Question
Hello, I need help with implementing my main function. I am having some difficulites in reading each element into an array. Then using the function
Hello,
I need help with implementing my main function. I am having some difficulites in reading each element into an array. Then using the function created to successlly do this. I will attach my main function and also my prototypes. You can make any changes to source code.
Program Description
program must open the binary file threesData.bin and read each element into an array. Once the integers have been read in, you will write one or more functions that sorts the integers in the array into ascending order (low to high.) Your program should sort this array using the Mergesort.
#include
#include
#include
#include "Mergesort.h"
using namespace std;
int main (){
ifstream file;
fstream outfile;
int n=0;
int array[n];
uint32_t a;
file.open("threesData.bin",ios::binary | ios::in);
if (!file)
{
cout << "Error opening file ";
}
int count = 0;
while (!file.eof())
{
file.read(reinterpret_cast
count++;
count = array[n];
}
file.close();
MergeSort(array,0,n-1);
// print the array elements to file
for(int i=0;i { outfile.open("sortedThreesData.bin", ios::out | ios::binary); outfile.write(reinterpret_cast outfile.close(); } return 0;} I dont include an array[i] which I believe stores the elements, does my above main function do this, if not please let me know what I could do to change this. My outfile.write, am I using the correct arguments for the mergesort function to be working. .h #ifndef MERGESORT_H_INCLUDED #define MERGESORT_H_INCLUDED void Merge(int *a, int low, int high, int mid); void MergeSort(int *a, int low, int high); void SelectionSort(int *a, int low,int high); void swap(int *x, int *y); #endif // MERGESORT_H_INCLUDED Function prototypes #include "Mergesort.h" void Merge(int *a, int low, int high, int mid) { // We have low to mid and mid+1 to high already sorted. int i, j, k, temp[high-low+1]; i = low; k = 0; j = mid + 1; // Merge the two parts into temp[]. while (i <= mid && j <= high) { if (a[i] < a[j]) { temp[k] = a[i]; k++; i++; } else { temp[k] = a[j]; k++; j++; } } // Insert all the remaining values from i to mid into temp[]. while (i <= mid) { temp[k] = a[i]; k++; i++; } // Insert all the remaining values from j to high into temp[]. while (j <= high) { temp[k] = a[j]; k++; j++; } // Assign sorted data stored in temp[] to a[]. for (i = low; i <= high; i++) { a[i] = temp[i-low]; } } // A function to split array into two parts. void MergeSort(int *a, int low, int high) { int mid; if (low < high) { mid=(low+high)/2; // Split the data into two half. SelectionSort(a, low, mid); SelectionSort(a, mid+1, high); // Merge them to get sorted output. Merge(a, low, high, mid); } } void SelectionSort(int *a, int low,int high) { int i, j, min_idx; // One by one move boundary of unsorted subarray for (i = low; i <=high; i++) { // Find the minimum element in unsorted array min_idx = i; for (j = i+1; j <= high; j++) if (a[j] < a[min_idx]) min_idx = j; // Swap the found minimum element with the first element swap(&a[min_idx], &a[i]); } } void swap(int *x, int *y) { int tmp = *x; *x = *y; *y = tmp; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
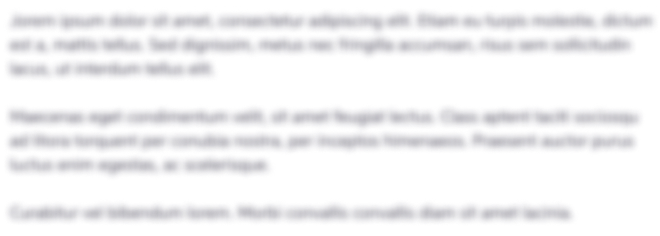
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started