Question
Hello! I was wondering if someone could please help me figure this out. Comments on the code would be much appreciated. I need the answer
Hello! I was wondering if someone could please help me figure this out. Comments on the code would be much appreciated. I need the answer as fast as possible please help!
Encryption is the act of changing information using an algorithm called a cipher that is unreadable. Encryption is commonly used in military communication (see Enigma machine) and to protect the confidentiality of electronic messages (the internet, e-commerce, Automatic Teller Machines, mobile phones, etc.).
Your goal is to create a program that can encrypt a text file as well as decrypt the text file using a given cipher. There are limitless ways to do this - you will be attempting two encryption programs, with the option for an advanced encryption project. You will be graded in two ways: your programs ability to encrypt accurately, and your programs ability to decrypt an already encrypted passage that will be provided to you.
CS Concepts implement in the project:
Modifying text using the String / Character / StringBuffer classes
Traversing a String using iteration
Reading and writing to a text file
Using a Scanner object to obtain user input
Using ASCII values to simplify Character modification
Part 1 - Secret Encryption
Secret encryption will analyze each character in a text file. It will find the most commonly occurring alphabetical character and use this as the conversion key.
In the encrypted output file, the first character printed will be the actual character that is responsible for the conversion key. The rest of the file will be the passage with each character shifted by the conversion key.
Steps for encrypting
1. Read the text file into a String (or StringBuffer) object
2. Traverse through the entire String. Find the most frequently occurring alphabetical character (advice: create a single method that takes a String as a parameter and returns a char)
3. Create a new String with the most common character appended to the front.
4. Shift each character in the String by incrementing by the ASCII value of the most common character (keep in range 32-126). Append each shifted character to the new String
* chars are primitive and can be treated as their int equivalent on an ASCII table
* visible chars have an ASCII range of 32-126. If you end up with a value of 127, it should instead be 32.
ex) Do not scorn pity that is the gift of a gentle heart, Eowyn!
most common char = t
ASCII value of t = 116
First few characters: D = 68; o = 111; = 32, n = 110, o = 111, t = 116
Do not becomes Y%5$%* check to see if you can do this by hand first!
Steps for decrypting
1. Strip the 1st char from the String. This is the most common char and has been unmodified
2. Shift each char by decrementing this ASCII value
* keep in range 32-126; if a char has an ASCII value of 31, it should instead be 126
3. Write this new String to a brand new text file
Part 2 - Top Secret Encryption
Top-Secret Encryption also analyzes each character in a text file, but it adds an additional level of encryption by utilizing a passcode. The passcode will then remap the alphabet onto a new cipher.
Steps for encrypting
1. Read the text file into a String (or StringBuffer)
2. Have the user enter a passcode
3. Form a cipher that has each alphabetical character occuring once. The first part of the cipher is composed of the unique letters of the passcode. The second part is the remainder of the alphabet in reverse order
For example:
* If the passcode is canons, then the cipherArray should be:
c a n o s z y x w v u t r q p m l k j i h g f e d b
*notice that n is repeated in the passcode, but each letter may only be used once in the cipherArray
*notice after the passcode, the unused alphabetical characters are placed in reverse order
4. Create a 2nd cipher for capital letters (hint - you dont need to repeat steps 2 & 3; consider using existing String method)
5. Go through each alphabetical character in the original text String; replace each letter with its cipherArray
For example:
Alphabet: A b c d e f g h i j k l m n o p q r s t u v w x y z
cipherArray: c a n o s z y x w v u t r q p m l k j i h g f e d b
Do not scorn becomes Op qp jnpkq
6. To disguise spaces, find the first non-occuring alphabetical character in the encrypted String. Replace all spaces with this character (advice: create a method that takes a String as a parameter and returns a char). Append this character to the front of the encrypted String
7. To disguise periods, find the second non-occuring (or 1st non-occuring AFTER replacing spaces) in the encrypted String. Replace all periods with this character. Append this character to index 1 (2nd position) of the encrypted String
Steps for decrypting
1. Read the encrypted text into a String
2. Strip the 1st 2 characters; the 1st is the encrypted space, the 2nd is the encrypted period
3. Replace all instances of both characters with spaces and periods. You must do this before step 5 to avoid issues.
4. Have the user type in the passcode; form the cipher as you did earlier
5. Replace all ciphered characters with their alphabetical equivalent
6. Write the final result to a new text file
Part 3 - explaining your code
Having good comments is an important part of communicating the purpose of your code to others. Sometimes it isnt enough; for this you will create a Flipgrid where you go through your own code and explain what each part does. Not only will this help demonstrate that you wrote and understand your own code, but it will help communicate to your teacher what each part of your code does.
Rubric
Comments
___/2 Uses /**JavaDoc*/ comments prior to each methods and constructor
___/2 uses //comments for complex code or unexplained variables
Programming Prose
___/5 code uses methods where appropriate; no excessive variables; professional prompts used
Secret
___/3 finds the correct most frequently occuring alphabetical character
___/3 shifts each character appropriately
___/1 checks and removes first encrypted character
___/3 characters are shifted back to their original state (including new-lines)
TopSecret
___/1 password is obtained through user input
___/2 cipherArray is properly formed (create a method that prints it to screen)
___/2 alphabetical letters appropriately replaced with cipher equivalent
___/2 spaces and periods appropriately replaced with non-occurring letters
___/3 characters are shifted back to their original state (including capitalization)
File Read/Write
___/2 reads text file and converts text to a String or StringBuffer
___/3 writes both encrypted and decrypted passages to new text files
Evaluation Check
Secret
___/2 program reads text file and displays decrypted version of String to console with no errors
___/3 encrypted String is appropriately decrypted
Top Secret
___/1 program prompts user for passcode
___/1program reads text file and displays decrypted version of String to console with no errors
___/3 encrypted String is appropriately decrypted
Video Reflection
___/1 Video is of appropriate length (3-5 minutes)
___/2 Video details all important segments of complex code
___/3 Video clearly demonstrates your understanding of your own code and explains it well
Total___ / 50
Step by Step Solution
There are 3 Steps involved in it
Step: 1
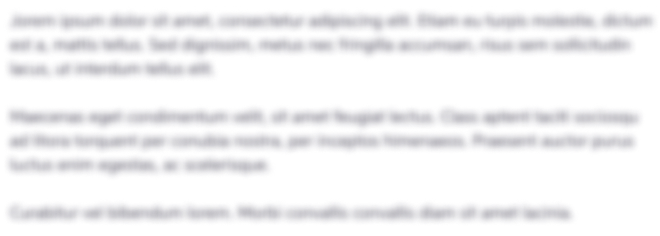
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started