Question
Hello, I've been having trouble with this assignment, and I would like some assistance. I can't seem to get it to run properly, and as
Hello, I've been having trouble with this assignment, and I would like some assistance. I can't seem to get it to run properly, and as per the assignment's rules, I've used classes when they apparently weren't allowed. I'll post my code beneath the question.
For these two labs (19 and 20) you are going to construct functionality to create a simple address book. Conceptually the address book uses a structure to hold information about a person and an array of structures to hold multiple persons (people).
When you get a person, you get the first person in the address book. With each successive call to get a person, you get the next person in the array. For instance the first call to get a person you will get "Joe Smith" when you make the second call to get a person you would get "Jane Doe" so on and so forth. After you get the last person from the array the next call to get a person will start over at the beginning ("Joe Smith" in this case).
Details
This lab is intended to prepare you to start working with object oriented programming. This means defining classes and creating objects. A class is an encapsulation of data and functions that operate on that data. An object is an instance of a class. Since we haven't covered how to create a class we are going to simulate it. How do we do that? Well hopefully it will be simple. First off we need to determine what the data section is. In this case it is going to be the array that holds the structs (people). Because the functions you write need to operate on the data in some way you are going to have to put this array in global scope. This way all of the functions have direct access to the array without it having to be passed around. One thing to note is that if we think about this from main's perspective, main should not know how the data is stored or what it is stored in. All main should know is that if you use the addPerson function you can add a person to the address book. This is what we call a public interface to private data. Since we are putting the array in global scope in addressBook.cpp we pseudo encapsulate it. This means that only addressBook functions have access to the array and variable in global scope. Of course since it is in global scope there is a way around this but again, we are simulating.
When you add a person struct to the end of the array you are going to need to keep track of where you put it. This way you have an idea of where the end of the array is so that you can add the next person. You are also going to need to do this for the beginning of the array so that you know where to get the next person from. This means that along with the array that you have in global scope you are also going to have to create a couple of int variables to be used as indexes to keep track of where you are in the array. A suggestion here would be to call these variables head and tail.
Ok, so you should have three files:
1. main.cpp - holds you main function
2. addressBook.cpp - holds the address book functions that are required for the assignment
3. addressBook.h - which holds the definitions for addressBook.cpp
Here is how things should flow for the addPerson function:
1) In main create a person struct
2) fill the person struct with information about a person. Get it from the keyboard if you want.
3) Pass the struct to addPerson which attempts to add the person to the next slot in the array.
4) If the address book is full and a person cannot be added then addPerson returns false
5) If the person was successfully added to the array addPerson returns true
Here is how things should flow for the getPerson function:
1) In main create a person struct
2) call getPerson passing the created struct by reference
3) If the addressBook is empty getPerson simply returns false
4) If the addressBook is not empty the next person in the addressBook is copied to the struct passed by reference and true is returned
5) *If the last person in the address book is returned back then the next call to getPerson will get the first person again.
*This is circular so if have 5 people in the address book and you call get person in a loop 25 times from main all five people will be returned five times.
Here is how the findPerson functions should flow:
1) In main fill a string with the last name (or strings with first and last names)
2) create a struct to hold the found person
3) pass the string to search for and the empty struct to findPerson
4) the findPerson function will search the array looking to match the string
5) If a match is found the found struct is copied to the struct passed by reference and the function returns true
6) If no match is found the function simply returns false.
Conceptually what you are creating is a circular queue that holds structs. Because you need to know where the next available spot in the array is you will need an index. This should be in the global section of addresBook.cpp. You are actually probably going to need two indexes, one to keep track of what is being added, and one that keeps track of what is being removed. There are four functions and I can tell you that each of these can be written in about 5 or 6 lines of code.
What not to do
Using any of the following will drop your grade for this assignment by 70%:
cin in any funciton other than main
*cout in any funciton other than main
passing the array to the addPerson, getPerson, and findPerson functions
*If you want to create a function called printBook it is acceptable to have cout in that funciton but not in any others.
What to submit
For this assignment you are going to submit 3 files by uploading these into Canvas using the assignment link:
main.cpp
addressBook.cpp
addressBook.h
Canvas will only accept cpp and h files so don't try to submit any other kind of file.
No text submission for this assignment, only upload the 3 files requested.
addressbook.cpp
#include "addressBook.h"
#include
using namespace std;
int counter = 0;
int getcount = 0;
using namespace std;
void printStruct(struct Person &print);
int main()
{
char last[20];
char first[20];
char choice;
AddressBook aBook;
Person add;
Person getStruct;
cout << "Address Book" << endl << endl;
while (true)
{
cout << endl << " Enter 1 to add to the address book. " << endl;
cout << " Enter 2 to get a person. " << endl;
cout << " Enter 3 to find a person by last name only." << endl;
cout << " Enter 4 to find a person by last name and first. " << endl;
cout << " Enter 5 to print Book." << endl;
cout << " Enter any other key to quit. " << endl;
cout << endl << "Please make a selection: " << endl;
cin >> choice;
switch (choice)
{
case '1':
cout << "Enter First Name" << endl;
cin >> add.firstname;
cout << "Enter Last Name" << endl;
cin >> add.lastname;
cout << "Enter Address" << endl;
cin.getline(add.address, 40);
cin >> add.address;
aBook.addPerson(add);
continue;
case '2':
cout << endl << "Getting the next person in the address book: " << endl << endl;
aBook.getPerson(getStruct);
printStruct(getStruct);
continue;
case '3':
cout << "Please enter the last name to search for: " << endl;
cin >> last;
if (aBook.findPerson(last, add))
printStruct(add);
else
cout << "Sorry could not find the name." << endl;
continue;
case '4':
{
cout << "Please enter the last name and then the first name to search for: " << endl;
cout << "Last name: ";
cin >> last;
cout << "First name: ";
cin >> first;
if (aBook.findPerson(last, first, add))
{
printStruct(add);
}
else
{
cout << "Sorry could not find the name." << endl;
}
continue;
}
case '5':
{
aBook.printBook();
continue;
}
default:
{
return 0;
break;
}
}
}
return 0;
}
void printStruct(struct Person &print)
{
cout << print.firstname << " " << print.lastname << endl;
cout << print.address << endl;
}
addressbook.h [This is where the class items are, and I'm unsure how to change them into an acceptable format for this assignment]
#ifndef addressBook
#define addressBook
#include
const int MAXIMUM = 10;
struct Person
{
char firstname[20];
char lastname[20];
char address[50];
};
class AddressBook
{
private:
Person person[MAXIMUM];
int count;
public:
AddressBook();
AddressBook(char* fName, char *lName, char *address);
AddressBook(const Person &p);
AddressBook(const Person p[], int size);
bool addPerson(const Person p);
bool addPerson(const char *fName, const char *lName, const char *phone);
bool getPerson(Person &p);
bool getPerson(char *fName, char *lName, char *Phone);
bool findPerson(char *lastName, Person &p);
bool findPerson(char *lastName, char *firstName, Person &p);
void printBook();
void sort();
Person& operator() (int i);
};
#endif
main.cpp
#include
#include "addressBook.h"
using namespace std;
static int head = 0;
static int tail = -1;
AddressBook::AddressBook()
{
}
AddressBook::AddressBook(const Person &p)
{
addPerson(p);
}
AddressBook::AddressBook(const Person p[], int size)
{
for (int i = 0; i < size; i++)
addPerson(p[i]);
}
AddressBook::AddressBook(char *fName, char *lName, char *address)
{
Person tmp;
strcpy_s(tmp.firstname, fName);
strcpy_s(tmp.lastname, lName);
strcpy_s(tmp.address, address);
addPerson(tmp);
}
bool AddressBook::addPerson(const Person p)
{
if (head < MAXIMUM)
{
person[head] = p;
head++;
if (tail == -1)
tail++;
return true;
}
return false;
}
bool AddressBook::getPerson(Person &p)
{
if (tail >= 0)
{
if (tail >= MAXIMUM)
tail = 0;
p = person[tail];
tail++;
return true;
}
return false;
}
bool AddressBook::findPerson(char *lName, Person &p)
{
for (int i = 0; i < head; i++)
{
if (!(person[i].lastname, lName))
{
p = person[i];
return true;
}
}
return false;
}
bool AddressBook::findPerson(char *lastName, char *firstName, Person &p)
{
for (int i = 0; i < head; i++)
{
if (!(person[i].lastname, lastName) && !(person[i].firstname, firstName))
{
p = person[i];
return true;
}
}
return false;
}
void AddressBook::printBook()
{
for (int i = 0; i < head; i++)
{
cout << person[i].firstname << "\t" << person[i].lastname << "\t" << person[i].address << endl;
}
}
void AddressBook::sort()
{
Person temp;
for (int i = 0; i < head; i++)
{
for (int j = 0; j < head; j++)
{
if ((person[j + 1].lastname, person[j].lastname) < 0)
{
temp = person[j];
person[j] = person[j + 1];
person[j + 1] = temp;
}
}
}
}
ostream& operator<<(ostream& output, const Person& p) {
output << "(" << p.firstname << ", " << p.lastname << ")";
return output;
}
Person& AddressBook::operator() (int i)
{
return person[i];
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
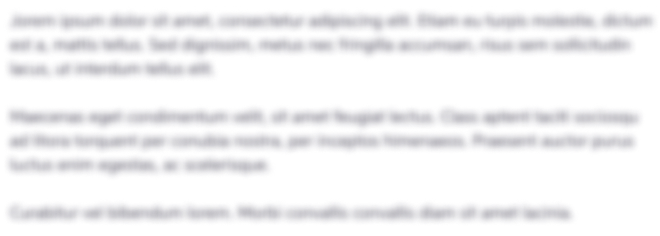
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started