Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Hello, this is for programming in C++ . Please follow the assignment below. I have several errors and I just dont know how to do
Hello, this is for programming in C++. Please follow the assignment below. I have several errors and I just dont know how to do this, please oh please help. I included the default programs that are included with this assignment at the bottom. Please follow the instructions. I really really need help, Thank you sooooo very much.
Objective Students will gain familiarity with using a user-created Stack class, exception handling, parsing algorithms, and working with postfix expressions. Assignment Summary Implement a simple stack-based calculator. It should work like dc. In Linur, dc is a reverse-polish (postfix) desk calculator. It reads from the standard input and sends output to the screen You are writing a client program whicl operations have already been implemented for you in stack.cpp. Exception handling should be provided for stack underflow, overflow, and any invalid operator which is entered by user h makes use of the stack operations in stack.h. These Name your client file dc.cpp and store it in a Prog2 directory of your class directory on your class account Calculator Operations Our version of dc will support integer arithmetic. To enter a negative number, begin with an u derscore (). The character cannot be used for this, as it is a binary operator for subtraction instead. To enter two numbers in succession, separate them with spaces or newlines. White space has no meaning as a command Your calculator should support the following operations p Prints the value on the top of the stack, without altering the stack. A newline is printed after the value n Prints the value on the top of the stack, pops it off, and does not print a newline after f Prints the entire contents of the stack without altering anything.A newline is printed after each value . c-Clears the stack, renderig t empty d Duplicates the value on the top of the stack, pushing another copy of i. Thus "4d*p" computes 4 squared and prints it r Reverses the order of (swaps) the top two values on the stack Pops two values off the stack, adds them, and pushes the result Pops two values, subtracts the first one popped from the second one popped, and pushes the result Pops two values, multiplies them, and pushes the result ./-Pops two values, divides the second one popped from the first one popped, and pushes the result % Pops two values, computes the remainder of the division that the / command would do. and pushes that Sample Run If the input is 2 3+ 4 6 P 4%p 2 100 3 4 5 f 5d*p rf2pc 



Below are the default programs given for the assignment. they are as follow and are separated by dash symbols:
stack.cpp, stack.h, and dsexceptions.h.
Please follown assignment... again thank you very verymuch
-------------------------------------------------------------------------------------------------------------------------------
//stack.cpp
/**
* Construct the stack.
*/
template
Stack
{
topOfStack = -1;
}
/**
* Test if the stack is logically empty.
* Return true if empty, false otherwise.
*/
template
bool Stack
{
return topOfStack == -1;
}
/**
* Test if the stack is logically full.
* Return true if full, false otherwise.
*/
template
bool Stack
{
return topOfStack == theArray.size( ) - 1;
}
/**
* Make the stack logically empty.
*/
template
void Stack
{
topOfStack = -1;
}
/**
* Get the most recently inserted item in the stack.
* Does not alter the stack.
* Return the most recently inserted item in the stack.
* Exception Underflow if stack is already empty.
*/
template
const Object & Stack
{
if( isEmpty( ) )
throw Underflow( );
return theArray[ topOfStack ];
}
/**
* Remove the most recently inserted item from the stack.
* Exception Underflow if stack is already empty.
*/
template
void Stack
{
if( isEmpty( ) )
throw Underflow( );
topOfStack--;
}
/**
* Insert x into the stack, if not already full.
* Exception Overflow if stack is already full.
*/
template
void Stack
{
if( isFull( ) )
throw Overflow( );
theArray[ ++topOfStack ] = x;
}
/**
* Return and remove most recently inserted item from the stack.
* Return most recently inserted item.
* Exception Underflow if stack is already empty.
*/
template
Object Stack
{
if( isEmpty( ) )
throw Underflow( );
return theArray[ topOfStack-- ];
}
--------------------------------------------------------------------------------------------------------------------------------------
//stack.h
#include "dsexceptions.h"
#include
using namespace std;
// Stack class -- array implementation
//
// CONSTRUCTION: with or without a capacity; default is 10
//
// ******************PUBLIC OPERATIONS*********************
// void push( x ) --> Insert x
// void pop( ) --> Remove most recently inserted item
// Object top( ) --> Return most recently inserted item
// Object topAndPop( ) --> Return and remove most recently inserted item
// bool isEmpty( ) --> Return true if empty; else false
// bool isFull( ) --> Return true if full; else false
// void makeEmpty( ) --> Remove all items
// ******************ERRORS********************************
// Overflow and Underflow thrown as needed
template
class Stack
{
public:
explicit Stack( int capacity = 10 );
bool isEmpty( ) const;
bool isFull( ) const;
const Object & top( ) const;
void makeEmpty( );
void pop( );
void push( const Object & x );
Object topAndPop( );
private:
vector
int topOfStack;
};
#include "stack.cpp"
------------------------------------------------------------------------------------------------------------------------------------------------
//dsexceptions.h
#ifndef _DSEXCEPTIONS_H_
#define _DSEXCEPTIONS_H_
class Underflow { };
class Overflow { };
class OutOfMemory { };
class BadIterator { };
class DataError { };
class DivisionByZero { };
#endif
-----------------------------------------------------------------------------------------------------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
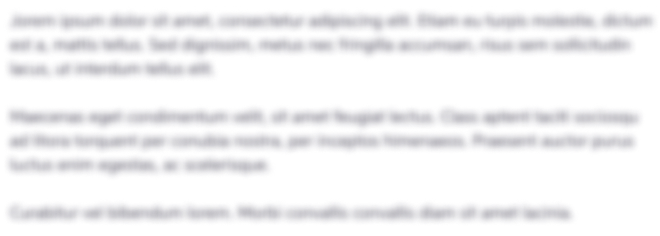
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started