Question
Hello how can help me with this problem . For each of the following algorithms, determine the complexity in Big O notation in as simplified
Hello how can help me with this problem .
For each of the following algorithms, determine the complexity in Big O notation in as simplified a form as possible. Explain your solutions.
-
Naive Fibonacci calculation. State the complexity in terms of n.
public static int simpleFib(int n) { if (n == 0 || n == 1) { return 1; } else { return simpleFib(n - 2) + simpleFib(n - 1); } }
-
Improved Fibonacci calculation. State the complexity in terms of n.
public static int goodFib(int n) { int[] fibs = new int[n + 1]; fibs[0] = 1; fibs[1] = 1; for (int i = 2; i < fibs.length; i++) { fibs[i] = fibs[i - 2] + fibs[i - 1]; } return fibs[n]; }
-
Simple sorting. State the complexity in terms of the length of arr.
public static void sort(int[] arr) { for (int i = arr.length - 2; i >= 0; i--) { for (int j = 0; j <= i; j++) { if (arr[j] > arr[j + 1]) { int tmp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = tmp; } } } }
-
The indexOf(char[] needle, char[] hay) method in the sample solution of assignment 9.3. State the complexity in terms of the lenths of needle and hay.
-
Determining the index of a given number in a sorted array of numbers. State the complexity in terms of the length of hay.
public static int sortedIndexOf(int needle, int[] hay) { int lowerBound = 0; int upperBound = hay.length - 1; while (lowerBound <= upperBound) { int mid = (lowerBound + upperBound) / 2; if (hay[mid] < needle) { lowerBound = mid + 1; } else if (hay[mid] > needle) { upperBound = mid - 1; } else { return mid; } } return -1; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
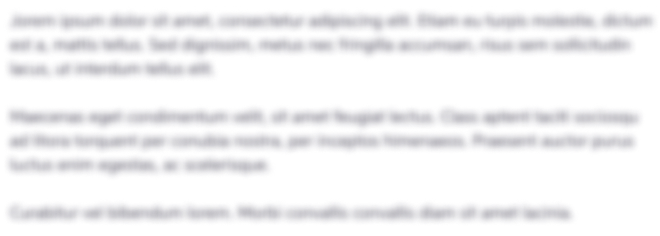
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started