Question
Hello, this question set is for C++ programming. Your help will be greatly appreciated, these questions are closely related to what will be on my
Hello, this question set is for C++ programming. Your help will be greatly appreciated, these questions are closely related to what will be on my final.
What is the correct way to call the accelerate(10) function on car1?
class Car
{
public:
Car(double speed);
void start();
void accelerate(double speed);
void stop();
double get_speed() const;
private:
double speed1;
};
Car::Car(double speed)
{
speed1 = speed;
}
void Car::start()
{
speed1 = 1;
}
void Car::accelerate(double speed)
{
speed1 = speed1 + speed;
}
void Car::stop()
{
speed1 = 0;
}
double Car::get_speed() const
{
return speed1;
}
int main()
{
Car* car1 = new Car(100);
// call the accelerate function on car1
return 0;
}
car1->accelerate(10); or *car1.accelerate(10); | ||
car1.accelerate(10); or *car1.accelerate(10); | ||
(*car1).accelerate(10); or car1.accelerate(10); | ||
(*car1).accelerate(10); or car1->accelerate(10); |
5 points
Question 2
Which option best describes this class definition, assuming the member functions are implemented correctly, but not shown below?
class Month
{
public:
int get_Month_num() const;
string get_Month() const;
Month(int);
private:
int mon;
};
void main()
{
Month m(3);
cout << Month << get_Month() << is number << m.mon << endl;
}
It does not have enough member functions. | ||
It needs more private data members. | ||
It has a syntax error. | ||
It prints out "Month March is number 3". |
5 points
Question 3
The technique of hand-tracing objects uses
I. Public member functions written on the front of an index card.
II. Private data members written on the back of an index card.
III. Constructors in a global master list for all defined objects.
IV. One unique index card for each instance of an object.
I, II, III | ||
I, III, IV | ||
II, III, IV | ||
I, II, IV |
5 points
Question 4
In an online billing system, SalesOrderItem must have a cost data member that cannot be less than zero. How can you ensure that this condition will be maintained?
Define a global variable to store the cost data and a function that checks that the cost is never less than zero. | ||
Define a SalesOrderItem class with a public cost data member and add a comment advising programmers that the cost cannot be less than zero. | ||
Define a SalesOrderItem class with a private cost data member and define public mutator functions that never let the cost value to be less than zero. | ||
All of these options can be used to ensure that the cost is less than zero. |
5 points
Question 5
In the given code snippet, what type of member function is view()?
class CashRegister
{
public:
void view() const;
private:
int item_count;
double total_price;
};
void CashRegister::view() const
{
cout << item_count << endl;
cout << total_price << endl;
}
Accessor member function | ||
Mutator member function | ||
Private member function | ||
Constructor |
5 points
Question 6
The Item class has a public function called display_item(). What is the correct way of calling the display_item() function in the main() function?
int main()
{
Item* item_ptr = new Item;
// Call the display_item function using item_ptr
return 0;
}
item_ptr.display_item(); | ||
*item_ptr.display_item(); | ||
item_ptr->display_item(); | ||
display_item(item_ptr); |
5 points
Question 7
To improve the design of a program, parallel vectors should be replaced with which of the following?
A single vector whose elements are objects containing the data in the parallel vectors | ||
Arrays that contain the same data as the parallel vectors | ||
A single array that contains the parallel vectors | ||
One vector that contains all of the original parallel vectors |
5 points
Question 8
Parallel vectors are vectors that
Never intersect | ||
Have the same length and have data elements at each slice that should be processed together | ||
Have the same length and contain exactly the same data elements | ||
Have the same overall dimension and are integer |
5 points
Question 9
In a car rental application, the following Car class interface is defined. One of the programmers discovers a bug in the void accelerate(double speed) function. She corrects the bug by modifying the code snippet in the implementation of the Car class accelerate function. What must be changed in the interface?
class Car
{
public:
void start();
void accelerate(double speed);
void stop();
double get_speed() const;
private:
double speed;
};
The accelerate(double speed) declaration must be updated. | ||
A new private acceleration data member must be added. | ||
The accelerate function must be declared as void accelerate(double acceleration). | ||
No change is required in the interface because only the implementation was modified. |
5 points
Question 10
Study the following class interface for the class AeroPlane:
class AeroPlane
{
public:
void set_new_height(double new_height);
void view() const;
void view_new_height() const;
AeroPlane();
AeroPlane(double new_height);
AeroPlane(double new_height, double new_speed);
AeroPlane(int new_height, int new_speed);
private:
double height;
double speed;
};
Which of the following constructors is called for the object declaration AeroPlane c1(10, 100)?
AeroPlane() | ||
AeroPlane(double new_height) | ||
AeroPlane(double new_height, double new_speed) | ||
AeroPlane(int new_height, int new_speed) |
5 points
Question 11
Does this CashRegister class support encapsulation?
class CashRegister
{
public:
double total_price;
void view_count() const;
private:
int item_count;
};
Yes, it supports encapsulation of its data members. | ||
No, it should not have a private data member. | ||
No, it should not have a public data member. | ||
No, it should have a private data function. |
5 points
Question 12
Which of the following code snippets is legal for implementing the Shopping_Cart class member function named add_product? Assume that the Shopping_Cart class contains data members product_count and total_price.
void Shopping_Cart::add_product(double price) { product_count = product_count + 1; total_price = total_price + price; } | ||
void add_product(Shopping_Cart shpcrt, double price) { shpcrt.product_count = shpcrt.product_count + 1; shpcrt.total_price = shpcrt.total_price + price; } | ||
void add_product(double price) { product_count = product_count + 1; total_price = total_price + price; } | ||
void Shopping_Cart_add_product(double price) { product_count = product_count + 1; total_price = total_price + price; }; |
5 points
Question 13
Examine the following code snippet.
class Product
{
public:
Product();
Product(double price);
Product(string d_description);
Product(string d_description, double price);
void set_price(double price);
void set_description(string d_description);
string get_description() const;
void display_product() const;
private:
string description;
double product_price;
};
class SalesOrder
{
public:
SalesOrder(string customer_name, string d_description,
double price);
void display_sales_order() const;
private:
Product prdt;
string customer;
};
class RetailShop
{
public:
RetailShop();
void set_sales_order(SalesOrder new_SalesOrder);
SalesOrder get_sales_order();
private:
SalesOrder sales_order;
};
Which of the following statements is correct?
The SalesOrder class aggregates the Product class. | ||
The Product class aggregates the RetailShop class. | ||
The Product class aggregates the SalesOrder class. | ||
The SalesOrder class aggregates the RetailShop class. |
5 points
Question 14
You are given the class definition for CashRegister. One of the member functions of this class is get_total(), which returns a double that represents the register total for the object. Furthermore, you have set up and allocated an array of CashRegisterobjects. Given the declaration of the array all_registers below, which code snippet correctly calls the get_total() function on every object in the all_registers array and uses it to calculate the sum of all registers (total of all individual register totals)?
CashRegister all_registers[20];
double result = 0.0; for (int i = 0; i < 20; i++) { result += all_registers[i].get_total(); } | ||
double result = 0.0; for (int i = 0; i < 20; i++) { result += all_registers[i]->get_total(); } | ||
double result = 0.0; for (int i = 0; i < 20; i++) { if (all_registers[i].get_total() > result) { result = all_registers[i]->get_total(); } } | ||
double result = 0.0; for (int i = 0; i < 20; i++) { if (all_registers[i].get_total() > result) { result = all_registers[i].get_total(); } } |
5 points
Question 15
For defining class member functions, a bank balance, cash register total, level of gas in a tank, or a students GPA would be examples of what common object data/function?
Book-keeping | ||
Calculations | ||
Keeping a total | ||
Collecting values |
5 points
Question 16
What is the output of the following code snippet?
class CashRegister
{
public:
void set_item_count(int count);
void view() const;
private:
int item_count;
};
void CashRegister::view() const
{
cout << item_count << endl;
}
void CashRegister::set_item_count(int count)
{
item_count = count;
}
int main()
{
CashRegister reg1, reg2;
reg1.set_item_count(15);
reg2.set_item_count(10);
reg1.view();
reg2.view();
return 0;
}
15 25 | ||
15 10 | ||
10 15 | ||
15 15 |
5 points
Question 17
The class Person is defined so that it contains a public data member answers. This data member is defined as:
vectoranswers;
Suppose client code has declared a variable of type Person named p1. Which code snippet correctly finds the number of times the integer 4 appears in the answers vector?
int n = 0; for (int i = 0; i < p1.answers.size(); i++) { if (p1.answers == 4) { n++ } } cout << "Answer is " << n << endl; | ||
int n = 0; for (int i = 0; i < p1.answers.size(); i++) { if (p1.answers[n] == 4) { n++ } } cout << "Answer is " << n << endl; | ||
int n = 0; for (int i = 0; i < p1.answers.size(); i++) { if (p1.answers[i] == 4) { n++ } } cout << "Answer is " << n << endl; | ||
int n = 0; for (int i = 0; i < p1.answers.size(); i++) { if (p1->answers == 4) { n++ } } cout << "Answer is " << n << endl; |
5 points
Question 18
What is the output of the following code snippet?
class CashRegister
{
public:
void set_item_count(int count);
private:
int item_count;
};
void CashRegister::set_item_count(int count)
{
item_count = count;
}
int main()
{
CashRegister reg1;
reg1.set_item_count(15);
cout << "Item count: " << reg1.item_count;
return 0;
}
Item count: | ||
Item count: 15 | ||
The code snippet does not compile. | ||
Item count: 0 |
5 points
Question 19
Study the following class interface for a class Car:
class Car
{
public:
void start();
void accelerate(double speed);
void stop();
double get_speed() const;
Car();
Car(double speed, bool condition);
Car(double speed, double distance, bool condition);
Car(double speed, double distance);
private:
double speed1;
double distance2;
};
Which constructor is called for the object declaration Car c1(42.0)?
Car() | ||
Car(double speed, double distance) | ||
accelerate(double speed) | ||
None of the listed items |
5 points
Question 20
When designing classes to solve a specific problem, one simple approach to discovering classes and member functions is to
Identify nouns and verbs, which correspond to classes and functions, respectively | ||
Identify nouns and verbs, which correspond to functions and classes, respectively | ||
Turn actions into classes | ||
Search for aggregation relationships, where classes share global data members |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
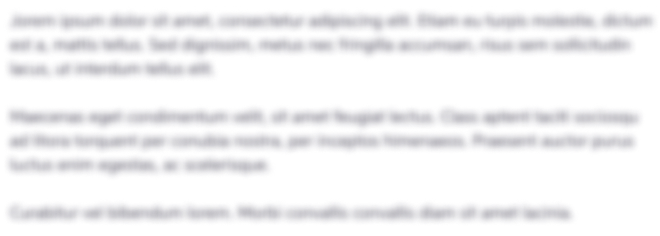
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started