Question
Hello this is my c# project, so if a want to build another form called cola type which when i click drink>>cola it shows types
Hello this is my c# project, so if a want to build another form called "cola type" which when i click drink>>cola it shows types of cola type button i wanted, now i can only do two layer of buttons and cant go futher more orwise i got error. i want three layers of buttons i want add a custom configuration Form that can click in and add items, such as toppings, can someone help me how to add another layer of button. following code is what i got so far.I can send you my database if you want
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms;
namespace DynFormEx { // All non-fixed data for Forms are read from the DB // frmMain is instantiated for all forms public partial class frmMain : Form { public bool DEBUG = true; // DEBUG mode for testing public Button btnOper; // Button on Form to close or perform other Ops public Label lblTitle; // Title of Form public Button[] btnArray; // Button array for Form private FormConfigArr frmCfgArr;// Array of configs for all Forms in App public int frmCfgArrIdx; // Index of current Form public string frmType; // Type of Form public string frmImage; // Path of background image on Form
// Form constructor for a typFrmButton Form private frmMain(int instNum, string frmType, string frmImage, FormConfigArr frmCfgArr) { this.frmCfgArrIdx = instNum; this.frmType = frmType; this.frmImage = frmImage; this.frmCfgArr = frmCfgArr; InitializeComponent(); }
// Form constructor for a typFrmImage Form private frmMain(int instNum, string frmType, FormConfigArr frmCfgArr) { this.frmCfgArrIdx = instNum; this.frmType = frmType; this.frmCfgArr = frmCfgArr; InitializeComponent(); }
// Form constructor for first Form - called from Program.cs public frmMain(int instNum, string frmType) { this.frmCfgArrIdx = instNum; this.frmType = frmType; InitializeComponent(); }
// btnOper event handler public void btnOper_Click(object sender, EventArgs e) { if (btnOper.Text == "Exit") { // Dispose deletes Form this.Dispose(); // Close would keep instance of Form // This may lead to multiple unneeded copies in memory //this.Close(); } else if (btnOper.Text == "Settings") { // Dispose deletes Form this.Dispose(); // Close would keep instance of Form // This may lead to multiple unneeded copies in memory //this.Close(); } }
// Form close for typFrmImage Forms public void frmMain_Click(object sender, EventArgs e) { // Dispose deletes Form this.Dispose(); // Close would keep instance of Form // This may lead to multiple unneeded copies in memory //this.Close(); }
// btnArray[] event handler public void btnArray_Click(object sender, EventArgs e) { // Cast sender as Button Button b = (Button)sender; // Cast Tag as ButtonConfig ButtonConfig bc = (ButtonConfig)b.Tag; // Declare reference to a frmMain Form frmMain nf = null; // Instantiate Form given type of Form if (bc.btnCfgTarget == "typFrmButton") nf = new frmMain(bc.frmOpenIdx, bc.btnCfgTarget, frmCfgArr); else if (bc.btnCfgTarget == "typFrmItem") nf = new frmMain(bc.frmOpenIdx, bc.btnCfgTarget, frmCfgArr); else if(bc.btnCfgTarget == "typFrmImage") nf = new frmMain(bc.frmOpenIdx, bc.btnCfgTarget, bc.btnCfgImage, frmCfgArr); // Show and transfer flow of contril to new Form nf.ShowDialog(); }
// Form load event handler - Set initial Form // and handle subsequent Forms private void frmMain_Load(object sender, EventArgs e) { // If initial Form if (frmCfgArrIdx == 0) { // Instantiate and fill frmCfgArr frmCfgArr = new FormConfigArr(); frmCfgArr.GetFormConfigs();
// Draw initial Form if(frmCfgArr != null && frmCfgArr.Count > 0 && frmCfgArr[0] != null) frmCfgArr[0].ConfigureButtonForm(this); } else // A subsequent Form { // If a Button Form if (frmType == "typFrmButton") { frmCfgArr[frmCfgArrIdx].ConfigureButtonForm(this); } // If a Image Form else if (frmType == "typFrmImage") { frmCfgArr[frmCfgArrIdx].ConfigureImageForm(this, frmImage); } } }
} }
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Drawing; using System.Windows.Forms;
namespace DynFormEx { // The FormConfig class contains data and methods to draw a Form class FormConfig { // Form data read from DB public int frmIdx; // The unique ID for the Form in app abd DB private string frmTitle; // The title shown on the Form (none for none) private string frmImage; // The path of the image to be displayed for background public string frmType; // The type of Form (typFrmButton or TypeFrmImage fir this app) private string imgDir; // The directory (folder) that contains the app images
// Button data public ButtonConfig[] btnConfig; // Array fo Buttons for this Form public int numButtons; // Number of Butons on Form public int maxBtnRows = 3; // Max rows of Buttons on Form public int maxBtnCols = 4; // Max cols of Buttons on Form
// Calculated measurements private int frmWidth; // Maximized width of Form private int frmHeight; // Maximized height of Form private int btnWidth; // Calculated width of a Button wrt the Form private int btnHeight; // Calculated height of a Button wrt the Form private int btnStartPosX; // Starting X position on Form of first Button private int btnStartPosY; // Starting Y position on Form of first Button private int btnOffsetX; // Spacing X between Buttons private int btnOffsetY; // Spacing Y between Buttons
// Constructor for FormConfig public FormConfig(int frmIdx, string frmTitle, string frmImage, string frmType, string imgDir) { this.frmIdx = frmIdx; this.frmTitle = frmTitle; this.frmImage = frmImage; this.frmType = frmType; this.imgDir = imgDir; }
// Draw a Form to expand an image public void ConfigureImageForm(frmMain fm, string path) { // Set Form Properties // Maximize the Form fm.WindowState = FormWindowState.Maximized; // Remove the Form control box fm.ControlBox = false; // Remove border around Form fm.FormBorderStyle = FormBorderStyle.None; // Read image from file Image image1 = Image.FromFile(path, true); // Set Image as background fm.BackgroundImage = image1; // Click event wiring fm.Click += new System.EventHandler(fm.frmMain_Click); // Stretch image to fit background size fm.BackgroundImageLayout = ImageLayout.Stretch; // Get width and height of maximized Form frmWidth = fm.Size.Width; frmHeight = fm.Size.Height; }
public void ConfigureButtonForm(frmMain fm) {
// Instantiate Form Button and Label fm.btnOper = new Button(); fm.lblTitle = new Label();
// Set Form Properties - These are fixed // Maximize the Form fm.WindowState = FormWindowState.Maximized; // Remove the Form control box fm.ControlBox = false; // Remove border around Form fm.FormBorderStyle = FormBorderStyle.None; string path = imgDir + frmImage; // Read image from file Image image1 = Image.FromFile(path, true); // Set Image as background fm.BackgroundImage = image1; // Stretch image to fit background size fm.BackgroundImageLayout = ImageLayout.Stretch; // Get width and height of maximized Form frmWidth = fm.Size.Width; frmHeight = fm.Size.Height;
// Get sizes and offsets - These are calculated from Form width and height btnWidth = (int)(frmWidth / 6.5); btnOffsetX = (int)(btnWidth * 0.5); btnStartPosX = btnOffsetX; btnHeight = (int)(frmHeight / 7.0); btnOffsetY = (int)(btnHeight * 1.75); btnStartPosY = btnOffsetY;
// Create exit Button if in DEBUG mode if (fm.DEBUG) { // Set Text property fm.btnOper.Text = "Exit"; // Set location of Button on Form fm.btnOper.Location = new System.Drawing.Point(frmWidth - 80, frmHeight - 30); // Event handler wiring fm.btnOper.Click += new System.EventHandler(fm.btnOper_Click); // Add Button to Form fm.Controls.Add(fm.btnOper); } // Else put Admin Button on Form else { // Set Text property fm.btnOper.Text = "Settings"; // Set location of Button on Form fm.btnOper.Location = new System.Drawing.Point(frmWidth - 80, frmHeight - 30); // Event handler wiring fm.btnOper.Click += new System.EventHandler(fm.btnOper_Click); // Add Button to Form fm.Controls.Add(fm.btnOper); }
// Put title Label on Form // Set position of Label // Note that (0, 0) is the upper left of Form int thisLblPosX = frmWidth / 2 - 250; int thisLblPosY = 75; // Set Label Name fm.lblTitle.Name = "lblTitle"; // Set Label Text fm.lblTitle.Text = frmTitle; // Set Label Font color fm.lblTitle.ForeColor = Color.AntiqueWhite; // Set Label background color fm.lblTitle.BackColor = Color.Black; // Set Label size fm.lblTitle.Size = new System.Drawing.Size(500, 50); // Set position of text in Label fm.lblTitle.TextAlign = ContentAlignment.MiddleCenter; // St Font family, point and style fm.lblTitle.Font = new Font("Arial", 24, FontStyle.Bold); // Set Label location fm.lblTitle.Location = new System.Drawing.Point(thisLblPosX, thisLblPosY); // Add Label to Form fm.Controls.Add(fm.lblTitle);
// Put Buttons on form // Init Button count int btnCount = 0; // Position of current Button on Form int thisBtnPosX = btnStartPosX; int thisBtnPosY = btnStartPosY; // Instantiate the array of Buttons for this Form fm.btnArray = new Button[maxBtnRows * maxBtnCols]; // Loop through rows of Buttons for (int i = 0; i
// ToString to print Form data public override string ToString() { string s = frmIdx + " "; s += frmTitle + " "; s += frmImage + " "; s += frmType + " "; s += imgDir + " "; s += numButtons; return s; }
} // End class
} // End namespace
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Data.OleDb; //using System.Windows.Forms; using System.IO;
namespace DynFormEx {
// Array of FormConfig for project class FormConfigArr : List
private static string imgDir;
// Constructor for FormConfigArr public FormConfigArr() { // Get Image dir from DB GetImgDir(); }
// Get Image dir from DB public static void GetImgDir() { // DB objects OleDbConnection conn = null; OleDbCommand comm = null; string sqlStr; // Query image dir from DB try { conn = GetConnection(); conn.Open(); sqlStr = "SELECT fldImgDir " + "FROM tblApplication " + "WHERE fldID = 0"; comm = new OleDbCommand(sqlStr, conn); OleDbDataReader reader = comm.ExecuteReader( System.Data.CommandBehavior.SingleRow); if (reader.Read()) { // Note that Access uses index of data given its // location in forst line of sqlStr imgDir = reader.GetString(0); } } catch (Exception ex) { //MessageBox.Show("Exception: " + ex.ToString()); } finally { if (conn != null) conn.Close(); } }
// Get Form config data from DB // This reads all the Form config data from DB public void GetFormConfigs() { // Number of Buttons on current Form int numButtons; // DB objects OleDbConnection conn = null; OleDbCommand comm = null; string sqlStr; // Read all Forms from DB try { conn = GetConnection(); conn.Open(); sqlStr = "SELECT fldID, fldFrmTitle, fldFrmImage, fldFrmType " + "FROM tblForms"; comm = new OleDbCommand(sqlStr, conn); OleDbDataReader reader = comm.ExecuteReader( System.Data.CommandBehavior.SingleResult); while (reader.Read()) { // Use FormConfig constructor to instantiate Form FormConfig cf = new FormConfig(reader.GetInt32(0), reader.GetString(1), reader.GetString(2), reader.GetString(3), imgDir); // Add form to this List this.Add(cf); } } catch (Exception ex) { //MessageBox.Show("Exception: " + ex.ToString()); } finally { if (conn != null) conn.Close(); }
// Add Bottons to Forms for (int i = 0; i
}
// Get a connection to DB public static OleDbConnection GetConnection() { OleDbConnection conn = null; string connStr = File.ReadAllText(@"..\..\DBConnStr.txt", Encoding.UTF8); conn = new OleDbConnection(connStr); return conn; }
} }
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Data.OleDb; using System.IO;
namespace DynFormEx { class BikesDB {
public static OleDbConnection GetConnection() { OleDbConnection conn = null; string connStr = File.ReadAllText(@"..\..\DBConnStr.txt", Encoding.UTF8); conn = new OleDbConnection(connStr); return conn; }
public static string[] getFormData() { string[] sArr = null; return sArr; }
} }
All Access Obje Search... Tables EEE tblApplication EEE tblButtons EEE tblForms EE tbl Forms EE tblApplication tblButtons fldFrmTitle fldID 0 Huang's pizzahut 1 pizzas 2 sandiwich 3 drink 4 cola type fldFrmlmag fldFrmType Click to Add wallpaper. jpg typFrmButton wallpaper. jpg typFrmButton wallpaper. jpg typFrmButton wallpaper. jpg typFrmButton wallpaper jpg typFrmButton
Step by Step Solution
There are 3 Steps involved in it
Step: 1
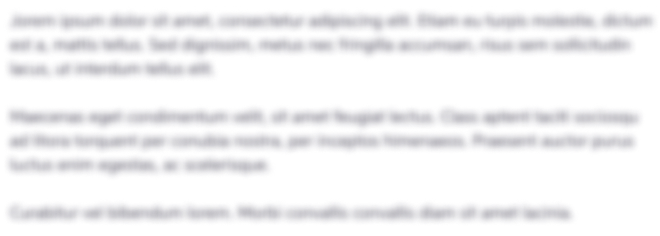
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started