Question
Hello, this assignment is based on this program, which is divided into two class files: https://pastebin.com/KwVg9G7C . Thank you for your help on this. Problem
Hello, this assignment is based on this program, which is divided into two class files: https://pastebin.com/KwVg9G7C . Thank you for your help on this.
Problem Summary
The home mortgage loan company that you wrote the Topic 11 program for would like you to expand the program to process a data file. They would like to have a program that will help them determine how much money they will earn in interest from a set of loans they have issued. Each line of the data file will contain a loan number, a loan amount, an annual interest rate, and a loan term.
Test Data Files
loanData1.txt
112233 200000 6.5 360 445566 155555 5.25 240
loanData2.txt
111111 111111 1.1 120 222222 222222 2.22 240 333333 300000 3.3 360 444444 340000 4.44 180 555555 350000 5 300
loanData3.txt
777777 700000 3.7 360 888888 180000 4 240 999999 999999 2.2 300
You will write a program to:
Read data from a file and determine the total interest paid on each loan. NOTE: You may assume all file data will be valid (no error checking necessary)
Provide a report on the loan company profits.
NOTE: Include file and method JavaDoc comments.
The program will contain two separate classes:
The first class will be the same ModLoan class you defined for Topic 11 part B
The second class will be the class that contains the main method
This class will be different from the Topic 11 main class, and is now called CalcInterestProfits
Delete all the NetBeans template code in this class, and replace it with the following template code to start:
import java.util.Scanner;
import java.io.*;
public class CalcInterestProfits {
public static void main(String[] args) throws IOException {
final String LOG_FILENAME = "log.txt";
Scanner keyboard = new Scanner(System.in);
String filename;
File inFile = null;
Scanner fileReader = null;
// Add additional variable declarations here
System.out.println("Enter input data filename:");
filename = keyboard.next(); //
// Insert more code here
}
}
Tests 1 & 2: Read data from one line of a data file, use it to instantiate an object, and display the data in the object
Within the CalcInterestProfits class: In the variables section of the template code: o Define a ModLoan reference variable and initialize it to null. o Define variables to hold the data read from the file.
After the template code that reads a filename from the user, open the input text data file as follows: Using the already defined File and Scanner variables from the template, add more code after the lines that read a filename from the user that will: o Instantiate a File object for the file whose name was input by the user. o Instantiate a Scanner object that can be used to read the file. NOTE: No exception handling will be implemented yet.
Read each of the 4 data items from the first line of the file only.
Using the defined ModLoan reference variable, instantiate a ModLoan object with the data read from the file line as the arguments to the constructor.
To confirm that the read worked correctly, call the displayLoanTerms() method with the ModLoan object to display the data from the line read: Loan 112233 is for $200000.00 at 6.50% APR, to be paid over 360 month(s)
Close the file.
Test 3 & 4: Read all of the lines in the data file and display them
Within the CalcInterestProfits class:
Add loop around the code that:
o Reads the data from one line of the file
o Instantiates a ModLoan object
o Calls displayLoanTerms() to display the data stored in the Loan object so that it reads and displays the data from all lines in the data file. NOTE: The loop should work no matter how many lines are in the file.
Test 5 & 6: Display a message with the number of lines read
Within the CalcInterestProfits class:
Add code to the loop keep track of how many lines are read
After all data in the file has been read and displayed, display another message, including the filename and number of lines read, as follows:
Done reading file loanData1.txt -- 2 lines of data read
Test 7 & 8: Compute monthly payments and interest paid for the loan object. Total the interest paid on all loan objects.
Within the CalcInterestProfits class:
Within the file reading loop, after the data in the Loan object is displayed:
o Call the computeMonthlyPayment()method with the object, and save the returned monthly payment for the loan.
o Call the computeTotalInterest()method with the object and save the returned interest for the loan
o Keep track of the total interest from all loans
After the loop, display a blank line, followed by the total interest, as follows:
Total interest to be collected on all loans: $ 351101.57
Test 9: Implement exception handling if the input file cannot be opened
Within the CalcInterestProfits class:
Remove the following clause from the main method header. throws IOException
The IOExceptions will now need to be handled
Implement file exception handling:
o Add a try block to determine if an IOException occurs when opening the data file.
o Add a catch block to handle IOException exceptions. If an exception occurs:
Display the system message stored in the exception object.
Then display: Program exiting.
And exit the program using the code: System.exit(1);
Tests 10 12: Create an output file, logging the interest paid on each loan
Within the CalcInterestProfits class,
Above the file reading loop (at the top of the main method):
o Declare a second File variable to be used with an output file.
o Declare a PrintWriter variable.
o Within a try block:
Using the File variable, instantiate a File object associated with LOG_FILENAME.
Using the PrintWriter variable, instantiate a PrintWriter object to be used for writing to an output file.
o Add a catch block to catch any IOException errors. If an IOException occurs:
Display the following message: Processing loan data without creating a log file
Within the file reading loop, at the end:
o Add code check if the output file was opened, and if it was:
to write the loan number and interest paid on the loan out to the log file, as follows:
xx.xx interest will be collected on loan number 112233
After the file reading loop and all other output displays:
o If the file was opened, close the file.
o Display the message: Loan interest details are located in file log.txt
Tests 13 & 14: Blind tests
Sample Run (when fully implemented)
Enter input data filename:
loanData1.txt
Loan 112233 is for $200000.00 at 6.50% APR, to be paid over 360 month(s)
Loan 445566 is for $155555.00 at 5.25% APR, to be paid over 240 month(s)
Done reading file loanData1.txt -- 2 lines of data read
Total interest to be collected on all loans: $ 351101.57
Loan interest details are located in file log.txt
Sample Log File
255088.98 interest will be collected on loan number 112233
96012.59 interest will be collected on loan number 445566
That's all. Please let me know if there is anything I need to clarify. Again, thank you!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
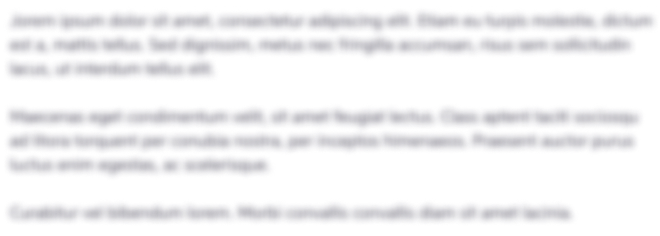
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started