Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Hello, I would like to get help in making a rectangle that can move within a background rectangle. It doesn't matter the directions of which
Hello, I would like to get help in making a rectangle that can move within a background rectangle. It doesn't matter the directions of which the rectangle will move, as long as it can move by itself without user interact to it. The speed can be gradually similar across the background rectangle.
Here is the code I have up to this point. Thank you for your help.
package com.example.pongs21; import java.util.Vector; import android.content.Context; import android.graphics.Canvas; import android.graphics.Paint; import android.graphics.Paint.FontMetrics; import android.graphics.Paint.Style; import android.graphics.Rect; import android.util.Log; import android.view.KeyEvent; import android.view.MotionEvent; import android.view.SurfaceHolder; import android.view.SurfaceView; import android.view.animation.AnimationUtils; public class PongView extends SurfaceView implements SurfaceHolder.Callback, Runnable { private final GameActivity gameActivity; private Thread _thread; private int screenWidth; // screen width private int screenHeight; // screen height Context _context; private SurfaceHolder _surfaceHolder; private boolean _run = false; // All pong variables go in here private float rPaddle; // example variable set by touch event private final static int MAX_FPS = 30; //desired fps private final static int FRAME_PERIOD = 1000 / MAX_FPS; // the frame period private Paint background = new Paint(); private Paint dark = new Paint(); //added // private Paint colorOfBall = new Paint(); // private Paint colorInsideBall = new Paint(); private float ballX = 10.0f; // ball location private float ballY = 10.0f; // private float ballXloc = 0.0f; //ball X direction move per update // private float ballYloc = 0.0f; // private float ballXsize = 30.0f; // private float ballYsize = 30.0f; // private float insideBallEdge = 3.0f; public PongView(Context context) { super(context); _surfaceHolder = getHolder(); getHolder().addCallback(this); this.gameActivity = (GameActivity) context; _context = context; setFocusable(true); setFocusableInTouchMode(true); } public void setRPaddle(float rp) { synchronized (_surfaceHolder) { rPaddle = rp; } } @Override public void run() { float avg_sleep = 0.0f; float fcount = 0.0f; long fps = System.currentTimeMillis(); Canvas c; while (_run) { c = null; long started = System.currentTimeMillis(); try { c = _surfaceHolder.lockCanvas(null); synchronized (_surfaceHolder) { // Update game state update(); } // draw image drawImage(c); } finally { // do this in a finally so that if an exception is thrown // during the above, we don't leave the Surface in an // inconsistent state if (c != null) { _surfaceHolder.unlockCanvasAndPost(c); } } float deltaTime = (System.currentTimeMillis() - started); int sleepTime = (int) (FRAME_PERIOD - deltaTime); if (sleepTime > 0) { try { _thread.sleep(sleepTime); } catch (InterruptedException e) { } } } } public void pause() { _run = false; boolean retry = true; while (retry) { try { _thread.join(); retry = false; } catch (InterruptedException e) { // try again shutting down the thread } } } public void initialize(int w, int h) { screenWidth = w; screenHeight = h; // create paints, rectangles, init time, etc background.setColor(0xff200040); // should really get this from resource file dark.setColor(0xffdddddd); } protected void update() { // game update goes here if (ballY > 10.0f) { ballX = 10.0f; ballY += 1.0f; } ballX++; invalidate(); } protected void drawImage(Canvas canvas) { //if (canvas == null) return; int width = getWidth(); int height = getHeight(); // Draw commands go here canvas.drawRect(ballX, ballY, ballX+1000, ballY+1000, background); canvas.drawRect(ballX, ballY, ballX+10, ballY+10, dark); } @Override protected void onSizeChanged(int w, int h, int oldw, int oldh) { screenWidth = w; screenHeight = h; super.onSizeChanged(w, h, oldw, oldh); initialize(w, h); } @Override public void surfaceChanged(SurfaceHolder holder, int format, int width, int height) { // TODO Auto-generated method stub } @Override public void surfaceCreated(SurfaceHolder holder) { _run = true; _thread = new Thread(this); _thread.start(); } @Override public void surfaceDestroyed(SurfaceHolder holder) { // simply copied from sample application LunarLander: // we have to tell thread to shut down & wait for it to finish, or else // it might touch the Surface after we return and explode boolean retry = true; _run = false; while (retry) { try { _thread.join(); retry = false; } catch (InterruptedException e) { // we will try it again and again... } } } @Override public boolean onTouchEvent(MotionEvent event) { setRPaddle(event.getY() / screenHeight); return true; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
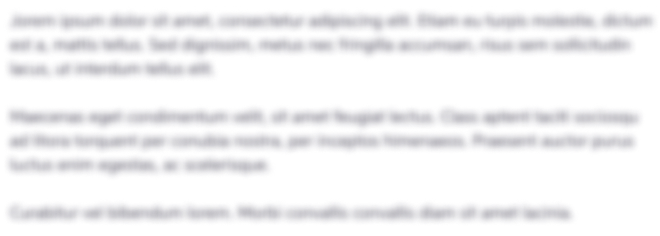
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started