Question
Hello, my question is I have this line of code me and a friend worked on and we are suppose to be able to go
Hello, my question is I have this line of code me and a friend worked on and we are suppose to be able to go back in and add more to the code but our professor looked over what we had and said it would be hard to go back in and change and add to the code next week when we add to the game more features and buttons and such. it is a treasure hunt game the game turns out to look like this
https://cdn.discordapp.com/attachments/285590814934171649/386148724440563712/TreasureHunt_Start.png
i posted the url for the pic because for some odd reason chegg wont let me upload the picture
My professor specifically said this
"Can you avoid using instanceof? for instance by looking at what is on the button? like displayText or other? It's considered a bad practice because next week you might implement another button of a different type and then this structure will not work correctly.
You could also place ActionListeners in the constructors of the button classes for disabling and changing look when the button are clicked."
can you please help by altering the code below to his preference and pointing out what was changed in the code so i can understand and learn from what you change up.
i assume what we did is pretty much we made the code in like a wrong way that we cant go back and change stuff so the question pretty much is if you can fix that for me so i can see how it was suppose to be or what my professor wants us to do and show me what was changed just to explain a bit more if i was being confusing with what i am asking for
/////////////////////////////////////////////////////////////////////////////////////////////////
The code is below and is a new class at each .java comment
///////////////////////////////////////////////////////////////////////////////////////////////
// BoardButton.java
import java.awt.Dimension;
import javax.swing.JButton;
import javax.swing.SwingConstants;
public class BoardButton extends JButton {
// Instance variables
private String displayText; //the displayText variable is used to hold the strings text
/**
* Default Constructor
*/
public BoardButton() {
this("O");
}
/**
* Parameterized Constructor
*/
public BoardButton(String text) {
super();
// Set text
this.displayText = text;
// Set text alignment
setHorizontalAlignment(SwingConstants.CENTER);
// Set size
setPreferredSize(new Dimension(60, 40));
}
/**
* @return the text
*/
public String getDisplayText() {
return displayText;
}
}
//TreasureButton.java
public class TreasureButton extends BoardButton { /** * Constructor */ public TreasureButton() { super("$$$");
}
}
// TreasureBoardPanel.java
import java.awt.GridLayout;
import java.util.Random;
import javax.swing.JPanel;
public class TreasureBoardPanel extends JPanel {
private static final int WIDTH = 10;
private static final int HEIGHT = 10;
// Instance variables
private BoardButton[] button; //variable used to add buttons to the panel
private TreasureGame game; //used to make the constructor for the TreasureBoardPanel
/**
* Constructor
*/
public TreasureBoardPanel(TreasureGame game) {
super();
this.game = game;
// Set layout
setLayout(new GridLayout(WIDTH, HEIGHT));
// Add buttons
addButtons();
}
/**
* Creates and adds buttons to the panel
*/
private void addButtons() {
this.button = new BoardButton[WIDTH * HEIGHT];
// Create random treasure buttons
Random r = new Random();
for (int i = 0; i < TreasureGame.NUM_TREASURES; i++) {
int index = r.nextInt(this.button.length);
// Check if index is already a button
while (this.button[index] != null)
index = r.nextInt(this.button.length);
this.button[index] = new TreasureButton();
}
// For all the buttons which are not TreasureButton i.e. null buttons
// create BoardButton
for (int i = 0; i < this.button.length; i++) {
if (this.button[i] == null)
this.button[i] = new BoardButton();
}
// Add all buttons to the panel and add action listener
for (int i = 0; i < this.button.length; i++) {
this.button[i].addActionListener(new ButtonListener(game));
add(this.button[i]);
}
}
/**
* Displays text on all treasure buttons
*/
public void displayAllTreasures() {
for (int i = 0; i < this.button.length; i++) {
if (this.button[i] instanceof TreasureButton)
this.button[i].setText(this.button[i].getDisplayText());
}
}
}
//StatsPanel.java
import java.awt.Dimension;
import javax.swing.Box;
import javax.swing.BoxLayout;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class StatsPanel extends JPanel {
private static final String treasuresLeftStr = "Treasures left: %d";
private static final String treasuresFoundStr = "Treasures found: %d";
private static final String triesLeftStr = "Tries left: %d";
// Instance variables
//used to hold the variable of how much treasure is left private int treasuresLeft; //holds how much treasure is found by the user when playing private int treasuresFound; //holds how many tries left
private int triesLeft;
private JLabel treasuresLeftLbl;
private JLabel treasuresFoundLbl;
private JLabel triesLeftLbl;
/**
* Constructor
*/
public StatsPanel() {
super();
// Set size
setPreferredSize(new Dimension(200, 100));
// Set layout
setLayout(new BoxLayout(this, BoxLayout.Y_AXIS));
// Set defaults
treasuresLeft = TreasureGame.NUM_TREASURES;
treasuresFound = 0;
triesLeft = TreasureGame.NUM_TRIES;
// Create labels
treasuresLeftLbl = new JLabel(
String.format(treasuresLeftStr, treasuresLeft));
treasuresFoundLbl = new JLabel(
String.format(treasuresFoundStr, treasuresFound));
triesLeftLbl = new JLabel(String.format(triesLeftStr, triesLeft));
// Add labels
add(Box.createVerticalGlue());
add(treasuresLeftLbl);
add(treasuresFoundLbl);
add(triesLeftLbl);
add(Box.createVerticalGlue());
}
/**
* Increases/Decreases treasure found by 1 based on treasureFound
*/
public void updateTreasureFound(boolean treasureFound) {
if (treasureFound) {
treasuresFound += 1;
treasuresFoundLbl
.setText(String.format(treasuresFoundStr, treasuresFound));
treasuresLeft -= 1;
treasuresLeftLbl
.setText(String.format(treasuresLeftStr, treasuresLeft));
}
// Update tries
updateTriesLeft();
}
/**
* Decreases tries left by 1
*/
private void updateTriesLeft() {
triesLeft -= 1;
triesLeftLbl.setText(String.format(triesLeftStr, triesLeft));
}
/**
* @return the treasuresLeft
*/
public int getTreasuresLeft() {
return treasuresLeft;
}
/**
* @return the triesLeft
*/
public int getTriesLeft() {
return triesLeft;
}
}
//TreasureGame.java
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.SwingConstants;
public class TreasureGame extends JFrame {
// Number of tries
protected static final int NUM_TRIES = 50;
// Number of treasures
protected static final int NUM_TREASURES = 20;
// Last move
private static final String lastMove = "Last move: %s";
// Instance variables
private JLabel lastMoveLbl;
private StatsPanel statsPanel;
private TreasureBoardPanel boardPanel;
private boolean gameOver;
/**
* Constructor
*/
public TreasureGame() {
super("Treasure Hunt Game");
// Set layout
setLayout(new BorderLayout());
// Set default close operation
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Show frame
setVisible(true);
}
/**
* Starts the game
*/
public void play() {
gameOver = false;
// Add components
addComponents();
// Resize frame to the components
pack();
}
/**
* Adds all the required panels to the frame
*/
private void addComponents() {
// Create game header label
JLabel headerLbl = new JLabel("Treasure Hunt");
headerLbl.setPreferredSize(new Dimension(60, 40));
headerLbl.setHorizontalAlignment(SwingConstants.CENTER);
// Add label
add(headerLbl, BorderLayout.NORTH);
// Add stats panel
statsPanel = new StatsPanel();
add(statsPanel, BorderLayout.WEST);
// Add board panel
boardPanel = new TreasureBoardPanel(this);
add(boardPanel, BorderLayout.CENTER);
// Add last move label
lastMoveLbl = new JLabel(String.format(lastMove, ""));
lastMoveLbl.setPreferredSize(new Dimension(60, 40));
lastMoveLbl.setHorizontalAlignment(SwingConstants.CENTER);
add(lastMoveLbl, BorderLayout.SOUTH);
}
/**
* Updates UI based on the move
*/
public void updateTreasureFound(boolean treasureFound) {
// Update stats panel
statsPanel.updateTreasureFound(treasureFound);
// Update last move
String move = "";
if (statsPanel.getTreasuresLeft() == 0) {
move = String.format(lastMove, "Game over - You win");
gameOver = true;
} else if (statsPanel.getTriesLeft() == 0) {
move = String.format(lastMove, "Game over - You lose");
gameOver = true;
} else {
if (treasureFound)
move = String.format(lastMove, "Treasure");
else
move = String.format(lastMove, "Miss");
}
// Set last move label
lastMoveLbl.setText(move);
// If game is over show all button text
if (gameOver && statsPanel.getTreasuresLeft() != 0)
boardPanel.displayAllTreasures();
}
/**
* @return the gameOver
*/
public boolean isGameOver() {
return gameOver;
}
}
class ButtonListener implements ActionListener {
// Instance variables
private TreasureGame game;
/**
* Constructor
*/
public ButtonListener(TreasureGame game) {
this.game = game;
}
/**
* Handles the button click event
*/
@Override
public void actionPerformed(ActionEvent ae) {
// Check if game is not over
if (!game.isGameOver()) {
// Get source
BoardButton btn = (BoardButton) ae.getSource();
// Set text
btn.setText(btn.getDisplayText());
// Check if btn is a treasure button
if (btn instanceof TreasureButton)
game.updateTreasureFound(true);
else
game.updateTreasureFound(false);
// Set disabled
btn.setEnabled(false);
}
}
}
//TreasureGUI.java
public class TreasureGameGUI { public static void main(String[] args) //main class used to play the game { new TreasureGame().play(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
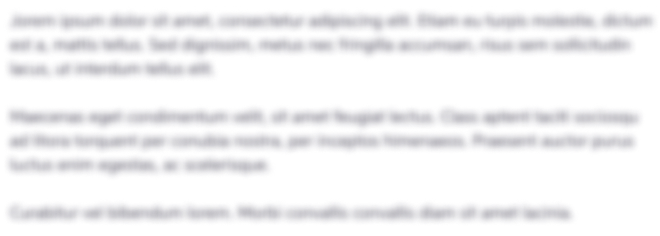
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started