containing all Athlete data and FreeStyleSkier data
Bobslider Object:
Has attribute for mass (number between 50 and 300)
getSkill() should return (skill + (.5 * mass))
Needs a toString() method which should return a String containing all Athlete data and Slider data
GENERAL CHANGES:
You will need to refactor (update/improve) this simulation to address these concerns. In addition to your final code, you will need to submit a document describing:
Your approach to solving/addressing the project
Why you did what you did
How effective you think your solutions were
What you would have liked to do better
Updated UML class diagrams for Athlete and each of the Athlete subclasses
-------------------------------------------------------------
Grading Breakdown:
Driver Code: 20 points
Athlete generation (10 points)
Event loop (10 points)
Athlete Refactoring: 10 points
Subclasses: 60 points, each subclass is 15 points distributed as:
Attribute (2 points)
get method for attribute (2 points)
set method for attribute, make sure to correctly validate input is in correct range! (3 points)
Correctly overriding toString() method (2 points)
Correctly overriding getSkill() method (3 points)
JavaDoc of methods and class (3 points)
Document describing how you addressed the concerns (10 points)
-------------------------------------------------------------------------------------------------
Athlete class
import java.util.Objects;
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
/** * Athlete Object * @author kerlin */ public class Athlete { private String name = "DEFAULT"; private Country homeNation = Country.Olympic; private EventType specialty = EventType.Unknown; private int skill = 0; private int goldMedals = 0;
public Athlete() { }
public Athlete(String inName, Country inHomeNation, EventType inSpecialty, int inSkill, int inGoldMedals) { setName(inName); setHomeNation(inHomeNation); setSpecialty(inSpecialty); setSkill(inSkill); setGoldMedals(inGoldMedals); }
public String getName() { return name; }
public void setName(String inName) { if (inName.length() > 0) name = inName; else name = "DEFAULT"; }
public Country getHomeNation() { return homeNation; }
public void setHomeNation(Country inHomeNation) { homeNation = inHomeNation; }
public EventType getSpecialty() { return specialty; }
public void setSpecialty(EventType inSpecialty) { specialty = inSpecialty; }
public int getSkill() { return skill; }
public void setSkill(int inSkill) { if (inSkill >= 0 && inSkill <= 10) skill = inSkill; else skill = 0; }
public int getGoldMedals() { return goldMedals; }
public void setGoldMedals(int inGoldMedals) { if (inGoldMedals > 0) goldMedals = inGoldMedals; else goldMedals = 0; }
@Override public String toString() { return "Athlete{" + "name=" + name + ", homeNation=" + homeNation + ", specialty=" + specialty + ", skill=" + skill + ", goldMedals=" + goldMedals + '}'; }
@Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null) { return false; } if (! (obj instanceof Athlete)) { return false; } if (getSkill() != ((Athlete) obj).getSkill()) { return false; } if (getGoldMedals() != ((Athlete) obj).getGoldMedals()) { return false; } if (!(getName().equals(((Athlete) obj).getName()))) { return false; } if (getHomeNation() != ((Athlete) obj).getHomeNation()) { return false; } if (getSpecialty() != ((Athlete) obj).getSpecialty()) { return false; } return true; }
}
--------------------------------------------------------------------------------
Enums for country
import java.util.Random;
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
/** * Olympic Countries * @author kerlin */ public enum Country { Togo, Kenya, Chile, Argentina, Japan, Korea, Italy, Germany, Australia, Tonga, Olympic;
public static Country next() { Random gen = new Random(); return values()[gen.nextInt(values().length)]; }
}
------------------------------------------------------------------------------
Driver Class
import java.util.Random;
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
/** * Run my Olympic Simulator * @author kerlin */ public class Driver {
/** * @param args the command line arguments */ public static void main(String[] args) { EventType[] types = new EventType[5]; String name = "Ath"; String eventName = "Event"; Random gen = new Random(); for (int x = 0; x < types.length;x++) types[x] = EventType.next(); Event[] events = new Event[5]; for (int x = 0; x < events.length; x++) { Athlete[] aths = new Athlete[3]; for (int y = 0; y < aths.length; y++) aths[y] = new Athlete(name + (x) + (y), Country.next(), types[x], gen.nextInt(10) + 1, 0); events[x] = new Event(eventName + x, Venue.next(),types[x], aths); System.out.println(events[x]); System.out.println(); System.out.println(); System.out.println("Winner of " +events[x].getName() + " is: " + events[x].compete()); System.out.println(); System.out.println(); } // TODO code application logic here } }
-------------------------------------------------------------------------------
Class events
import java.util.Random;
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
/** * Event Object * @author kerlin */ public class Event { private String name = "DEFAULT"; private Venue venueName = Venue.Unknown; private EventType thisEvent = EventType.Unknown; private Athlete[] athletes = new Athlete[0];
public Event() { }
public Event(String inName, Venue inVenue, EventType inEvent, Athlete[] listOfAthletes) { setName(inName); setVenue(inVenue); setEvent(inEvent); setAthleteList(listOfAthletes); }
public String getName() { return name; }
public void setName(String inName) { if (inName.length() > 0) name = inName; else name = "DEFAULT"; } public EventType getEvent() { return thisEvent; }
public void setEvent(EventType inEvent) { thisEvent = inEvent; } public Venue getVenue() { return venueName; }
public void setVenue(Venue inVenue) { venueName = inVenue; }
public Athlete[] getAthleteList() { return athletes; } public void setAthleteList(Athlete[] listOfAthletes) { if (listOfAthletes.length < 1) System.out.println("ERROR! NOT ENOUGH ATHLETES!"); else athletes = listOfAthletes; }
@Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null) { return false; } if (! (obj instanceof Event)) { return false; } if (getEvent() != ((Event) obj).getEvent()) { return false; } if (getVenue() != ((Event) obj).getVenue()) { return false; } if (!(getName().equals(((Event) obj).getName()))) { return false; } if (getAthleteList().equals(((Event) obj).getAthleteList())) { return false; } return true; }
@Override public String toString() { String output = "Event{" + "name=" + name + ", venueName=" + venueName + ", thisEvent=" + thisEvent + ", athletes="; for (Athlete a : athletes) output = output + " " + a; return output; }
public Athlete compete() { Random gen = new Random(); int[] scores = new int[athletes.length]; int winner = 0; for (int x = 0; x < athletes.length; x++) { scores[x] = gen.nextInt(100) + 1 + athletes[x].getSkill(); if (scores[winner] < scores[x]) winner = x; } athletes[winner].setGoldMedals(athletes[winner].getGoldMedals()+1); return athletes[winner]; } }
--------------------------------------------------------------------------------------------
Enum Event Type
import java.util.Random;
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
/** * Olympics Event Types * @author kerlin */ public enum EventType { AlpineSkiing, Biathlon, Bobsleigh, CrossCountrySkiing, Curling, FigureSkating, FreestyleSkiing, IceHockey, Luge, NordicCombined, ShortTrackSpeedSkating, Skeleton, SkiJumping, Snowboard, SpeedSkating, Unknown;
public static EventType next() { Random gen = new Random(); return values()[gen.nextInt(values().length -1)]; } }
--------------------------------------------------------------------------
Enum Venue
import java.util.Random;
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */
/** * Olympic Venues * @author kerlin */ public enum Venue { PyeongChangOlympicStadium, AlpensiaBiathlonCentre, AlpensiaCrossCountrySkiingCentre, AlpensiaSkiJumpingCentre, OlympicSlidingCentre, PhoenixSnowPark, JeongseonAlpineCentre, YongpyongAlpineCentre, KwandongHockeyCentre, GangneungCurlingCentre, GangneungHockeyCentre, GangneungIceArena, GangneungOval, Unknown;
public static Venue next() { Random gen = new Random(); return values()[gen.nextInt(values().length-1)]; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
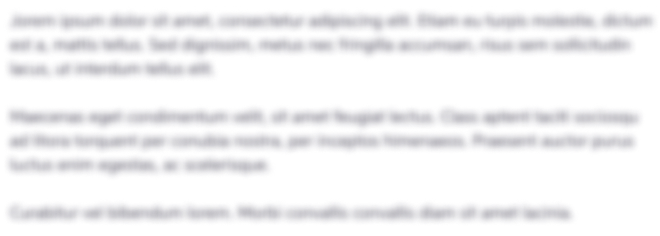
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started