Question
Hello Please help me with Game of life code for java in very basic way. Those three are the patterns of the games. Rules of
Hello Please help me with Game of life code for java in very basic way. Those three are the patterns of the games. Rules of the game
Birth : A dead cell with exactly three live neighbors becomes a live cell
Survival: A live cell with two or three neighbors stays alive
Overcrowding/Loneliness In all other cases a cell dies or remains dead
Birth : A dead cell with exactly three live neighbors becomes a live cell
Survival: A live cell with two or three neighbors stays alive
Overcrowding/Loneliness In all other cases a cell dies or remains dead
I need to figure out getNeighbours, edge. user needs to ask for pattern, # of generation, sleep time. I need it by tonight. Please help me, Thank you!
import java.util.Scanner; import java.util.Random; public class Life { private char[][] world; private char[][] glider = { { ' ', '*', ' ' }, { ' ', '*', '*' }, { '*', ' ', '*' } }; private char[][] patternB = { { '*', '*', ' ' }, { '*', ' ', '*' }, { ' ', '*', '*' } }; private char[][] patternC = { { ' ', ' ', ' ' }, { ' ', '*', '*' }, { ' ', '*', '*' } }; int dimension = 10; // char[][] world = new char[20][20]; long generation; public void putGlider(int r_pos, int c_pos) { for (int x = 0; x < glider.length; x++) { for (int y = 0; y < glider[0].length; y++) { world[x + r_pos][y + c_pos] = glider[x][y]; } } } public boolean isInbounds(int r, int c) { if (r >= 0 && c >= 0 && r < world.length && c < world[0].length) { return true; } else { return false; } } Life(int dimension) { world = new char[dimension][dimension]; for (int x = 0; x < dimension; x++) { for (int y = 0; y < dimension; y++) { world[x][y] = ' '; } } // dimension = nextDimension; // createNewWorld(); // nextGeneration = 0; } // Contains the logic for the starting scenario. // Which cells are alive or dead in generation 0. void createNewWorld() { char[][] newWorld = new char[dimension][dimension]; for (int row = 0; row < newWorld.length; row++) { for (int col = 0; col < newWorld[row].length; col++) { } } world = newWorld; } // Draws the world void drawWorld() { for (int row = 0; row < world.length; row++) { for (int col = 0; col < world[row].length; col++) { System.out.print(world[row][col] == '*' ? '@' : '.'); System.out.print(' '); } System.out.println(); } System.out.println("Generation:" + generation); } // Create the next generation // // // void nextGeneration() { char[][] newWorld = new char[dimension][dimension]; for (int row = 0; row < newWorld.length; row++) { for (int col = 0; col < newWorld[row].length; col++) { /* * + Any live cell with fewer than two live neighbours dies, as if by needs * caused by underpopulation. + Any live cell with more than three live * neighbours dies, as if by overcrowding. + Any live cell with two or three * live neighbours lives, unchanged, to the next generation. + Any dead cell * with exactly three live neighbours cells will come to life. + */ if (world[row][col] == '*' && getNeighbors(row, col) < 2) { newWorld[row][col] = ' '; } else if (world[row][col] == '*' && getNeighbors(row, col) > 3) { newWorld[row][col] = ' '; } else if (world[row][col] == '*') { newWorld[row][col] = '*'; } if (world[row][col] == ' ' && getNeighbors(row, col) == 3) { newWorld[row][col] = '*'; } else if (world[row][col] == ' '){ newWorld[row][col] = ' '; } // newWorld[row][col] = isAlive(row, col); } } world = newWorld; generation++; } // Calculate if an individual cell should be alive in the next generation. // Based on the game logic: int getNeighbors(int row, int col) { int liveCount = 0; for (int r = -1; r <= 1; r++) { int currentRow = row + r; currentRow = (currentRow < 0) ? dimension - 1 : currentRow; currentRow = (currentRow >= dimension) ? 0 : currentRow; for (int c = -1; c <= 1; c++) { int currentCol = col + c; currentCol = (currentCol < 0) ? dimension - 1 : currentCol; currentCol = (currentCol >= dimension) ? 0 : currentCol; if(!(currentRow == row && currentCol == col) && world[currentRow][currentCol] == '*'){ liveCount++; } } } return liveCount; } public static void main(String[] args) throws java.lang.InterruptedException { Scanner in = new Scanner(System.in); Life earth = new Life(10); earth.putGlider(4, 4); boolean choice = true; do { earth.drawWorld(); earth.nextGeneration(); System.out.println("Do you want to generate next generation(y/n): "); choice = !in.nextLine().trim().equalsIgnoreCase("n"); } while(choice); in.close(); } }
public class LifeUtils { public static boolean isEdge(char[][] w, int r, int c) { //it it in an edge ? if((r == 0) || (r == w.length-1) || (c == 0) || (c == w.length-1)) return true; else return false; } public static boolean isTopEdge(int r, int c) { if(r == 0) return true; else return false; } public static boolean isBottomEdge(int r, char[][] w) { if(r == w.length-1) return true; else return false; } public static boolean isLeftEdge(int c) { if(c == 0) return true; else return false; } public static boolean isRightEdge(int c, char[][] w) { if(c == w.length-1) return true; else return false; } }
public class SleepExample { public static void main(String args []) { //default sleep for one second long sleepTime = 1000; //get commnd line time if entered if(args.length == 1) { sleepTime = Long.parseLong(args[0]); } //start sleep() example while(true) { System.out.println("Sleeping ..... Ctrl-C to exit"); try { Thread.sleep(sleepTime); } catch(Exception ex) { System.out.println("Exception:"+ex.getStackTrace()); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
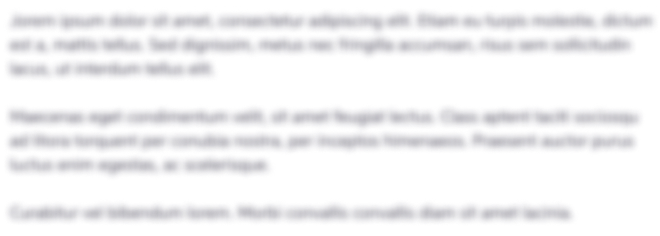
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started