Question
Hello there, this is C++, wondering if someone could help. I will make sure to upvote #include #include #include // std::string, std::to_string, std::fixed #include //
Hello there, this is C++, wondering if someone could help. I will make sure to upvote
#include
#include
#include
#include
using namespace std;
class Car
{
private:
float mileage; // Total cumulative mileage traveled by the Car
float gasInTank; // Total gas in gallons held in the Car's tank
float milesPerGallon; // Car's gas mileage in miles per gallon (mpg)
float tripMileage; // Mileage traveled by Car on its last trip
float tripGasConsumed; // Gallons of gas consumed by Car during last trip
public:
// Constructors
/**
* Car instance with an initial milesPerGallon (mpg).
* The other parameters are explicitly set to 0.0;
*/
Car(float mpg) {
milesPerGallon = mpg;
mileage = 0.0;
gasInTank = 0.0;
tripMileage = 0.0;
tripGasConsumed = 0.0;
}
// Constructor declared
Car(float mileage, float gasInTank, float milesPerGallon);
// Getters
float getMileage() {return mileage;}
float getGasInTank() {return gasInTank;}
float getMilesPerGallon() {return milesPerGallon;}
float getTripMileage() {return tripMileage;}
float getTripGasConsumed() {return tripGasConsumed;}
// Setters
void setMileage(double mileage){this->mileage = mileage;}
void setGasInTank(double gasInTank);
void setMilesPerGallon(double milesPerGallon);
void setTripMileage(double tripMileage);
void setTripGasConsumed(double tripGasConsumed) {this->tripGasConsumed = tripGasConsumed;}
// DO NOT EDIT OR CHANGE THIS METHOD
void display() // Used to display test case results
{
cout << fixed;
cout << setprecision(2);
cout << "Mileage: " << this->getMileage();
cout << " / Gas in tank: " << this->getGasInTank();
cout << " / MPG: " << this->getMilesPerGallon();
cout << " / Trip Mileage: " << this->getTripMileage();
cout << " / Trip Gas: " << this->getTripGasConsumed() << endl;
}
// Instance methods provided
//Add the given gallons of gas to the target Car
void pumpGas(float gallons) { gasInTank += gallons;}
//Resets both the trip mileage and trip gas consumed
//to 0 to start measuring the next (new) trip.
void resetTrip() {
tripMileage = 0;
tripGasConsumed = 0;
}
// Methods declared
Car mostFilled(Car &c2);
float fillToDistance(float miles, float gasCost);
void fillPayment(float moneyPaid, float gasCost);
float sellCar();
};
int main(){
cout << "HELLO FROM CAR CLASS" << endl;
cout << "---TEST CASES BELOW---" << endl;
//Example of Test Cases
//Exercise #3
Car Car31 = Car(30);
cout << Car31.fillToDistance(200,2) << endl;
//Expected Result
cout << "13.33" << endl;
cout << endl;
//Exercise #4
Car Car41 = Car(30);
Car41.fillPayment(5,1.95);
Car41.display();
//Expected Result
cout << "Mileage: 0.00 / Gas in tank: 2.56 / MPG: 30.00 / Trip Mileage: 0.00 / Trip Gas: 0.00" << endl;
cout << endl;
return 0;
}
/*********************************************************************
* EXERCISE #3
*
* Fill the gas tank of the target car to reach a specific given distance
* and return the amount of payment.
* The parameter gasCost is in dollars per gallon.
*/
float Car::fillToDistance(float miles, float gasCost){
// TODO -- YOUR CODE HERE
return 100; // Dummy return
}
/*********************************************************************
* EXERCISE #4
*
* Fill the gas tank of the target car upto a given amount of money.
* The parameter gasCost is in dollars per gallon.
*/
void Car::fillPayment(float moneyPaid, float gasCost){
// TODO -- YOUR CODE HERE
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
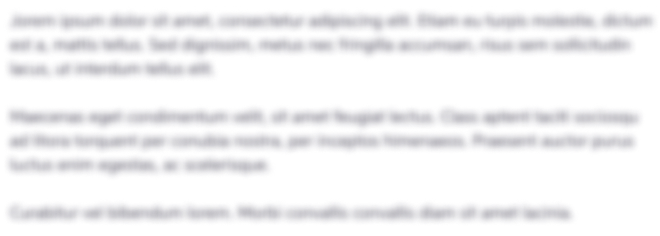
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started