Question
Hello need help with this lab, thank you for your help, Im leaving the program and the test import java.io.*; import java.util.*; /** * StackSort
Hello need help with this lab, thank you for your help, Im leaving the program and the test
import java.io.*; import java.util.*;
/** * StackSort is a program that will use two stacks to sort an array of integer values. * * @author Charles Hoot * @version 5.0 */ public class StackSort {
public static void main(String args[]) {
int data[] = null; int result[] = null;
Scanner input; input = new Scanner(System.in);
System.out.println("This program sorts an array of integer values.");
// Create an empty array of integers data = createArray(0, 1, 1); System.out.println("Original array is: " + representationOfArray(data)); result = doStackSort(data); System.out.println("Sorted array is: " + representationOfArray(result)); System.out.println();
// Create an array with one integer data = createArray(1, 0, 9); System.out.println("Original array is: " + representationOfArray(data)); result = doStackSort(data); System.out.println("Sorted array is: " + representationOfArray(result)); System.out.println();
// Create an array with two integers data = createArray(2, 0, 9); System.out.println("Original array is: " + representationOfArray(data)); result = doStackSort(data); System.out.println("Sorted array is: " + representationOfArray(result)); System.out.println();
// Create an array with 10 integers data = createArray(10, 0, 9999); System.out.println("Original array is: " + representationOfArray(data)); result = doStackSort(data); System.out.println("Sorted array is: " + representationOfArray(result)); System.out.println();
// Create an array with 20 integers data = createArray(20, 0, 9); System.out.println("Original array is: " + representationOfArray(data)); result = doStackSort(data); System.out.println("Sorted array is: " + representationOfArray(result)); System.out.println();
System.out.println("Please enter the number of values to sort"); int size = getInt(" It should be an integer value greater than or equal to 1."); // Create an array of the given size
data = createArray(size, 0, 99); System.out.println("Original array is: " + representationOfArray(data)); result = doStackSort(data); System.out.println("Sorted array is: " + representationOfArray(result)); System.out.println();
// animatedStackExample(createArray(10, 0, 99)); System.exit(0); }
/** * Use two stacks to sort the data in an array * * @param data An array of integer values to be sorted. * @return An array of sorted integers. */ public static int[] doStackSort(int data[]) {
int result[] = new int[data.length];
// ADD CODE HERE TO SORT THE ARRAY USING TWO STACKS
return result;
}
/** * Load an array with data values * * @param size The number of data values to generate and place in the array. * @param min The minimum value to generate. * @param max The maximum value to generate. * @return An array of randomly generated integers. */ public static int[] createArray(int size, int min, int max) {
Random generator = new Random();
// If we get a negative size, just make the size 1 if (size min for the random number generator to be happy
if (max
int[] data = new int[size];
for (int i = 0; i
return data; }
/** * Create a string with the data values from an array * * @param data An array of integer values. * @return A string representation of the array. */ private static String representationOfArray(int data[]) { String result = new String("";
return result; }
/** * Get an integer value * * @return An integer. */ private static int getInt(String rangePrompt) { Scanner input; int result = 10; // default value is 10 try { input = new Scanner(System.in); System.out.println(rangePrompt); result = input.nextInt();
} catch (NumberFormatException e) { System.out.println("Could not convert input to an integer"); System.out.println(e.getMessage()); System.out.println("Will use 10 as the default value"); } catch (Exception e) { System.out.println("There was an error with System.in"); System.out.println(e.getMessage()); System.out.println("Will use 10 as the default value"); } return result;
} }
import static org.junit.jupiter.api.Assertions.*;
import java.util.Scanner;
import org.junit.jupiter.api.Test;
class StackSortTest {
private static boolean check(int[] unsorted, int[] sorted) { if (unsorted == null || sorted == null) return false; else if (unsorted.length != sorted.length) return false; else if (unsorted.length > 0) { int last = Integer.MIN_VALUE; for (int i : sorted) { if (i
@Test void testDoStackSort() { int[] data, result;
data = StackSort.createArray(0, 1, 1); result = StackSort.doStackSort(data); assertTrue(check(data, result));
data = StackSort.createArray(1, 0, 9); result = StackSort.doStackSort(data); assertTrue(check(data, result));
data = StackSort.createArray(2, 0, 9); result = StackSort.doStackSort(data); assertTrue(check(data, result));
data = StackSort.createArray(10, 0, 9999); result = StackSort.doStackSort(data); assertTrue(check(data, result)); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
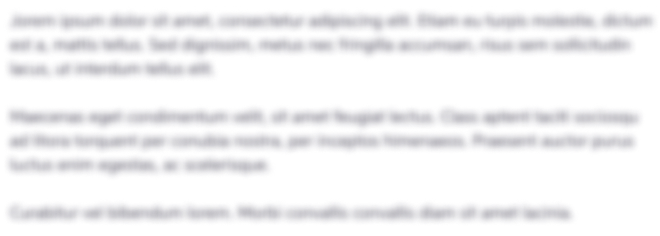
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started