Question
Hello, I seriously want to submit it ASAP, but in the matrix server for submitting I got an error message in the error.txt! How can
Hello, I seriously want to submit it ASAP, but in the matrix server for submitting I got an error message in the error.txt!
How can I resolve it??? Can you help me with an understandable explanation & correct code? Thank you in advance^-^
In Matrix server error.txt
In file included from Store.h:21:0, from Store.cpp:13: Toys.h:18:20: fatal error: String.h: No such file or directory #include "String.h" ^ compilation terminated. In file included from Toys.cpp:13:0: Toys.h:18:20: fatal error: String.h: No such file or directory #include "String.h" ^ compilation terminated. In file included from Store.h:21:0, from main_prof.cpp:18: Toys.h:18:20: fatal error: String.h: No such file or directory #include "String.h" ^ compilation terminated.
main.cpp
/*********************************************************************** // OOP244 Workshop 3 Part2: Member Functions and Privacy // File main.cpp // Version 1.0 // Date 2022/10/28 // Author Nargis // Description // Tests Store module // // Revision History // ----------------------------------------------------------- // Name Date Reason // ///////////////////////////////////////////////////////////////// ***********************************************************************/ #include
int main() {
Store s; cout << "Printing invalid list of Toys" << endl; s.setStore("****Children`s Dream store****", 2); s.setToys(nullptr, 20304576, 12.50, 2); //SKU should be 8 digit, price and age s.setToys("Car", 203045, 11.50, 12); s.display();
cout << endl << "Printing valid list of Toys" << endl; s.setStore("****Children`s Dream store****", 6); s.setToys("Racing Car", 11223344, 40.99, 8); s.setToys("Teddy Bear", 33772346, 25.99, 6); //onsale s.setToys("Airplane", 44453466, 60.99, 16); s.setToys("Doll", 55887896, 45.99, 5); s.setToys("Drone", 99221388, 90.99, 18);//onsale s.setToys("Lego", 88224596, 65.99, 10);//onsale s.display();
cout << endl << "Searching for toys and printing the list with sale price" << endl; s.find(33772346);//Teddy Bear s.find(99221388);//drone s.find(88224596); //lego s.display();
}
Store.cpp
#include "Store.h"
Store::Store() {}
Store::~Store() {}
void Store::setStore(const char* sName, int no) {
if ((sName != NULL) && (no != NULL)) {
// this->m_sName = new char[strlen(sName) + 1];
strcpy(this->m_sName, sName);
this->m_noOfToys = no;
}
}
//This will set the store name and the number of toys that could be added to the store. For invalid value, it will set the values to an empty state.
void Store::setToys(const char* tname, int sku, double price, int age) {
// check isf sku is equal to 8
std::stringstream ss;
ss << sku;
std::string str = ss.str();
if (str.length() != 8)
return;
if (m_addToys == m_noOfToys)
return;
if (tname == NULL) return;
Toys m_toys;
m_toys.addToys(tname, sku, price, age);
m_addToys++;
// check if we exceed the maximum number of array
try {
m_toy[m_addToys] = m_toys;
} catch (std::runtime_error& e) {
std::cout << "Error: " << e.what() << std::endl; }
}
//This will add the toys one at a time to the m_toy array and will keep track of the number of toys added. Toys will be added until num of added toys is less than m_noOfToys
void Store::display() const {
// check if store is valid
if ((m_sName != NULL) && (m_noOfToys != NULL) && (m_addToys != NULL)) {
//printHeader(m_sName);
/**
std::vector
**/
}
}
void Store::find(int sku) {
for (int i = 0; i < sizeof(m_toy) / sizeof(m_toy[0]); ++i) { /* code */
Toys current_m_toy = m_toy[i];
// check if the current sku matches with the provides sku
if (current_m_toy.matchSku(sku)) {
current_m_toy.isSale(true);
current_m_toy.calSale();
break;
}
}
}
//This method will go through the arrays of toys. It will match the received SKU number with the stored toys' SKU number to find out if the toys are on sale or not. If the number matches then it will pass true to the appropriate Toys method. After that, it will call the calSale() of the toys class to know the sale value.
void Store::setEmpty() {
// this->m_sName = NULL; // this->m_noOfToys= NULL;//No of toys; // this->m_addToys= NULL;//no of toys added // delete[] this->m_toy;// statically array of toys with size MAX_NUM_TOYS
}
Store.h
#ifndef STORE_H #define STORE_H
#define MAX_NUM_TOYS 10 //define it to 10. The maximum number of toys that could be added. #define MAX_SNAME 31 // define it to 31. The maximum length of a store name
#include "Toys.h"
#include "Utils.h"
#include
#include
#include
#include
#include
#include
class Store { char m_sName[MAX_SNAME];// Store name, up to MAX_SNAME size int m_noOfToys = 0;//No of toys; int m_addToys = 0;//no of toys added Toys m_toy[MAX_NUM_TOYS];// statically array of toys with size MAX_NUM_TOYS public: Store(); ~Store();
void setStore(const char* sName, int no);
//This will set the store name and the number of toys that could be added to the store. For invalid value, it will set the values to an empty state.
void setToys(const char* tname, int sku, double price, int age);
//This will add the toys one at a time to the m_toy array and will keep track of the number of toys added. Toys will be added until num of added toys is less than m_noOfToys
void display()const;
void find(int sku);
//This method will go through the arrays of toys. It will match the received SKU number with the stored toys' SKU number to find out if the toys are on sale or not. If the number matches then it will pass true to the appropriate Toys method. After that, it will call the calSale() of the toys class to know the sale value.
void setEmpty();
};
#endif
Toys.cpp
#include "Toys.h"
//Toys::Toys(){}
//After checking the validity, this method will set the received values to the appropriate data members. Otherwise will be set to an empty state. void Toys::addToys(const char* tname, int sku, double price, int age) {
if ((tname != NULL) && (tname[0] == '\0') && (sku != NULL) && (price != NULL) && (age != NULL)) {
strcpy(this->m_tname, tname);
this->m_sku = sku;
this->m_price = price;
this->m_age = age;
}
}
bool Toys::matchSku(int sku) {
return this->m_sku == sku;
}
//It will assign the received value to the data member m_onSale. void Toys::isSale(bool sale) {
this->m_onSale = sale;
}
//It will decrease the toys price to 20% then the original price. void Toys::calSale() {
//sale value
// decrease the sale price by 20%
this->m_price *= 0.8;
}
void Toys::display() const {
}
Toys::Toys() {}
Toys::~Toys() {}
Toys.h
#ifndef TOYS_H #define TOYS_H
#define MAX_TNAME 20
#include "String.h"
//#include "Store.h"
class Toys {
char m_tname[MAX_TNAME];// toy name, up to MAX_TNAME size int m_sku; //SKU number, maximum 8 digits long double m_price;//toy price int m_age;//toy according to age group bool m_onSale;// keep track, if toys are on sale or not
public: Toys(); ~Toys();
void addToys(const char* tname, int sku, double price, int age);
//After checking the validity, this method will set the received values to the appropriate data members. Otherwise will be set to an empty state.
void isSale(bool sale);
//It will assign the received value to the data member m_onSale.
void calSale();
//It will decrease the toys price to 20% then the original price.
void display()const;
bool matchSku(int sku);
};
#endif
Utils.h
#include
void printHeader(std::string m_sName);
void printHeader();
Utils.cpp
#include "Utils.h"
#include
void printHeader(char m_cName) { std::string m_sName(1, m_cName); std::cout << std::string(60, '*') << std::endl; std::cout << m_sName << std::endl; std::cout << std::string(60, '*') << std::endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
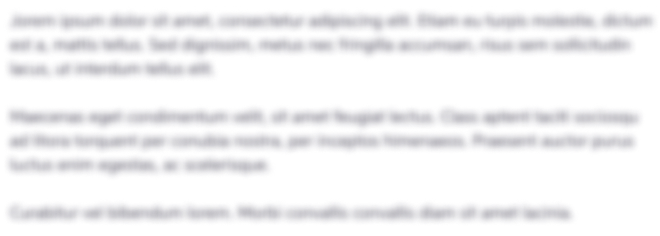
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started