Question
Hello! I need help implementing the methods in the SortedSet class and to create a SetTester class that tests all of the methods. Below, I
Hello! I need help implementing the methods in the SortedSet class and to create a SetTester class that tests all of the methods. Below, I have added the grading rubric, and the 3 classes. The SetInterface should not be modified.
/**
An interface that describes the operations of a set of objects.
@author Frank M. Carrano
@author Timothy M. Henry
@author Charles Hoot
@version 4.0
*/
public interface SetInterface
{
/** Gets the current number of entries in this set.
@return The integer number of entries currently in the set. */
public int getCurrentSize();
/** Sees whether this set is empty.
@return True if the set is empty, or false if not. */
public boolean isEmpty();
/** Adds a new entry to this set, avoiding duplicates.
@param newEntry The object to be added as a new entry.
@return True if the addition is successful, or
false if the item already is in the set. */
public boolean add(T newEntry);
/** Removes a specific entry from this set, if possible.
@param anEntry The entry to be removed.
@return True if the removal was successful, or false if not. */
public boolean remove(T anEntry);
/** Removes one unspecified entry from this set, if possible.
@return Either the removed entry, if the removal
was successful, or null. */
public T remove();
/** Removes all entries from this set. */
public void clear();
/** Tests whether this set contains a given entry.
@param anEntry The entry to locate.
@return True if the set contains anEntry, or false if not. */
public boolean contains(T anEntry);
/** Retrieves all entries that are in this set.
@return A newly allocated array of all the entries in the set. */
public T[] toArray();
} // end SetInterface
XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
import LinkedBag.Node;
/**
* A linked-node implementation of the Set ADT in which elements of the set are
* always sorted (in this case, lexicographically, which is a fancy way of
* saying "alphabetically"). Note that the String class has a compareTo method
* which you should be using to assist you in keeping the set sorted.
*
*/
public class SortedSet implements SetInterface
// +++
private Node head;
private int size;
// +++
public SortedSet() {
head = null;
size = 0;
}
@Override
public int getCurrentSize() {
return size;
}
@Override
public boolean isEmpty() {
return size == 0;
}
// +++
@Override
public boolean add(String newEntry) {
Node newNode = new Node(newEntry);
newNode.next = head;
head.next = newNode;
size++;
return true;
}
@Override
public boolean remove(String anEntry) {
Node temp = head;
if(size
return false;
if(head.next.data.equals(anEntry))
{
remove();
return true;
}
while (temp.next != null) {
if(!temp.next.data.equals(anEntry))
temp = temp.next;
else
{
String data = temp.next.data;
temp.next.data = head.next.data;
head.next.data = data;
remove();
return true;
}
}
return false;
@Override
public String remove() {
Node current = head.next;
if (head.next == null) {
return null;
}
head.next = head.next.next;
size--;
return current.data;
}
@Override
public void clear() {
head = null;
size = 0;
}
@Override
public boolean contains(String anEntry) {
Node temp = head;
while (!temp.next.data.equals(anEntry)) {
temp = temp.next;
if (temp.next == null) {
return false;
}
}
return true;
}
/*
* returns a string representation of the items in the bag, specifically a space
* separated list of the strings, enclosed in square brackets []. For example,
* if the set contained cat, dog, then this should return "[cat dog]". If the
* set were empty, then this should return "[]".
*/
@Override
public String toString() {
String result = "";
Node current = head;
while (current.next != null) {
result = result + current.next.data + " ";
current = current.next;
}
return result;
}
// +++
@Override
public String[] toArray() {
@SupressWarnings("unchecked")
String[] result = (String[])new Object[size];
int index = 0;
Node currentNode = head;
while((index
result[index] = currentNode.data;
index++;
currentNode = currentNode.next;
}
return result;
}
// +++
private class Node {
private String data;
private Node next;
private Node(String data) {
this.data = data;
}
private Node(String data, Node nextNode) {
this.data = data;
next = nextNode;
}
}
}
XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
public class SetTester {
SortedSet sortedSet = new SortedSet();
sortedSet.add("dog");
sortedSet.add("tree");
sortedSet.add("cat");
System.out.println("Expecting to see [cat dog tree]");
System.out.println("Result:" + sortedSet.toString());
sortedSet.remove("dog");
System.out.println("Removing dog and expecting to see [cat tree]");
System.out.println("Result:" + sortedSet.toString());
}
Outcomes: Implement an ADT (specifically a linked-node based implementation of the Set ADT in which all elements of the set are kept sorted) Test a class Naming requirements (not following any of these may result in a score of 0) You have been provided two source code files that you must download and put in your project: o SetInterface.java, which should not be changed in ANY WAY. o SortedSet.java, which is where you will write most of your code In addition, you will write a third file, SetTester.java, which you will use to test your SortedSet (following the same guidelines as in project2). Use the default package (this means there should be no package statements in any of your files). The above three.java files should be the only source code files in your project. Your assignment is to: 1. Implement the SortedSet class using linked nodes a. Create a private inner class named Node within the SortedSet class. b. At all times, the set should be in sorted order. So, if a new item is inserted, or items are removed, it is important to make sure that the set is still sorted after these operations. Do not use an array or any other Java data structures to help you sort. That would be very inefficient. You ought to be able to keep the data sorted without creating an extra array or list or map or table or an extra chain of linked nodes. The only instance variables should be a node representing the start, or head, of the nodes, and an int representing the size of the set. There should be one constructor: A no-parameter constructor that creates an empty set. SetTester should thoroughly test the methods and constructor in the Sorted class. It should utilize the main method and helper methods to print to the screen what is being tested, what results are expected, and then show the actual results. This should not involve any interaction from the user. Do not ask the user to enter input. Just run test cases. Output should look something like this Creating an empty set and adding three items: dog tree cat Expecting to see [cat dog tree] Result [cat dog tree] 2. 3. 4. Removing dog and expecting to see [cat tree] Result [cat tree]Step by Step Solution
There are 3 Steps involved in it
Step: 1
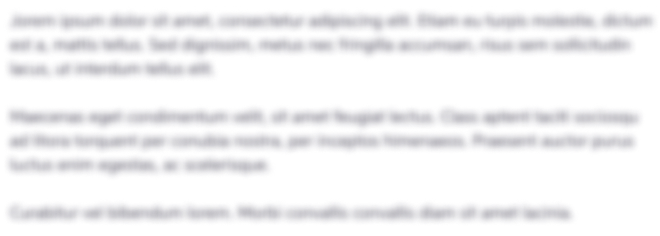
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started