Question
HELLO I NEED HELP TO CONVERT FROM ARRAYLIST TO ARRAYS Take the Helper class of the previous assignment, and change it to use an array
HELLO I NEED HELP TO CONVERT FROM ARRAYLIST TO ARRAYS
Take the Helper class of the previous assignment, and change it to use an array of 10 BestFriend objects, instead of an arrayList of BestFriend objects. Do all of the requirements of the assignment, but simulate "removing" the element by moving all the following elements up to previous index, and then moving nulls to the last element of the array that was moved to a previous index. You may wish to create a new method for this: shrinkArray(). You may need to pass parameter(s) to this method. When the array becomes too small to fit a new BestFriend object, define a new array with double the size of the original array, move everything from the original array to the new array, and then reset the reference of the original array to now point to the new array. You may wish to create a new method for this: expandArray(); You may need to pass parameter(s) to this method. Warning: Null pointer exceptions can occur whenever you try to compare an element in the array to the searchObject. Before comparing, make sure you ask: If (element retrieved from array != null) ...//proceed with the rest of your logic
public class BestFriend {
private String firstName;
private String lastName;
private String nickName;
private String cellPhone;
private String email;
public BestFriend(String firstName, String lastName, String nickName, String cellPhone, String email) {
this.firstName = firstName;
this.lastName = lastName;
this.nickName = nickName;
this.cellPhone = cellPhone;
this.email = email;
}
public BestFriend(String firstName, String lastName, String nickName) {
this.firstName = firstName;
this.lastName = lastName;
this.nickName = nickName;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getNickName() {
return nickName;
}
public void setNickName(String nickName) {
this.nickName = nickName;
}
public String getCellPhone() {
return cellPhone;
}
public void setCellPhone(String cellPhone) {
this.cellPhone = cellPhone;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
@Override
public String toString() {
return "BestFriend{" + "firstName=" + firstName + ", lastName=" + lastName + ", nickName=" + nickName + ", cellPhone=" + cellPhone + ", email=" + email + '}';
}
@Override
public boolean equals (Object obj ) {
BestFriend other;
if (this == obj){
return true;
}
if (obj == null){
return false;
}
if (obj instanceof BestFriend)
{
other = (BestFriend) obj;
}
else{
return false;
}
if (firstName.equals(other.firstName) && lastName.equals(other.lastName) && nickName.equals(other.nickName))
return true;
else
return false;
}
}
import java.util.ArrayList;
import java.util.Scanner;
public class BestFriendArrayListHelper {
ArrayList bestFriends;
Scanner scnr;
public BestFriendArrayListHelper()
{
bestFriends = new ArrayList();
scnr = new Scanner(System.in);
}
public void addAFriend()
{
//1. Ask the user for the firstName, LastName, nickName, cellPhone, email, of the friend
//2. Use Scanner object (keyboard) to retrieve the data from the user
//3. Create a bestFriend object
//BestFriend aFriend = new BestFriend(fName, lName, cPhone, email);
//4. add the aFriend object to the arraylist ****put it in aphabetical order.
System.out.println("Enter the First Name of ur friend");
String fname=scnr.nextLine();
System.out.println("Enter the Last Name of ur friend");
String lname=scnr.nextLine();
System.out.println("Enter the Nick Name of ur friend");
String Nname=scnr.nextLine();
System.out.println("Enter the Phone no of ur friend");
String phone=scnr.nextLine();
System.out.println("Enter the email of ur friend");
String email=scnr.nextLine();
BestFriend bff=new BestFriend(fname, lname, Nname, phone, email);
bestFriends.add(bff);
}
public void changeAFriend()
{
//1. call search method
//2. if returnCode == -1, then message to user saying NOT FOUND
//3. else retrieve object in arraylist at the returnCode position, display it
//4. ask user for all the data of the friend
//5. Use the object setters to change the values
//6. Put the objects back into the arraylist using the set method
int i=searchAFriend();
if(i==-1)
System.out.println("Not Found");
else {
System.out.println(bestFriends.get(i));
System.out.println("Enter the First Name of ur friend");
String fname=scnr.nextLine();
System.out.println("Enter the Last Name of ur friend");
String lname=scnr.nextLine();
System.out.println("Enter the Nick Name of ur friend");
String Nname=scnr.nextLine();
System.out.println("Enter the Phone no of ur friend");
String phone=scnr.nextLine();
System.out.println("Enter the email of ur friend");
String email=scnr.nextLine();
BestFriend bff=bestFriends.get(i);
bff.setCellPhone(phone);
bff.setEmail(email);
bff.setFirstName(fname);
bff.setLastName(lname);
bff.setNickName(Nname);
}
}
public void removeAfriend()
{
//1. call search method
//2. if returnCode == -1 then message to user saying NOT FOUND
//3. else retrieve object in arraylist at the returnCode position, display it
//4. ask the user, are you sure you want to remove that friend?
//5. If user says YES, remove from the arraylist the object pointed
// at by the returnCode of the search method, & give message
// else another Message
int i=searchAFriend();
if(i!=-1) {
System.out.println(bestFriends.get(i));
System.out.println("are you sure you want to remove that friend?");
String choice=scnr.nextLine();
if(choice.equalsIgnoreCase("yes")) {
bestFriends.remove(i);
System.out.println("Successfully removed");
}
else
System.out.println("Operation Aborted");
}
else
System.out.println("Not Found");
}
public void displayAFriend() {
System.out.println("Do you want to display one friend or All (One/ALL)");
String choice=scnr.nextLine();
if(choice.equalsIgnoreCase("all")) {
for (BestFriend bestFriend : bestFriends) {
System.out.println(bestFriend);
}
}else {
int i=searchAFriend();
if(i==-1)
System.out.println("Not Found");
else
System.out.println(bestFriends.get(i));
}
}
public int searchAFriend() {
System.out.println("Enter the First Name of ur friend");
String fname=scnr.nextLine();
System.out.println("Enter the Last Name of ur friend");
String lname=scnr.nextLine();
System.out.println("Enter the Nick Name of ur friend");
String Nname=scnr.nextLine();
BestFriend searchObj=new BestFriend(fname, lname, Nname);
int i=0;
for (BestFriend bestFriend : bestFriends) {
if(bestFriend.equals(searchObj))
return i;
i++;
}
return -1;
}
}
import java.util.Scanner;
public class BestFriendsArrayList {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
System.out.println("Welcome to the Best Friends' Contacts!");
BestFriendArrayListHelper myHelper = new BestFriendArrayListHelper();
int menuSelection;
do {
menuSelection = displayMenu();
switch (menuSelection) {
case 1:
System.out.println("calling add method");
myHelper.addAFriend();
break;
case 2:
System.out.println("calling change method");
myHelper.changeAFriend();
break;
case 3:
System.out.println("calling remove method");
myHelper.removeAfriend();
break;
case 4:
System.out.println("calling display method");
myHelper.displayAFriend();
break;
case 5:
System.out.println("Thanks for using BFF Contacts.");
break;
default:
System.out.println("Sorry, you entered an invalid option. Try Again.");
}
}while (menuSelection != 5);
}
public static int displayMenu()
{
int menuSelection;
Scanner keyboard = new Scanner(System.in);
System.out.println("1. Add a Best Friend");
System.out.println("2. Change a Best Friend");
System.out.println("3. Remove a Best Friend");
System.out.println("4. Display a Best Friend");
System.out.println("5. Quit");
menuSelection = keyboard.nextInt();
return menuSelection;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
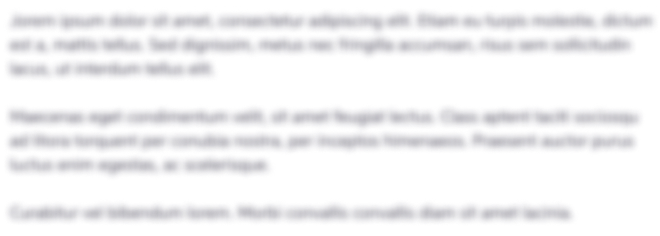
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started