Question
Hello, Please, I need your help with this assignment, it is in Java and using eclipse and cmd. I need to implement the tests for
Hello, Please, I need your help with this assignment, it is in Java and using eclipse and cmd.
I need to implement the tests for the public methods of BoggleDictionary
The tests must use the TestData.java class.
The BoggleDictionary constructor takes a BufferedReader reference to ingest the words. You use the TestData class like follows:
int expectedSize = TestData.count(); // the expected number of words String[] expectedList = TestData.list(); // the list of words StringReader sReader = new StringReader(TestData.getTestData()); // converts the TestData to a Reader BufferedReader reader = new BufferedReader(sReader); // pass this to the constructor
Use this at the start of your individual tests to create the BoggleDictionary instance to evaluate.
After creating the Test methods (uses the @Test attribute), you run the test by selecting Run As | JUnit Test
Using the JUnit built in Eclipse libraries won't work for this assignment.
The JUnitBoggleTest.java utilizes JUnit 5 and command line execution which is only supported by the following junit files.
hamcrest-core-1.3.jar
junit.jar
/**************This is the class I want to implement, I implemented the first**********/
package week08;
import static org.junit.Assert.*;
import org.junit.Test;
public class JUnitBoggleTest
{
@Test// I implemented the first one
public void testBoggleDictionary()
{
int expectedSize = TestData.count(); // the expected number of words
String[] expectedList = TestData.list(); // the list of words
StringReader sReader = new StringReader(TestData.getTestData()); // converts the TestData to a Reader
BufferedReader reader = new BufferedReader(sReader); // pass this to the constructor
try
{
BoggleDictionary bd = new BoggleDictionary(reader);
}
catch (AtmException ex)
{
String msg = " Failed: testBoggleDictionary " + ex.getMessage();
//trace(msg);
fail(msg);
}
}
@Test
public void testGetWords()
{
fail("Not yet implemented");
}
@Test
public void testSize()
{
fail("Not yet implemented");
}
@Test
public void testIsValid()
{
fail("Not yet implemented");
}
@Test
public void testIsWord()
{
fail("Not yet implemented");
}
}
/*************************************/
package week08;
import java.io.BufferedReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
/**
* This class provides storage for words. It loads the dictionary provided via a
* BufferedReader to the constructor. It creates a set of hash tables that are
* indexed by 1 to 16 letters Pass in a single character and it will return all
* words starting with the character, pass in two and you get words only
* starting with the first two
*
* The class provides a set of methods to query on whether a word is in the
* dictionary, if it is a partial word, or not found.
*
*
* @author Dina
*
*/
public class BoggleDictionary
{
/**
* Constructor to initialize the dictionary Reads a list of words from the
* stream, one word per line
*
* @param reader
* Text reader.
*/
public BoggleDictionary(BufferedReader reader) throws AtmException
{
initialize(reader);
}
/**
* Returns the list of words from the dictionary
*
* @return List of words
*/
public List
{
return new ArrayList
}
/**
* Returns the number of words in the dictionary
*
* @return count of words in the dictionary
*/
public int size()
{
return m_dictionary.values().size();
}
/**
* Checks if the string is part of a valid word.
*
* @param partial
* string to test
* @return true if the string is part of a word, otherwise false
*/
public boolean isValid(String partial)
{
WordEntry entry = getMapType(partial.length()).get(partial);
// if entry exists it is valid
return entry == null ? false : true;
}
/**
* Checks if the string is a valid word.
*
* @param testWord word to test
* @return true if the string is a word, otherwise false
*/
public boolean isWord(String testWord)
{
WordEntry entry = m_dictionary.get(testWord);
if(entry == null)
{
return false;
}
else
{
return entry.getIsWord();
}
}
/**
* Initializes the dictionary
*
* @param reader
* List of words to ingest
* @throws AtmException
* on error
*/
private void initialize(BufferedReader reader) throws AtmException
{
String word = "";
m_dictionary = new HashMap
m_oneCharMap = new HashMap
m_twoCharMap = new HashMap
m_threeCharMap = new HashMap
m_fourCharMap = new HashMap
m_fiveCharMap = new HashMap
m_sixCharMap = new HashMap
m_sevenCharMap = new HashMap
m_eightCharMap = new HashMap
m_nineCharMap = new HashMap
m_tenCharMap = new HashMap
m_elevenCharMap = new HashMap
m_twelveCharMap = new HashMap
m_thirteenCharMap = new HashMap
m_fourteenCharMap = new HashMap
m_fifteenCharMap = new HashMap
m_sixteenCharMap = new HashMap
m_mapList = new ArrayList
m_mapList.add(m_oneCharMap);
m_mapList.add(m_twoCharMap);
m_mapList.add(m_threeCharMap);
m_mapList.add(m_fourCharMap);
m_mapList.add(m_fiveCharMap);
m_mapList.add(m_sixCharMap);
m_mapList.add(m_sevenCharMap);
m_mapList.add(m_eightCharMap);
m_mapList.add(m_nineCharMap);
m_mapList.add(m_tenCharMap);
m_mapList.add(m_elevenCharMap);
m_mapList.add(m_twelveCharMap);
m_mapList.add(m_thirteenCharMap);
m_mapList.add(m_fourteenCharMap);
m_mapList.add(m_fifteenCharMap);
m_mapList.add(m_sixteenCharMap);
try
{
while((word = reader.readLine()) != null)
{
word = word.trim();
if(!word.equals(""))
{
// m_dictionary.put(word, new WordEntry(word));
initializeWord(word);
}
}
}
catch(IOException ex)
{
throw new AtmException("Unexpected error reading data", ex);
}
}
private void initializeWord(String word)
{
StringBuilder builder = new StringBuilder();
HashMap
for(int index = 0; index < word.length(); index++)
{
char ch = word.charAt(index);
builder.append(ch);
String key = builder.toString();
int len = key.length();
boolean isWord = len == word.length(); // only words are passed in.
map = getMapType(len);
if(map != null && !map.containsKey(key))
{
map.put(key, new WordEntry(key, isWord));
}
if(isWord)
{
m_dictionary.put(word, new WordEntry(word, true));
}
}
}
private HashMap
{
if(index <= MAX_WORD_LEN)
return m_mapList.get(index - 1);
else
return null;
}
private HashMap
private HashMap
private HashMap
private HashMap
private HashMap
private HashMap
private HashMap
private HashMap
private HashMap
private HashMap
private HashMap
private HashMap
private HashMap
private HashMap
private HashMap
private HashMap
private HashMap
private List
private static int MAX_WORD_LEN = 16;
}
class WordEntry
{
/**
* Creates a new word entry. The word may be a partial word. Defaults to
* false as a full word.
*
* @param wordPart
* Partial word string to identify
*/
WordEntry(String wordPart)
{
this(wordPart, false);
}
/**
* Creates a new word entry. The word may be a partial word oe a full word
* User specifies if the word is a full word by passing true
*
* @param wordPart
* Partial word string to identify
* @param isWord
* true if a word, otherwise false indicating a partial word
*/
WordEntry(String wordPart, boolean isWord)
{
m_wordPart = wordPart;
m_isWord = isWord;
}
// Getters
/**
* Gets the partial or complete word string
*
* @return The partial word or word
*/
public String getWordPart()
{
return m_wordPart;
}
/**
* Gets the partial or complete word string
*
* @return The partial word or word
*/
public boolean getIsWord()
{
return m_isWord;
}
// Setters
/**
* Sets whether the word is a full word or partial word.
*
* @param isWord
* true is a full word, false is a partial word
*/
public void setIsWord(boolean isWord)
{
m_isWord = isWord;
}
/**
* Provides a formatted representation of the state of WordEntry
*/
@Override
public String toString()
{
// TODO Auto-generated method stub
return String.format("%s - %s", m_wordPart, m_isWord);
}
private String m_wordPart;
private boolean m_isWord;
}
/*************************************/
package week08;
public class TestData
{
/**
* This returns a string where the each word is on it's own line
*
* @return List of words in a string
*/
public static String getTestData()
{
return builder.toString();
}
/**
* Gets the number of words in the test data
* @return number of words
*/
public static int count()
{
return m_count;
}
/**
* Retrieves the array of words
* @return String array of words in the test data
*/
public static String[] list()
{
return m_list;
}
private static String m_testWords = "apple,beta,bravo,charlie,faith,people,stupendous,holiday,holidays,journey,alpha,domino,tremendous,advantage,adverb,zebra,zelda,yankee"
+ ",forever,for,ever,brief,spin,process,advance,power,protocol,pete,pat,tap,tape,taps,pats,patsy,gypsy";
private static StringBuilder builder;
private static int m_count = 0;
private static String[] m_list;
static
{
builder = new StringBuilder();
m_list = m_testWords.split(",");
// build the text string
for(String word : m_list)
{
word = word.trim();
if(word.length() != 0)
{
builder.append(word);
builder.append(System.lineSeparator());
m_count++;
}
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
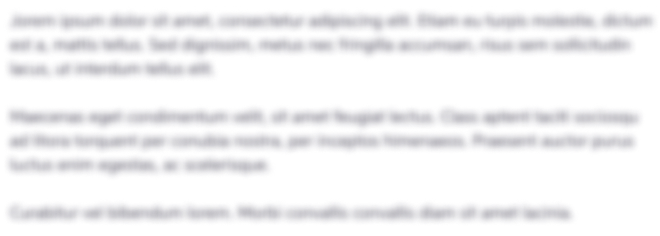
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started