Question
Hello, I'm in need of some help. I have a program almost done and I could use some help to finish part of the requirement.
Hello, I'm in need of some help. I have a program almost done and I could use some help to finish part of the requirement. I already have the first one done, its the second one I don't have done. Pretty much the program takes in numbers from the users, allows us to save the list made, and able to load the list back up. But it is also supposed to remove odd numbers, and right now it doesn't remove the odd numbers. Help please! Thank you!
"1. complete the NumberList class: fix the removeOdd() method so that it removes all odd numbers from the list.
2. complete the NumberListTest class: write a good unit test for the removeOdd() method so that we can make sure it works as it is supposed to."
//NumberList.java
package inclass;
import java.util.ArrayList; import java.util.Iterator; import java.util.List;
public class NumberList { private List
public NumberList() { numbers = new ArrayList<>(); }
public int add(int a, int b) { return a + b; }
public void add(int i) { numbers.add(i); }
/* * The remove(int) method will remove the specified value from the numbers * list and return true - if an item was removed false - if nothing was * removed. */ public boolean remove(int i) { Integer nr = i; if (numbers.contains(nr)) { return numbers.remove(nr); } else return false; }
/* * The getNumbers method will return a copy of the numbers list */ public List
/* * The isEqual(List
/* * The removeOdd method should remove all odd numbers from the numbers list * and return true - if one or more items were removed false - if nothing * was removed. */ public boolean removeOdd() { boolean result = false; Iterator
while (itera.hasNext()) {
Integer in = itera.next();
if (in.intValue() % 2 == 1) { itera.remove(); result = true; } } return result; }
}
---------------------
//MyNumber.java
package serial;
import java.io.Serializable;
public class MyNumber implements Serializable {
private static final long serialVersionUID = 2L;
private int number;
public MyNumber(int number) {
super();
this.number = number;
}
public int getNumber() {
return number;
}
public void setNumber(int number) {
this.number = number;
}
@Override
public String toString() {
return "" + number;
} }
--------------------------------
//SerializationExample.java
package serial;
import java.awt.Color; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream;
import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JTextField;
public class SerializationExample extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L; private JButton clearbutton; private JTextField ytextfield; private JButton savebutton; private JButton loadbutton; private MyNumber mynumber;
private void initializeWindow(String title) { setTitle(title); setBounds(100, 100, 400, 300); setLayout(null); getContentPane().setBackground(Color.YELLOW);
ytextfield = new JTextField(); ytextfield.setBounds(50, 20, 100, 25); add(ytextfield); ytextfield.setVisible(true); ytextfield.addActionListener(this);
clearbutton = new JButton("Clear"); clearbutton.setBounds(250, 20, 90, 25); add(clearbutton); clearbutton.addActionListener(this); clearbutton.setVisible(true);
savebutton = new JButton("Save to file"); savebutton.setBounds(30, 100, 150, 25); add(savebutton); savebutton.addActionListener(this); savebutton.setVisible(true);
loadbutton = new JButton("Load from file"); loadbutton.setBounds(200, 100, 150, 25); add(loadbutton); loadbutton.addActionListener(this); loadbutton.setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setVisible(true); }
public SerializationExample() { initializeWindow("Assignment #10: Integer Store"); }
public static void main(String[] args) { new SerializationExample(); }
@Override public void actionPerformed(ActionEvent e) { if (e.getSource() == ytextfield) {
} if (e.getSource() == clearbutton) { ytextfield.setText(""); } if (e.getSource() == savebutton) { int n = Integer.parseInt(ytextfield.getText()); mynumber = new MyNumber(n);
try { ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("CurrentValue.obj")); oos.writeObject(mynumber); oos.close(); } catch (FileNotFoundException e1) { e1.printStackTrace(); } catch (IOException e1) { e1.printStackTrace(); } } if (e.getSource() == loadbutton) { try { ObjectInputStream ois = new ObjectInputStream(new FileInputStream("CurrentValue.obj")); mynumber = (MyNumber) ois.readObject(); ytextfield.setText(mynumber.toString()); ois.close(); // May be needed on MacOS repaint(); } catch (FileNotFoundException e1) { e1.printStackTrace(); } catch (IOException e1) { e1.printStackTrace(); } catch (ClassNotFoundException e1) { e1.printStackTrace(); } }
} }
----------------------
//WindowSerialization.java
package serial;
import java.awt.Color; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.EOFException; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream;
import javax.swing.DefaultListModel; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JList; import javax.swing.JTextField;
public class WindowSerialization extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JLabel prompt;
private JButton clearbutton;
private JTextField ytextfield;
private DefaultListModel
private JList
private JButton savebutton;
private JButton loadbutton;
private void initializeWindow(String title) {
setTitle(title);
setBounds(100, 100, 400, 300);
setLayout(null);
getContentPane().setBackground(Color.YELLOW);
prompt = new JLabel("Enter a number and press Enter:");
prompt.setBounds(10, 20, 240, 25);
add(prompt);
prompt.setVisible(true);
ytextfield = new JTextField();
ytextfield.setBounds(250, 20, 100, 25);
add(ytextfield);
ytextfield.setVisible(true);
ytextfield.addActionListener(this);
listModel = new DefaultListModel
list = new JList
list.setBounds(30, 60, 250, 150);
list.setLayoutOrientation(JList.HORIZONTAL_WRAP);
add(list);
clearbutton = new JButton("Clear");
clearbutton.setBounds(300, 100, 90, 25);
add(clearbutton);
clearbutton.addActionListener(this);
clearbutton.setVisible(true);
savebutton = new JButton("Save to file");
savebutton.setBounds(30, 230, 150, 25);
add(savebutton);
savebutton.addActionListener(this);
savebutton.setVisible(true);
loadbutton = new JButton("Load from file");
loadbutton.setBounds(200, 230, 150, 25);
add(loadbutton);
loadbutton.addActionListener(this);
loadbutton.setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public WindowSerialization() {
initializeWindow("Assignment #10: Integer Store");
}
public static void main(String[] args) {
new WindowSerialization();
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == ytextfield) {
int n = Integer.parseInt(ytextfield.getText());
listModel.addElement(n);
}
if (e.getSource() == clearbutton) {
ytextfield.setText("");
listModel.clear();
}
if (e.getSource() == savebutton) {
try {
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("CurrentValue.obj"));
for (int i = 0; i < listModel.size(); i++)
{
MyNumber mynumber = new MyNumber(listModel.get(i));
oos.writeObject(mynumber);
}
oos.close();
} catch (FileNotFoundException e1) {
e1.printStackTrace();
} catch (IOException e1) {
e1.printStackTrace();
}
}
if (e.getSource() == loadbutton) {
try {
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("CurrentValue.obj"));
MyNumber mynumber = (MyNumber) ois.readObject();
listModel.clear();
while (mynumber != null)
{
listModel.addElement(mynumber.getNumber());
mynumber = (MyNumber) ois.readObject();
}
ytextfield.setText(mynumber.toString());
ois.close();
// May be needed on MacOS
repaint();
} catch (EOFException e2) {
// when EOF is reached
}
catch (FileNotFoundException e1) {
e1.printStackTrace();
} catch (IOException e1) {
e1.printStackTrace();
} catch (ClassNotFoundException e1) {
e1.printStackTrace();
}
}
}
}
----------------
(Following class is actually a Junit Test Case)
//NumberListTest.java
package inclass;
import static org.junit.Assert.fail;
import java.util.ArrayList; import java.util.List;
import org.junit.After; import org.junit.AfterClass; import org.junit.Assert; import org.junit.Before; import org.junit.BeforeClass; import org.junit.Test;
public class NumberListTest {
private NumberList add;
@BeforeClass public static void setUpBeforeClass() throws Exception { }
@AfterClass public static void tearDownAfterClass() throws Exception { }
@Before public void setUp() throws Exception { System.out.println("Set up"); add = new NumberList(); }
@After public void tearDown() throws Exception { }
@Test public void testAddTwoInts() { int t1 = 100; int t2 = 200; int expected = 300; int result = add.add(t1, t2); org.junit.Assert.assertEquals(expected, result); }
@Test public void testAddAnInt() { fail("Not Implemented"); }
@Test public void testGetNumbers() { List
add.add(1); add.add(2); add.add(3);
Assert.assertTrue(add.isEqual(numbers)); // fail("Not Implemented"); }
@Test public void testRemoveAnInt() { fail("Not Implemented"); }
@Test public void testIsEqual() { fail("Not Implemented"); }
@Test public void testRemoveOdd() { fail("Not Implemented"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
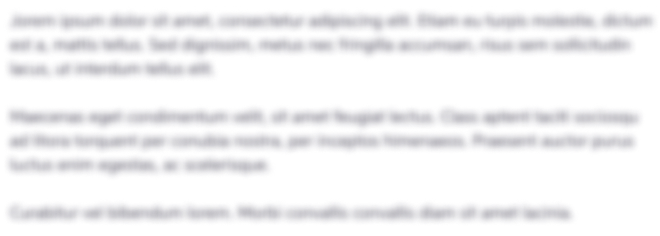
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started