Question
Hello I need some help with my code. I need it to call the Payable class computeAmountToPay(); method to show the computed output. Example: Enter
Hello I need some help with my code.
I need it to call the Payable class computeAmountToPay(); method to show the computed output. Example:
Enter your choice: 1. Add hourly employee 2. Add manager 3. Add sales manager 4. Add bill 5. List all payables 6. Exit 2 Enter the first name. james Enter the last name. turner Enter ID 12 Enter manager's annual salary 12 Enter your choice: 1. Add hourly employee 2. Add manager 3. Add sales manager 4. Add bill 5. List all payables 6. Exit 1 Enter the first name. time Enter the last name. jun Enter ID 234 Enter the hours. 4 Enter the rate 4 Enter your choice: 1. Add hourly employee 2. Add manager 3. Add sales manager 4. Add bill 5. List all payables 6. Exit 5 class edu.seminolestate.employees.Manager [First Name=james, Last Name=turner, Id=12class edu.seminolestate.employees.Manager 0.0 class edu.seminolestate.employees.HourlyEmployee [First Name=time, Last Name=jun, Id=234class edu.seminolestate.employees.HourlyEmployeeHourlyEmployees [ First Name=time, Last Name=jun, ID=234, Pay Rate=4.0, Hours Worked=4.0] Enter your choice: 1. Add hourly employee 2. Add manager 3. Add sales manager 4. Add bill 5. List all payables 6. Exit
THIS SHOULD SHOW HOW MUCH THE HOURLY EMPLOYEE IS TO BE PAYED which is $16. Same for the rest of the options. I have them worked out in the classes but cant figure out how to code this. I THINK I AM HAVING TROUBLE IN THE PRINTEWRITER AREA OF THE APPLICATION.
HERE IS WHAT I HAVE.
package edu.seminolestate.bill; import java.time.DateTimeException; import java.time.LocalDate;
import edu.seminolestate.exceptions.*; import edu.seminolestate.payable.*;
//DelilahPerez public class Bill implements Payable {
private String vendorName; private double amountOwed; private LocalDate dueDate;
public Bill(String newVendorName, double newAmountOwed, LocalDate newDueDate) throws IllegalArgumentException { this.setVendorName(newVendorName); this.setAmountOwed(newAmountOwed); this.setDueDate(newDueDate); }
public String toString() { return getClass() + " [Vendor Name=" + vendorName + ", Amount Owed=" + amountOwed + ", Due Date=" + dueDate; } public String getVendorName() { return vendorName; }
public void setVendorName(String newVendorName) {
if (newVendorName == null || newVendorName.length() < 1) throw new IllegalArgumentException("Vendor name cannot be null or empty."); else this.vendorName = newVendorName; }
public double getAmountOwed() { return amountOwed; }
public void setAmountOwed(double newAmountOwed) { if (amountOwed >=0) this.amountOwed = newAmountOwed; else throw new IllegalArgumentException("Amount Owed must be >= 0."); }
public LocalDate getDueDate() { return dueDate; }
public void setDueDate(LocalDate newDueDate) { if (newDueDate != null) this.dueDate = newDueDate; else throw new IllegalArgumentException("Purchase date cannot be null."); } public void setDueDate(int year, int month, int day) {
try { this.dueDate = LocalDate.of(year, month, day); } catch (DateTimeException e) { System.out.println("Date cannot be set"); } } @Override public double computeAmountToPay() { // TODO Auto-generated method stub return amountOwed; } }
**************
package edu.seminolestate.employees; import edu.seminolestate.payable.*;
public class Manager extends Employee implements Payable{ private double annualSalary;
public Manager(String newFName, String newLName, int newId, double salary) throws IllegalArgumentException { super(newFName, newLName, newId); }
@Override public double computeAmountToPay() { // TODO Auto-generated method stub return annualSalary / 12; }
public double getAnnualSalary() { return annualSalary; }
public void setAnnualSalary(double newAnnualSalary) { if (newAnnualSalary > 0) this.annualSalary = newAnnualSalary; else throw new IllegalArgumentException("Invalid Salary"); }
public String toString() { return super.toString() + getClass() + " " + annualSalary; }
}
*************************
package edu.seminolestate.employees;
import edu.seminolestate.payable.*;
public class SalesManager extends Manager implements Payable { private double salesAmount; private double commissionRate;
public SalesManager(String newFName, String newLName, int newId, double salary, double sales, double rate) throws IllegalArgumentException { super(newFName, newLName, newId, salary); }
public double getSalesAmount() { return salesAmount; }
public void setSalesAmount(double newSalesAmount) { if (newSalesAmount >= 0) this.salesAmount = newSalesAmount; else throw new IllegalArgumentException("Sales Amount is invalid"); }
public double getCommissionRate() { return commissionRate; }
public void setCommissionRate(double newCommissionRate) { if (newCommissionRate >= 0 && newCommissionRate <= 1) this.commissionRate = newCommissionRate; else throw new IllegalArgumentException("Comission rate is invalid"); }
@Override public double computeAmountToPay() { // TODO Auto-generated method stub return super.computeAmountToPay() + salesAmount * commissionRate; }
public String toString() { return super.toString() + getClass() + " " + salesAmount + commissionRate; }
}
***********************
package edu.seminolestate.employees; import edu.seminolestate.payable.*;
public class HourlyEmployee extends Employee implements Payable{ private double hoursWorked; private double payRate;
public HourlyEmployee(String newFName, String newLName, int newId, double newHours, double newRate) throws IllegalArgumentException{ super(newFName, newLName, newId); this.setFirstName(newFName); this.setLastName(newLName); this.setiD(newId); this.setHoursWorked(newHours); this.setPayRate(newRate); }
public String toString() { return super.toString() + getClass() + "HourlyEmployees [ First Name=" + getFirstName() + ", Last Name=" + getLastName() + ", ID=" + getiD() + ", Pay Rate=" + payRate + ", Hours Worked=" + hoursWorked + "]"; } public double getHoursWorked() { return hoursWorked; }
public void setHoursWorked(double newHoursWorked) { this.hoursWorked = newHoursWorked; }
public double getPayRate() { return payRate; }
public void setPayRate(double newPayRate) { if (newPayRate > 0) this.payRate = newPayRate; else throw new IllegalArgumentException("Pay Rate must be > 0"); }
@Override public double computeAmountToPay() { // TODO Auto-generated method stub return hoursWorked * payRate; }
}
***********************
package edu.seminolestate.employees; import edu.seminolestate.payable.*;
public class Employee implements Payable{ private String firstName; private String lastName; private int iD;
public Employee(String newFName, String newLName, int newId) {
this.setFirstName(newFName); this.setLastName(newLName); this.setiD(newId); }
public String getFirstName() { return firstName; }
public void setFirstName(String newFName) { if (newFName == null || newFName.length() < 1) throw new IllegalArgumentException("First name cannot be null or empty."); else this.firstName = newFName; } public String getLastName() { return lastName; } public void setLastName(String newLName) { if (newLName == null || newLName.length() < 1) throw new IllegalArgumentException("Last name cannot be null or empty."); else this.lastName = newLName; }
public int getiD() { return iD; }
public void setiD(int newId) { if (iD >= 0) this.iD = newId; else throw new IllegalArgumentException("Id must be >= 0."); }
public String toString() { return getClass() + " [First Name=" + firstName + ", Last Name=" + lastName + ", Id=" + iD; }
@Override public double computeAmountToPay() { // TODO Auto-generated method stub return 0; }
}
**********************
package edu.seminolestate.payments;
import java.util.List; import java.io.File; import java.io.FileNotFoundException; import java.io.PrintWriter; import java.time.LocalDate; import java.time.format.DateTimeFormatter; import java.time.format.DateTimeParseException; import java.util.ArrayList; import java.util.Scanner;
import edu.seminolestate.bill.Bill; import edu.seminolestate.employees.HourlyEmployee; import edu.seminolestate.employees.Manager; import edu.seminolestate.employees.SalesManager; import edu.seminolestate.exceptions.InvalidArgumentException;
import edu.seminolestate.payable.Payable;
public class ManagePayablesApplication { private static final String FILE_NAME = "purchases.txt"; private static ArrayList payables; private static Scanner keyboard = new Scanner(System.in); private static ArrayList bill; private static final int ADD_HOURLY_EMPLOYEE = 1; private static final int ADD_MANAGER = 2; private static final int ADD_SALES_MANAGER = 3; private static final int ADD_BILL= 4; private static final int LIST_ALL_PAYABLES = 5; private static final int EXIT = 6; public static void main(String[] args) { payables = new ArrayList(); File file = new File(FILE_NAME); if (file.exists()){ loadArrayListFromFile(); } int response = 0; do { response = menu(); switch (response){ case ADD_HOURLY_EMPLOYEE:{ String FName = getString("Enter the first name."); String LName = getString("Enter the last name."); int Id = getInt("Enter ID"); double hours = getDouble("Enter the hours."); double rate = getDouble("Enter the rate"); HourlyEmployee employee = new HourlyEmployee(FName,LName, Id, hours,rate); payables.add(employee); break; } case ADD_MANAGER:{ String FName = getString("Enter the first name."); String LName = getString("Enter the last name."); int Id = getInt("Enter ID"); double salary = getDouble("Enter manager's annual salary"); Manager manager = new Manager(FName, LName, Id, salary); payables.add(manager); break; } case ADD_SALES_MANAGER:{ String FName = getString("Enter the first name."); String LName = getString("Enter the last name."); int Id = getInt("Enter ID"); double salary = getDouble("Enter sale's manager annual salary"); double sales = getDouble("Enter sales amount"); double rate = getDouble("Enter commision rate"); SalesManager salesmanager = new SalesManager(FName, LName, Id, salary, sales, rate); payables.add(salesmanager); break; } case ADD_BILL: LocalDate dueDate = getDate(); String vendorName = getString("Enter vendor name"); double amountOwed = getDouble("Enter bill amount"); Bill bill = new Bill(vendorName, amountOwed, dueDate); payables.add(bill); break; case LIST_ALL_PAYABLES: if (payables.isEmpty()){ System.out.println("There are no purchases."); } else { for (Payable v : payables){ System.out.println(v); } } break; case EXIT: if (payables.size() > 0) writeFileFromArrayList(); System.out.println("Thanks for using the purchases manager."); } } while (response != EXIT); } public static void writeFileFromArrayList(){ DateTimeFormatter mdyFormatter = DateTimeFormatter.ofPattern("M/d/yyyy"); File file = new File(FILE_NAME); try (PrintWriter printWriter = new PrintWriter(file)) { for (Payable v : payables){ //printWriter.println(v.getDueDate().format(mdyFormatter)); }} catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public static void loadArrayListFromFile(){ DateTimeFormatter formatter = DateTimeFormatter.ofPattern("M/d/yyyy"); LocalDate date; try (Scanner scanner = new Scanner(new File(FILE_NAME))) { while (scanner.hasNextLine()) { String tempVName = scanner.nextLine(); double tempAO = Double.parseDouble(scanner.nextLine()); String tempDate = scanner.nextLine(); date = LocalDate.parse(tempDate, formatter); Bill billed= new Bill(tempVName, tempAO, date); bill.add(billed); } } catch (NumberFormatException e){ System.out.println("Invalid cost in file " + FILE_NAME); System.out.println("No existing data to load."); } catch (FileNotFoundException e){ System.out.println("Could not find file: " + FILE_NAME); System.out.println("No existing data to load."); } } public static int menu(){ int userResponse = 0; /* * Redisplay the menu if an invalid value (not 1 through 3) is * entered and continue the application. */ String userInput; do { System.out.println("Enter your choice: "); System.out.println(ADD_HOURLY_EMPLOYEE + ". Add hourly employee"); System.out.println(ADD_MANAGER + ". Add manager"); System.out.println(ADD_SALES_MANAGER + ". Add sales manager"); System.out.println(ADD_BILL + ". Add bill"); System.out.println(LIST_ALL_PAYABLES + ". List all payables"); System.out.println(EXIT + ". Exit"); userInput = keyboard.nextLine(); try{ userResponse = Integer.parseInt(userInput); } catch (NumberFormatException e) { System.out.println("Enter numeric values for menu choice"); userResponse = -1; } if (userResponse < ADD_HOURLY_EMPLOYEE || userResponse > EXIT){ System.out.println("Invalid value. Enter a value " + ADD_HOURLY_EMPLOYEE + " - " + EXIT); } } while (userResponse < ADD_HOURLY_EMPLOYEE || userResponse > EXIT); return userResponse; } public static LocalDate getDate(){ DateTimeFormatter formatter = DateTimeFormatter.ofPattern("M/d/yyyy"); LocalDate date = null; boolean isValidDate = false; System.out.println("Enter a date (like 6/23/2016)"); do { try { String userInput = keyboard.nextLine(); date = LocalDate.parse(userInput, formatter); isValidDate = true; } catch (DateTimeParseException e) { System.out.println("You enetered an invalid date."); System.out.println("Enter a date (like 6/23/2016)"); } } while (! isValidDate); return date; } public static String getString(String prompt){ String userValue; do { System.out.println(prompt); userValue = keyboard.nextLine(); if (userValue == null || userValue.length() < 1){ System.out.println("You must enter a value."); } } while (userValue == null || userValue.length() < 1); return userValue; } public static double getDouble(String prompt){ String userValue = null; double validDouble = 0; boolean isValidDouble = false; do { System.out.println(prompt); try{ userValue = keyboard.nextLine(); validDouble = Double.parseDouble(userValue); if (validDouble >= 0) { isValidDouble = true; } else { System.out.println("Cost must be >= 0."); } } catch (NumberFormatException e) { System.out.println("Enter numerical value only"); } } while (! isValidDouble); return validDouble; } public static int getInt(String prompt){ String userValue = null; int validInt = 0; boolean isValidInt = false; do { System.out.println(prompt); try{ userValue = keyboard.nextLine(); validInt = Integer.parseInt(userValue); if (validInt >= 0) { isValidInt = true; } else { System.out.println("Cost must be >= 0."); } } catch (NumberFormatException e) { System.out.println("Enter numerical value only"); } } while (! isValidInt); return validInt; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
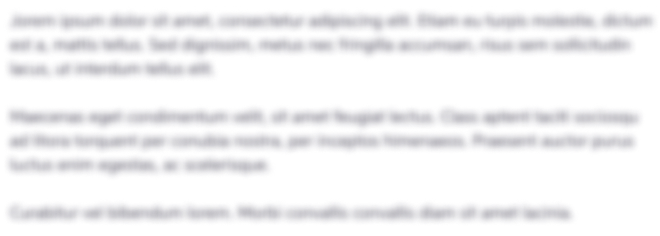
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started