Question
Hello! I need to implement sequence class using linked list in C++. This is the header file and class down here please help me.... FILE:
Hello! I need to implement sequence class using linked list in C++.
This is the header file and class down here please help me....
FILE: sequence.h
#ifndef SEQUENCE_H
#define SEQUENCE_H
#include
#include
using namespace std;
class Sequence
{
// The following declarations describe the functions that you must implement.
// You SHOULD NOT CHANGE any of the following PUBLIC declarations.
public:
typedef int value_type; // data type of sequence items
typedef unsigned int size_type; // data type of sequence counts and / or sizes
Sequence( size_type sz = 0 ); // creates a sequence indexed from 0 ... sz
Sequence( const Sequence& s ); // create a sequence from the existing sequence s
~Sequence(); // destroys the sequence
Sequence& operator=( const Sequence& s ); // assign sequence s to the sequence
value_type& operator[]( size_type p ); // return a reference to the item at index position p
value_type& at( size_type p ); // return a reference to the item at index position p
void push_back( const value_type& v ); // add v to the end of the sequence
void pop_back(); // remove item at the end of the sequence
void insert( size_type p, value_type v ); // insert the item v in the sequence at index position p
const value_type& front() const; // returns a reference to the first item in the sequence
const value_type& back() const; // returns a reference to the last item in the sequence
bool empty() const; // returns true if the sequence is empty
size_type size() const;
void clear(); // clears the sequence returning it to the empty state
void erase( size_type p, size_type n = 1 ); // deletes n number items starting a index position p
ostream& print( ostream& = cout ); // prints the items as a comma seperated sequence
// YOU CAN MODIFY the following private declarations as needed to implement your sequence class
private:
// If you choose to use a linked list, use the following
// private inner class for linked list nodes
// All data elements are public, since only class sequence can see SequenceNodes
class SequenceNode {
public:
SequenceNode *next;
SequenceNode *prev;
value_type elt;
SequenceNode() {
next = nullptr;
prev = nullptr;
}
SequenceNode(value_type item) {
next = nullptr;
prev = nullptr;
elt = item;
}
~SequenceNode() {};
};
// MEMBER DATA. These are the data items that each sequence object will contain. For a
// doubly-linked list, each sequence will have a head and tail pointer, and numElts
SequenceNode *head;
SequenceNode *tail;
size_type numElts; // Number of elements in the sequence
}; // End of class Sequence
// You must also implement the << operator. Do not change this declaration:
ostream& operator<<( ostream&, Sequence& );
#endif
FILE: Sequence.cpp
#include "Sequence.h"
using namespace std;
Sequence::Sequence( size_type sz ){}
Sequence::Sequence( const Sequence& s ){}
Sequence::~Sequence(){}
Sequence& Sequence::operator=( const Sequence& s ){}
Sequence::value_type& Sequence::operator[]( size_type position ){}
Sequence::value_type& Sequence::at( size_type position ){}
void Sequence::push_back( const value_type& value ){}
void Sequence::pop_back(){}
void Sequence::insert( size_type position, value_type value ){}
const Sequence::value_type& Sequence::front() const{}
const Sequence::value_type& Sequence::back() const{}
bool Sequence::empty() const{}
Sequence::size_type Sequence::size() const{}
void Sequence::clear(){}
void Sequence::erase( size_type position, size_type count ){}
ostream& Sequence::print( ostream& os ){}
ostream& operator<<( ostream& os, Sequence& s )
{}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
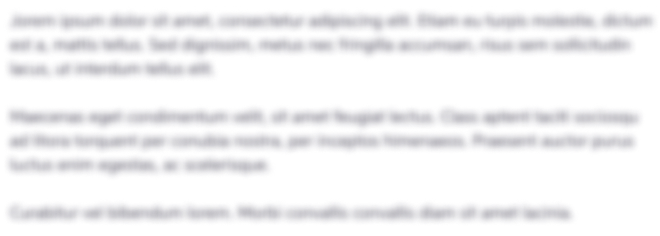
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started