Question
Hello, I'm trying to figure out a way to loop my program correctly, but I've run into a tree. What would be the correct code
Hello, I'm trying to figure out a way to loop my program correctly, but I've run into a tree. What would be the correct code in order to get similar output to the example?
Here's my code in Java:
import java.util.Scanner; import java.util.Random;
public class Main { public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); Random random = new Random(); System.out.println(); System.out.println(); System.out.println("You're a crewmember on a newly deserted spacecraft that has crashed on a hostile planet."); System.out.println("It's up to you to repair the spacecraft and leave the hostile planet, which houses gruesome aliens!"); System.out.println("Your spaceship is equipped with missiles, which you are to use effectively against the aliens."); System.out.println("But be careful! There is only a limited amount. Use it wisely to defeat the aliens and return home!"); int startingHealth = random.nextInt(11) + 15; int playerAmmo = 4; int maxAmmo = 4; int aliensStanding = random.nextInt(11) + 10; int maxHealth = 25; boolean playAgain = true;
while (playAgain) { boolean continuePlaying = true; while (continuePlaying) { while (aliensStanding > 0 && startingHealth > 0) { System.out.println(); System.out.println("Player Health: " + startingHealth); System.out.println("Remaining Ammo: " + playerAmmo); System.out.println("Alien foes: " + aliensStanding); System.out.println(); System.out.println("Choose your next move!"); System.out.println("1. Attack the enemy"); System.out.println("2. Retreat from battle"); System.out.println("3. Reload ammunition");
int userChoice = keyboard.nextInt();
if (userChoice == 1) { if (playerAmmo == 0) { System.out.println("You've run out of ammo!"); System.out.println("You attempted to escape, but unfortunately, you died."); startingHealth = 0; continuePlaying = false; } else { int aliensDestroyed = random.nextInt(5) + 1; int damageTaken = 0; if (random.nextDouble() < 0.5) { damageTaken = random.nextInt(6) + 1; System.out.println("You've engaged the enemy and destroyed " + aliensDestroyed + " aliens, but took " + damageTaken + " damage!"); aliensStanding -= aliensDestroyed; playerAmmo--; startingHealth -= damageTaken; if (startingHealth <= 0) { System.out.println("You've taken too much damage and died."); continuePlaying = false; } } else { System.out.println("You've engaged the enemy and destroyed " + aliensDestroyed + " aliens!"); aliensStanding -= aliensDestroyed; playerAmmo--; } if (aliensStanding <= 0) { System.out.println("Congratulations! You've defeated all the aliens!"); continuePlaying = false; } } } else if (userChoice == 2) { if (random.nextDouble() < 0.4) { if (startingHealth < maxHealth) { startingHealth += 5; if (startingHealth > maxHealth) { startingHealth = maxHealth; } System.out.println("You've successfully evaded! You've gained 5 health."); } else { startingHealth -= 5; System.out.println("You already have full health! Take 5 damage."); if (startingHealth <= 0) { System.out.println("You've taken too much damage and died."); continuePlaying = false; } } } else { int damageTaken = random.nextInt(10) + 1; startingHealth -= damageTaken; System.out.println("You've taken " + damageTaken + " damage."); if (startingHealth <= 0) { System.out.println("You've taken too much damage and died."); continuePlaying = false; } } } else if (userChoice == 3) { if (playerAmmo == maxAmmo) { startingHealth -= 5; System.out.println("You already have max ammo! Take 5 damage."); if (startingHealth <= 0) { System.out.println("You've taken too much damage and died."); continuePlaying = false; } } else { int reloadAmount = random.nextInt(2) + 1; if (playerAmmo + reloadAmount > maxAmmo) { reloadAmount = maxAmmo - playerAmmo; } if (random.nextDouble() < 0.4) { int damageTaken = random.nextInt(10) + 1; playerAmmo += reloadAmount; System.out.println("You've taken " + damageTaken + " damage while reloading!"); System.out.println("You've gained " + reloadAmount + " missiles!"); startingHealth -= damageTaken; if (startingHealth <= 0) { System.out.println("You've taken too much damage and died."); continuePlaying = false; } } else { playerAmmo += reloadAmount; System.out.println("You've successfully reloaded " + reloadAmount + " without taking damage!"); } } } else { System.out.println("Invalid choice! Try again."); continue; }
System.out.println("Do you want to play again? (y/n)"); String playAgainInput = keyboard.next(); if (playAgainInput.equals("n")) { playAgain = false; } else if (playAgainInput.equals("y")) { playAgain = true; } else { System.out.println("Invalid Input."); playAgain = false; }
if (playAgain) { startingHealth = random.nextInt(11) + 15; playerAmmo = 4; aliensStanding = random.nextInt(11) + 10; } else { System.out.println("Thanks for playing!"); break; }
} } } } }
Example Output:
Example Output 2 You are a crew member of a spacecraft that has crash-landed on a hostile planet. Your mission is to repair the spacecraft and escape before the planet's alien inhabitants find and attack you. Your spacecraft is equipped with missiles, but it has limited ammo. You must use your weapon wisely to defeat the aliens and avoid taking damage. Good luck! Player health: 25 Player ammo: 4 Aliens remaining: 25 Choose an action: 1. Attack 2. Evade 3. Reload 1 You attacked the aliens and defeated 5 of them, and avoided taking damage! Player health: 25 Player ammo: 3 Aliens remaining: 20 Choose an action: 1. Attack 2. Evade 3. Reload 1 You attacked the aliens and defeated 2 of them, but took 6 damage! Player health: 19 Player ammo: 2 Aliens remaining: 18 Choose an action: 1. Attack 2. Evade 3. Reload 2 The aliens hit you while you were evading and caused 4 damage! Player health: 15 Player ammo: 2 Aliens remaining: 18 Choose an action: 1. Attack 2. Evade 3. Reload 2 You successfully avoided the aliens and gained 5 health points! Player health: 20 Player ammo: 2 Aliens remaining: 18 Choose an action: 1. Attack 2. Evade 3. Reload 3 You reloaded 2 missile(s) into your magazine. Reloading operation complete! Player health: 20 Player ammo: 4 Aliens remaining: 18 Choose an action: 1. Attack 2. Evade 3. Reload 1 You attacked the aliens and defeated 5 of them, and avoided taking damage! Player health: 20 Player ammo: 3 Aliens remaining: 13 Choose an action: 1. Attack 2. Evade 3. Reload 1 You attacked the aliens and defeated 1 of them, but took 5 damage! Player health: 15 Player ammo: 2 Aliens remaining: 12 Choose an action: 1. Attack 2. Evade 3. Reload 1 You attacked the aliens and defeated 4 of them, and avoided taking damage! Player health: 15 Player ammo: 1 Aliens remaining: 8 Choose an action: 1. Attack 2. Evade 3. Reload 1 You attacked the aliens and defeated 3 of them, and avoided taking damage! Player health: 15 Player ammo: 0 Aliens remaining: 5 Choose an action: 1. Attack 2. Evade 3. Reload 1 Unbelievable! You have run out of ammo and caused your own death! Game over! The aliens have defeated you! Do you want to play again? (Y/N)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
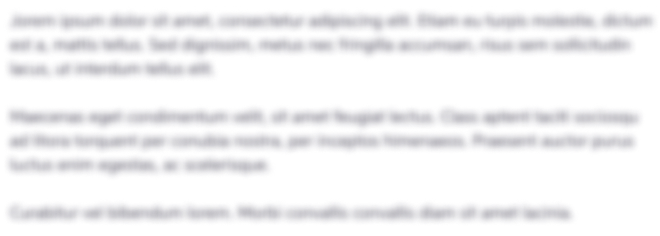
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started