Question
Hello, I am having trouble with the following code that causes the program to crash. I tried debugging and I think the problem is that
Hello, I am having trouble with the following code that causes the program to crash. I tried debugging and I think the problem is that the stack is empty somewhere and the operations for pop/ top are performed, however I don't know where. I am using visual studios. Please help me find the problem so that the code works and evaluates the postfix expression, Thank you for your help in advance
This is displayed:
The code is below:
For main:
#include
#include
#include
#include
#include
#include
#include "EvalBool.h"
using namespace std;
//Function to return precedence of operators
int prec(char);
/*
this function takes in an Infix string expression and turns it into a postfix
expression, it returns the postfix string expression
*/
string InfixToPostfix(string);
// Generates a random boolean expression of length int
string rand_gen_logExp(int);
// This function should receive the string passed from the Postfix function and evaluate the expression, returning true or false
char EvaluateBooleanPostFixExpression(string);
// Operands are T or F, they will be evaluated
bool IsOperand(char);
// The Operators perform the evaluation
bool IsOperator(char);
int main()
{
string expression = "T+!(T+T)*(T)*(!F)*T*T+!T+!T+F+T*!!F*!F*(F+!F*F)*F+F";
string postfix = InfixToPostfix(expression);
cout
cout
cout
return 0;
}
For the header file:
#include
#include
#include
#include
using namespace std;
int prec(char);
string InfixToPostfix(string);
string rand_gen_logExp(int);
char EvaluateBooleanPostFixExpression(string);
bool IsOperand(char);
bool IsOperator(char);
//Function to return precedence of operators
int prec(char c)
{
if (c == '!')
return 3;
else if (c == '*')
return 2;
else if (c == '+')
return 1;
else
return -1;
}
/*
this function takes in an Infix string expression and turns it into a postfix
expression, it returns the postfix string expression
*/
string InfixToPostfix(string Expression)
{
// initialize stack S
stack
// this will serve as the first element of the stack so it wont be empty
S.push('L');
// initialize output string Tokens to be empty
string Tokens;
int l = Expression.length();
// for i = 0 to n do the following cases (there is already an element in the first stack so it is for i =1 to jump it )
for (int i = 0; i { // process the array of Expression[1..n] // If the scanned character is an operand, add it to output string, Tokens. // all operands need to be copied to Tokens first if ((Expression[i] == 'T') || (Expression[i] == 'F')) Tokens += Expression[i]; // If the scanned character is an (, push it to the stack.*** it wont be popped until the closing parenthese else if (Expression[i] == '(') S.push('('); // If the scanned character is an ), pop both ()... eventually, but first all the operators need to be cppied into the output string else if (Expression[i] == ')') { //push all operators of the string to to the stack until an ( is encountered. while (S.top() != 'L' && S.top() != '(') { // is it a (? No? then pop the operator char c = S.top(); S.pop(); //and put it in Tokens Tokens += c; } // when the parentheses close then pop them from the stack if (S.top() == '(') { char c = S.top(); S.pop(); } } else { // pop out the operators from the stack according to their precedence, greatest to least // this is case II in the pseudocode from the powerpoint slide 32 while (S.top() != 'L' && prec(Expression[i]) { char c = S.top(); S.pop(); // The operators popped are according to their precedence, add the operators popped into the ouput string Tokens += c; } S.push(Expression[i]); } } // at the end of the input, Pop all the remaining symbols from the stack to the end of the output while (S.top() != 'L') { char c = S.top(); S.pop(); Tokens += c; } cout return Tokens; } // This function should receive the string passed from the Postfix function and evaluate the expression, returning true or false char EvaluateBooleanPostFixExpression(string x) { // length of expression int n = x.length(); char Op1, Op2, symbol; // initialize a stack to be empty stack // for i = 0 to length n of the Expression do for (int i = 0; i /// if character is an operand(T or F) then push it into the stack as it is scanned if (IsOperand(x[i])) { // push character in the stack Y.push(x[i]); } /*else if the character is an operator, pop two items n1 and n2 from the stack, calculate the op of n1 and n2, and then push the result into the stack*/ else if (IsOperator(x[i])) { switch (x[i]) { // case when character is NOT then pop one element from stack and push it's negation (i.e if !T, then push F into the stack) case '!': // stacks are last in first out, so Operator1 is inserted in the end of the stack // top then pushes the operator into the last element of the stack Op1 = Y.top(); // pop the item Y.pop(); // evaluate the NOT operation,k if (Op1 == 'T') Y.push('F'); // and then push the result into the stack else Y.push('T'); break; // case for AND when * is encountered case '*': // pop n1 and n2 from the stack Op1 = Y.top(); Y.pop(); Op2 = Y.top(); Y.pop(); // evaluate using the Truth operation for AND, and then push the result into the stack if (Op1 == 'T' && Op2 == 'T') Y.push('T'); else Y.push('F'); break; // Case for OR when + is encountered case '+': // pop n1 and n2 from the stack Op1 = Y.top(); Y.pop(); Op2 = Y.top(); Y.pop(); // evaluate the OR Truth operation on the popped elements, then push the result back into the stack if (Op1 == 'F' && Op2 == 'F') Y.push('F'); else Y.push('T'); break; // if there are no sufficient operands report error and exit default: exit(-1); break; // incase of error } } } // output the only remaining item in the stack, the answer to the expression return Y.top(); } // Operands are T or F, they will be evaluated bool IsOperand(char Operand) { if(Operand == 'T' && Operand == 'F') return true; else return false; } // The Operators perform the evaluation bool IsOperator(char Operator) { if(Operator == '!' && Operator == '*' && Operator =='+') return true; else return false; } string rand_gen_logExp(int n) { if (n return ""; //possible symbols in the expression string symbols = "FT()!*+"; //possible symbols after each current symbol string succ[] = { "*+)","*+)", "!FT", "*+)", "FT(!", "FT(!", "FT(!" }; //Ouput string initialized; string logical_exp = ""; /umber of left apparantheses generated so far int num_of_lp = 0; //initializz first symbol of the output char first_symbol = ' '; //randomly generate first symbol in the output //seed for rand srand(time(0)); switch (rand() % 4) { case 0: first_symbol = 'F'; break; case 1: first_symbol = 'T'; break; case 2: first_symbol = '!'; break; case 3: first_symbol = '('; num_of_lp++; break; } logical_exp += first_symbol; // The last symbol generated char last_symbol = first_symbol; // The main loop that generates the rest of the expression for (int i = 1; i { //string s = succ[symbols.indexOf(last_symbol)]; string s = succ[symbols.find(last_symbol)]; char next_symbol; bool again; // genrating the next symbol in the expression and // make sure that it conforms to format of loogical expressions do { again = false; next_symbol = s.at(rand() % (s.length())); switch (next_symbol) { case ')': // All parantheses seen so far matched // ) is not allowed for next symbol if (num_of_lp == 0) { again = true; } else num_of_lp--; break; case '(': num_of_lp++; break; } } while (again); logical_exp += next_symbol; last_symbol = next_symbol; } // check if the last symbol is an operator and hence need an operand if ((last_symbol == '(' || last_symbol == '*' || last_symbol == '+' || last_symbol == '!')) logical_exp += rand() % 2 == 0 ? 'F' : 'T'; // adding necessary closing )'s for (int i = 0; i logical_exp += ')'; return logical_exp; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
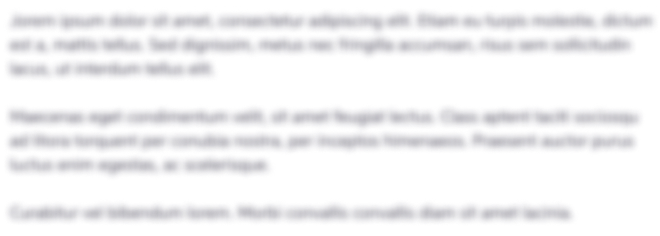
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started