Hello, I'm having some trouble with this Java project. I cannot figure out why the text files member.txt and register.txt are not being updated and
Hello,
I'm having some trouble with this Java project. I cannot figure out why the text files member.txt and register.txt are not being updated and saved when i run the program. Methods "modify_member" and "modify_registration" should be updating the files... I am also not sure how to write the code for step 5. Any guidance in the right direction is very much appreciated. Thank you!
INSTRUCTIONS:
1. Add two new sets of parallel arrays to your program, these arrays will store the information that will be read in from the register.txt file. That file contains the member's code and the name of the class they will be registered for. The two parallel arrays will be names of your choice, but each will be defined as being 100 big.
- Again, you will need to define:
- Register Member code to be an Integer array defined as 100 big
- Register Class name to be a String array defined as 100 big
- You will also need to define a new variable that will keep track of how many registration items that have been read in from the data file.This new variable will work with the registration file the same way the variable mcount works with the member file.
2. Add code to the read_registration method. In this method, you will read in all data from the register.txt file into the new parallel arrays defined in #1 above. This code is just like the code in the method read_member where you are reading in the member information.
- You will have to change the call to the read_register statement passing the arrays and the count you defined in step #1 above.
- Drag the additional data file register.txt to the same folder where the file member.txt is. Remember that the location of the data files is the main folder of your java project (just under the eclipse workspace folder) (contents of the register.txt file can be viewed at the end of this assignment)
- Add code to the Modify member method. In this method, you will increment the member count (mcount) and prompt the user for new member data to be read into the member parallel arrays. You will have to change the call to the modify_member method, passing the arrays and the member count
3. Add code to the Modify Registration method In this method, you will increment the registration count (defined in step #1 above) and prompt the user for new registration data to be read into the registration parallel arrays. You will have to change the call to the modify_registration method, passing the arrays ant the registration count
- The possible class names are:
- Yoga
- Karate
- AerobicsI
- AerobicsII
- SwimmingI
- SwimmingII
4. Add code to the exit_program method. In this method you will:
- Add the code to write the member data back to the data file member.txt.
- Add the code to write the registration data back to the data file register.txt.
- You will have to change the call to the exit_program method, passing the arrays and counts
5. Add code to the Report Method
- You will have to change the call to the report method, passing the arrays and the counts
- Change the menu text to the wording below
- All Member Information
- All Registration Information
- All Members of a Specific type
- All Members between a specific age range
- All Members of a specific gender
- Specific Member's Registration
- Report 7
- Report 8
- Report 9
- Report 10
- Exit Report Menu
- Each report is defined as follows:
- All Member Information - Displays all information on all members
- All Registration information -Display all information for all registrations
- All Members of a Specific type- Prompt for the type and then display all information for members of that type
- All Members between a specific age range - Prompt for the beginning and ending age, then display all member information for the members within the date range
- All Members of a specific gender - Prompt for the gender and then display all members of that gender.
- Specific Member's Registration - List of all classes a specific member is signed up for (prompt for the member code) You must display all of the member's information and then search the registration arrays and when there is a match on the member's code, print the list of all classes he is registered for
- Report 7 print message "Report 7 to be defined later"
- Report 8 print message "Report 8 to be defined later"
- Report 9 print message "Report 9 to be defined later"
- Report 10 print message "Report 10 to be defined later"
MY CODE:
package ymca; import javax.swing.JOptionPane; import java.io.*; import java.util.*; import java.text.*; public class ymca { public static void main(String[] args) { //***************************************************** int mcount=-1,i; int rcount=-1,r; int [] code = new int[100]; int [] age = new int [100]; int [] membercode = new int [100]; String [] type = new String [100]; String [] name = new String [100]; String [] gender = new String[100]; String [] classname = new String [100]; double [] payment = new double [100]; //*****************************************************
//Main Method********************************************** int selection; String snumber; mcount=read_member(mcount,code,name,type,age,gender,payment); rcount=read_registration(rcount,code,classname); selection = menu(); while(selection !=4) { if(selection==1) Modify_member(mcount,code,name,type,age,gender,payment); else if(selection==2) Modify_registration(rcount,code,classname); else if(selection==3) Report(mcount,code,name,type,age,gender,payment); selection = menu(); }//while loop exit_program(mcount,code,name,type,age,gender,payment,rcount,classname); exit_program2(mcount,code,name,type,age,gender,payment,rcount,classname); System.exit(0); } //Main Method END***************************************
//read_member Method*********************************** public static int read_member(int mcount,int[]code,String[]name,String[]type,int[]age,String[]gender,double[]payment) { int i; String newLine; try { //define a file variable for Buffered read BufferedReader member_file = new BufferedReader(new FileReader("member.txt")); //read lines in file until there are no more lines in the file to read while ((newLine = member_file.readLine()) != null) { //there is a "#" between each data item in each line StringTokenizer delimiter = new StringTokenizer(newLine,"#"); mcount=mcount+1; code[mcount] = Integer.parseInt(delimiter.nextToken()); name[mcount] =delimiter.nextToken(); type[mcount] =delimiter.nextToken(); age[mcount] = Integer.parseInt(delimiter.nextToken()); gender[mcount] =delimiter.nextToken(); payment[mcount] = Double.parseDouble(delimiter.nextToken()); }//while loop member_file.close(); }//end try catch (IOException error) { //there was an error on the file writing System.out.println("Error on file read " + error); }//error on read return mcount; } //read_member Method END**************************************** //read_registration Method****************************** public static int read_registration(int rcount,int[]code,String[]classname) { int r; String newLine; try { BufferedReader register_file = new BufferedReader(new FileReader("register.txt")); while ((newLine = register_file.readLine()) != null) { StringTokenizer delimiter = new StringTokenizer(newLine,"#"); rcount=rcount+1; code[rcount] = Integer.parseInt(delimiter.nextToken()); classname[rcount] =delimiter.nextToken(); } register_file.close(); } catch (IOException error) { System.out.println("Error on file read " + error); } return rcount; } //read_registration Method END************************************** //Menu Method*********************************************** public static int menu() { String snum; int selection; String Output = "Pittsburgh Area YMCA" + " " + "1. Add/Modify Member Information" + " " + "2. Add/Modify Class Registrations " + " " + "3. Report Section" + " " + "4. Exit the System"+ " " +" " + "Please make your seleciton"; snum = JOptionPane.showInputDialog(null, Output, "",JOptionPane.QUESTION_MESSAGE); selection = Integer.parseInt(snum); return selection; } //Menu Method END*********************************************
//Modify_member Method******************************************* public static int Modify_member(int mcount,int[]code,String[]name,String[]type,int[]age,String[]gender,double[]payment) { String value; int select; int delvalue,position=0,i; int modvalue,changeit; String output="Modify Member Information"+" "+ "1. Add member"+" "+ "2. Modify member"+" "+ "3. Delete member"+" "+ "4. Exit Modify"+" "+ "please make your selection "; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); select =Integer.parseInt(value); while(select!=4) { if(select ==1) { mcount=mcount+1; output= "Enter the Code"; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); code[mcount]=Integer.parseInt(value); output= "Enter the Name"; name[mcount] =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); output= "Enter the type (Single, Couple, or Family)"; type[mcount] =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); output="Enter the age"; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); age[mcount]=Integer.parseInt(value); output= "Enter the Gender (Male or Female)"; gender[mcount] =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); output="Enter the Payment Amount"; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); payment[mcount]=Double.parseDouble(value); }//end of select =1 else if (select==2) { output= " Enter the Code to modify "; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); modvalue=Integer.parseInt(value); //find the position of the number to modify for (i=0; i<=mcount; ++i) { if(code[i]==modvalue) position = i; } //modify the proper data output="1. Change the name "+" "+ "2. Change the Type "+" "+ "3. Change the age "+" "+ "4. Change the Gender "+" "+ "5. Change the Payment "+" "+ "Enter Your selection "; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); changeit=Integer.parseInt(value); if(changeit ==1) { //read new name output= "Enter the NEW Name"; name[position] =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); } else if(changeit ==2) { //read new type output= "Enter the NEW Type (Single, Couple, or Family)"; type[position] =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); }
else if(changeit ==3) { //read new age output="Enter the NEW Age"; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); age[position]=Integer.parseInt(value); } else if(changeit ==4) { //read new gender output= "Enter the NEW Gender (Male or Female)"; gender[position] =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); } else if(changeit ==3) { //read new Payment output="Enter the NEW Payment"; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); payment[position]=Double.parseDouble(value); } }//end of select=2 if(select==3) { output= " Enter the Code to delete "; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); delvalue=Integer.parseInt(value); //find the position of the number to delete for (i=0; i<=mcount; ++i) { if(code[i]==delvalue) position = i; } //delete from the arrays for(i=position;i<=mcount-1;++i) { code[i]=code[i+1]; name[i]=name[i+1]; type[i]=type[i+1]; age[i]=age[i+1]; gender[i]=gender[i+1]; payment[i]=payment[i+1]; } mcount=mcount-1; }//end of select=3 output="Modify Member Information"+" "+ "1. Add member"+" "+ "2. Modify member"+" "+ "3. Delete member"+" "+ "4. Exit Modify"+" "+ "please make your selection "; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); select =Integer.parseInt(value); }//end of while loop return mcount; }
//Modify_member Method END***********************************************
//Modify_registration Method******************************************** public static int Modify_registration(int rcount,int[]code,String[]classname) { String value; int select; int delvalue,position=0,i; int modvalue,changeit; String output="Modify Member Information"+" "+ "1. Add Member"+" "+ "2. Modify Member"+" "+ "3. Delete Member"+" "+ "4. Exit Modify"+" "+ "please make your selection "; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); select =Integer.parseInt(value); while(select!=4) { if(select ==1) { rcount=rcount+1; output= "Enter the Code"; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); code[rcount]=Integer.parseInt(value); output= "Enter the Class Name (Yoga, Karate, AerobicsI, AerobicsII, SwimmingI, or SwimmingII"; classname[rcount] =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); }//end of select =1 else if (select==2) { output= " Enter the Code to modify "; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); modvalue=Integer.parseInt(value); //find the position of the number to modify for (i=0; i<=rcount; ++i) { if(code[i]==modvalue) position = i; } //modify the proper data output="1. Change the Code "+" "+ "2. Change the Class Name "+" "+ "Enter Your selection "; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); changeit=Integer.parseInt(value); if(changeit ==1) { //read new Code output="Enter the NEW Code"; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); code[position]=Integer.parseInt(value); } else if(changeit ==2) { //read new class name output= "Enter the NEW Class name"; classname[position] =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); } }//end of select=2 else if(select==3) { output= " Enter the Code to delete "; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); delvalue=Integer.parseInt(value); //find the position of the number to delete for (i=0; i<=rcount; ++i) { if(code[i]==delvalue) position = i; } //delete from the arrays for(i=position;i<=rcount-1;++i) { code[i]=code[i+1]; classname[i]=classname[i+1]; } rcount=rcount-1; }//end of select=3 output="Modify Member Information"+" "+ "1. Add member"+" "+ "2. Modify member"+" "+ "3. Delete member"+" "+ "4. Exit Modify"+" "+ "please make your selection "; value =JOptionPane.showInputDialog(null, output," ",JOptionPane.QUESTION_MESSAGE); select =Integer.parseInt(value); }//end of while loop return rcount; } //Modify_registration Method END*************************************************
//Report Method********************************************* public static void Report(int mcount,int[]code,String[]name,String[]type,int[]age,String[]gender,double[]payment) { int selection,i; String value; String words= "Pittsburgh Area YMCA"+" " + "1. All Member Information"+" " + "2. All Registration Information"+" " + "3. All Members of a Specific type"+" " + "4. All Members between a specific age range"+" " + "5. All Members of a specific gender"+" " + "6. Specific Member's Registration"+" " + "7. Report 7"+" " + "8. Report 8"+" " + "9. Report 9"+" " + "10. Report 10"+" " + "11. Exit Report Menu"+" " + " Please make your selection "; value=JOptionPane.showInputDialog(null, words, "Input Data", JOptionPane.QUESTION_MESSAGE); selection=Integer.parseInt(value); while (selection != 11) { if (selection == 1) { System.out.println("All Member Information"); for(i=0;i<=mcount;++i) { System.out.println(code[i]+" "+name[i]+" "+type[i]+" "+age[i]+" "+gender[i]+" "+payment[i]); } }//end of report 1 else if (selection == 2) { System.out.println("Add Code here for Report #2"); }//end of report 2 else if (selection == 3) { System.out.println("Add Code here for Report #3"); }//end of report 3 else if (selection == 4) { System.out.println("Add Code here for Report #4"); }//end of report 4 else if (selection == 5) { System.out.println("Add Code here for Report #5"); }//end of report 5 else if (selection == 6) { System.out.println("Add Code here for Report #6"); }//end of report 6 else if (selection == 7) { System.out.println("Add Code here for Report #7"); }//end of report 7 else if (selection == 8) { System.out.println("Add Code here for Report #8"); }//end of report 8 else if (selection == 9) { System.out.println("Add Code here for Report #9"); }//end of report 9 else if (selection == 10) { System.out.println("Add Code here for Report #10"); }//end of report 10 value=JOptionPane.showInputDialog(null, words, "Input Data", JOptionPane.QUESTION_MESSAGE); selection=Integer.parseInt(value); }//end of while loop } //Report Method END******************************************************
//exit_program1 Method****************************************** public static int exit_program(int mcount,int[]code,String[]name,String[]type,int[]age,String[]gender,double[]payment,int rcount,String[]classname) { System.out.println("executing exit_system"); int r; try {
BufferedWriter register_file = new BufferedWriter(new FileWriter("register.txt")); for (r=0; r<=rcount; ++r) { register_file.write(code[r]+"#"+classname[r] + "#"); register_file.newLine(); } register_file.close(); } catch (IOException error) { System.out.println("Error on file write " + error); } return rcount; } //exit_program Method END************************************************
//exit_program2 Method****************************************** public static int exit_program2(int mcount,int[]code,String[]name,String[]type,int[]age,String[]gender,double[]payment,int rcount,String[]classname) { System.out.println("executing exit_system2"); int i; try { BufferedWriter member_file = new BufferedWriter(new FileWriter("member.txt")); for (i=0; i<=mcount; ++i) { member_file.write(code[i]+"#"+name[i] + "#" + type[i] + "#" +age[i]+ "#"+gender[i]+ "#" +payment[i]+ "#"); member_file.newLine(); } member_file.close();
} catch (IOException error) { System.out.println("Error on file write " + error); } return mcount; } //exit_program2 Method END************************************************ }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
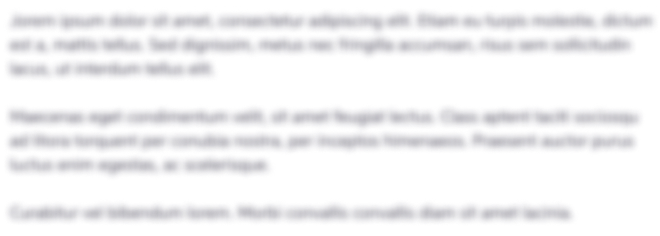
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started