Question
Hello. I would like to solve task 1,2, 3 today. Please, with details using java Task1: Write the implementation of the global (not member) method
Hello. I would like to solve task 1,2, 3 today. Please, with details using java
Task1: Write the implementation of the global (not member) method removeElements(StackList S1, int x) that takes a stack and integer value x, and then removes elements from the stack until finding the value x or the stack becomes empty. The method should return the stack after modification.
Example: S1 =
98 |
11 |
76 |
23 |
12 |
After executing the method removeElements (S1, 23 )
S1:
23 |
12 |
Task 2: Write the implementation of the global (not member) method WrapQueue(QueueList Q1, int x). The method takes a Queue and integer value X, and then wraps the queue according to the given value.
Example: Q1 =
32 | 12 | 5 | 6 | 7 |
After executing the method WrapQueue(Q1, 3 )
6 | 7 | 32 | 12 | 5 |
Note: Use the methods defined in the Queue ADT, (enQueue(int val), deQueue(),first()).
Task3: Write the implementation of the global (not member) method RevQue(QueueList Q1, int x). The method takes a Queue and integer value X, and reverses the order of the first X elements in the queue. The method should use the Stack structure to reverse the elements order.
Example: Q1 =
50 | 44 | 11 | 22 | 33 |
After executing the method RevQue(Q1, 3 )
11 | 44 | 50 | 22 | 33 |
Task4: Use Big-O notation to analyze the efficiency of the methods that you implemented in tasks 1, 2, and 3. Write the time complexity (in terms of Big-O) for each method as a comment in the source code file.
Task 5: Using the data structures you have learned in this course, develop a management system for students' records in the college. The system should enable users to efficiently store, process/update, and track students records. In order to achieve this, design and implement the appropriate structures/Classes/Interfaces/methods with space/time efficiency in mind
The main functionalities of the system:
- Insert: inserts a new student record in the system
- Retrieve: searches for a student record using a key value/attribute.
- Process: performs processing tasks. The major processing tasks are: assigning a new GPA, modifying Mobile No, change Speciality.
- Print: Prints students' records
- Delete: deletes a student record in the system
- Track: Allows to back track the latest updated/processed students' records
For each student record, you need to include the following attributes (you can add more attributes as needed):
(Studentid, Name, Birth Date, Mobile, Address, Specialty, GPA)
- Draw the class diagrams for the system.
- Implement and test the system. Use a driven menu to display the above requirements properly.
- Design and Implement each of the following data structures along with their essential operations:
- Implement a Singly Linked List to store all students' records.
- Implement a Stack for tracking the updated/processed records.
- Implement a Queue for handling the processing tasks of students records.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
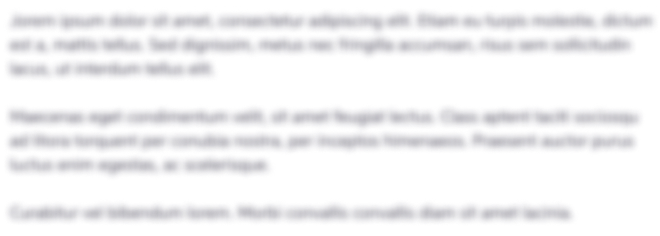
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started