Question
Hello. Would appreciate some help with this assignment. It's a Pod game in Java. The full assignment details are listed below, followed by the application
Hello. Would appreciate some help with this assignment. It's a "Pod" game in Java. The full assignment details are listed below, followed by the application code given to us and what I have so far for the Pod support class. Thank you in advance.
Introduction
This assignment is meant to introduce you to generalized container classes in Java.
In this assignment you will re-implement the pod chase game from Assignment 2, adding a PodList class that stores a list of Pod object, as well as rewriting the Pod class to better take advantage of container methods.
Game Structure and User Interface Class
The basic structure of the game and visual application is mostly the same as in Project 1, in which the player (marked #) moves around a 2D map (currently 15x9) to collect pods (marked *). The game provides buttons (N, S, E, and W) that moves the player one square in the direction north, south, east, or west respectively. The pods themselves are always in motion, moving is a diagonal direction (either NE, NW, SE, or SW). When one reaches the edge of the board, it bounces off of the wall, changing its direction. When the player reaches the current location of a pod, then that pod is considered caught and will no longer be displayed.
The main way that the game has changed is that every move there is a chance that a new pod will be generated. More specifically:
Each turn, a new pod will be generated with a 10% chance. This probability may be change in future versions of the game, so you will need to make it easy to change.
When a new pod is generated, it is placed at a random location on the board, and will be moving in a random direction (either NE, NW, SE, or SW). I have provided on the course web page a user interface class called PodApp. While you will not be changing any of the code in this class, I do encourage you to take a look at the code in order to better understand the requirements for the classes that you will be creating.
Requirements for the PodList class
As you can see from the PodApp code, it now stores a single support object called PodList, rather than an array of Pod objects.
This PodList class is to act as a container for a list of Pod objects. Its purpose is to simplify the code in the application. Rather that the application having to loop through the list of pods to do things, this will be the responsibility of the PodList.
Internal Structure
Your PodList class is required to store its Pod objects in an ArrayList. To simplify your code, I suggest that you use generics to make it an ArrayList of type Pod.
Required Methods and Constructors
Your PodList class is to have the following methods (which you can see in action in the PodApp application):
public PodList(int width, int height)
This constructor takes the width and height of the board as parameters (and should probably store them in member variables).
It should construct four Pod objects and add them to its ArrayList. These pods are to be initialized to the following locations and directions: x = 1, y = 5, direction = NE x = 2, y = 1, direction = SW x = 12, y = 2, direction = NW x = 13, y = 6, direction = SE
public void moveAll()
This method should loop through the ArrayList, calling the move method of each pod in the list. This method is called by the application each turn.
public void generate()
This method is called by the application each turn. When it is called, it should generate a new Pod object with a 10% chance, and add that pod to the end of the ArrayList.
public boolean isPod(int x, int y)
This method should return true if there is a Pod in the ArrayList at location (x, y), false otherwise. This method is called by the application during the process of redrawing the board.
public void playerAt(int x, int y)
This method is called every turn, passing the current (x, y) location of the player. If there is a pod at that (x, y) location, it is considered to be caught, and should be removed from the ArrayList.
Requirements for the Pod class
The Pod class will also be greatly simplified from the first assignment. You should be able to reuse most of the code you already have, and either delete or comment out the parts that are no longer necessary. Specifically, you should no longer need: isVisible: Since pods caught by the player are now removed from the list, there is no need to make them invisible when caught. playerAt: Determining whether a pod is caught is now handled by the playerAt method of the PodList. getX and getY: Previously, this was used by the app to draw the pod on the board, which is now handled by the isPod method of the PodList. The only code that you should need to add is the overloaded .equals method described below.
Your new Pod class should now have the following constructors and methods:
public Pod(int x, int y, String direction, int width, int height)
This constructor takes as its parameters: The initial x and y coordinates of the pod. The initial direction it is moving in. This will be a string with value either NE, NW, SE, or SW. The width and height of the game board (you will need to know this in order to bounce the pod off of the walls).
This constructor should pretty much be unchanged from your first assignment.
public void move() This method moves the pod in the direction of its current motion. Specifically: Going north (either NE or NW) increment the Y location by 1. Going south (either SE or SW) decrement the Y location by 1. Going east (either NE or SE) increment the X location by 1. Going west (either NW or SW) decrement the X location by 1.
As before, if the pod hits a wall (which can be computed from the parameters passed to the constructor), it should bounce off of the wall. More specifically: If it hits the top or bottom wall, the vertical direction changes. For example, if one hits the top wall going NE its direction changes to SE, and if it was going NW, its direction changes to SW. If it hits the left or right wall, the horizontal direction changes. For example, if one hits the right wall going NE its direction changes to NW, and if it was going SE, its direction changes to SW.
This method will probably also be pretty much unchanged from the first assignment.
public boolean equals(Object obj)
This method should return true if the object obj is equivalent to this pod. We will define equivalence as having the same location (that is, the same x and y coordinates).
As described in class, this will involve: Making sure that obj is a Pod, and then casting it to a Pod. Comparing the x and y coordinates of this pod to the x and y coordinates of the pod you have cast obj to.
Note that creating this equals method will make it much easier to implement the isPod and playerAt methods of the PodList class, as described in the next section.
Using Pod Equality to Implement PodList Methods
Note that the the isPod and playerAt methods of the PodList class both take an (x, y) location as parameters, and then determine whether there is a pod at that location (to display it or delete it respectively.
One way to implement this would be to manually search the ArrayList in the PodList for a pod with those coordinates. However, the existence of the equals method in the Pod class allows us to use the built-in search methods of the ArrayList instead.
Given that method, a very simple way to search for a pod with a given (x, y) location is to: Construct a Pod object with that a given (x, y) location (the direction, etc. is not relevant). Search for that Pod object in the ArrayList. This is to be done using the contains method. If that Pod object is found, either return true (for isPod) or remove that Pod object (for playerAt).
This will allow you to replace the manual search with a single method call!
Here's the application code given to us (doesn't need any changing):
/* * This class represents a visual application for the "pod chase" game. */ package project6; /** * * @author */ import javax.swing.*; import java.awt.*; import java.awt.event.*; public class PodApp extends JFrame implements ActionListener { // Member variables for visual objects. private JLabel[][] board; // 2D array of labels. Displays either # for player, // * for pod, or empty space private JButton northButton, // player presses to move up southButton, // player presses to move down eastButton, // player presses to move right westButton; // player presses to move left // Current width and height of board private int width = 15; private int height = 9; // Current location of player private int playerX = 7; private int playerY = 4; // PodList object private PodList pods; public PodApp() { // Construct a panel to put the board on and another for the buttons JPanel boardPanel = new JPanel(new GridLayout(height, width)); JPanel buttonPanel = new JPanel(new GridLayout(1, 4)); // Use a loop to construct the array of labels, adding each to the // board panel as it is constructed. Note that we create this in // "row major" fashion by making the y-coordinate the major // coordinate. We also make sure that increasing y means going "up" // by building the rows in revers order. board = new JLabel[height][width]; for (int y = height-1; y >= 0; y--) { for (int x = 0; x < width; x++) { // Construct a label to represent the tile at (x, y) board[y][x] = new JLabel(" ", JLabel.CENTER); // Add it to the 2D array of labels representing the visible board boardPanel.add(board[y][x]); } } // Construct the buttons, register to listen for their events, // and add them to the button panel northButton = new JButton("N"); southButton = new JButton("S"); eastButton = new JButton("E"); westButton = new JButton("W"); // Listen for events on each button northButton.addActionListener(this); southButton.addActionListener(this); eastButton.addActionListener(this); westButton.addActionListener(this); // Add each to the panel of buttons buttonPanel.add(northButton); buttonPanel.add(southButton); buttonPanel.add(eastButton); buttonPanel.add(westButton); // Add everything to a main panel attached to the content pane JPanel mainPanel = new JPanel(new BorderLayout()); getContentPane().add(mainPanel); mainPanel.add(boardPanel, BorderLayout.CENTER); mainPanel.add(buttonPanel, BorderLayout.SOUTH); // Size the app and make it visible setSize(300, 200); setVisible(true); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // Construct the PodList with 4 pods initially pods = new PodList(width, height); // Draw the initial board drawBoard(); } // Auxiliary method to display player and pods in labels. public void drawBoard() { // Determine what to display in each square for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { // Is the player at this (x, y) location? if (playerX == x && playerY == y) { board[y][x].setText("#"); } // Is there a pod at this (x, y) location? else if (pods.isPod(x, y)) { board[y][x].setText("*"); } // Otherwise, draw a space there. else { board[y][x].setText(" "); } } } } public void actionPerformed(ActionEvent e) { // Move the pods pods.moveAll(); // Determine which button was pressed, and move player in that // direction (making sure they don't leave the board). if (e.getSource() == southButton && playerY > 0) { playerY--; } if (e.getSource() == northButton && playerY < height-1) { playerY++; } if (e.getSource() == eastButton && playerX < width-1) { playerX++; } if (e.getSource() == westButton && playerX > 0) { playerX--; } // Notify the pod list about player location pods.playerAt(playerX, playerY); // Possibly generate another pod pods.generate(); // Redraw the board drawBoard(); } /** * @param args the command line arguments */ public static void main(String[] args) { PodApp a = new PodApp(); } }
And here's what I have so far for the Pod support class (needs some additions) public class Pod { //member variables for pod class int x; //x coordinate of pod int y; //y coordinate of pod String direction; //direction of the pod int width; //width of the gameboard int height; //height of the gameboard Boolean caught=false; //make sure the game starts with pod not caught //constructor for pod class, intializes the member variables public Pod(int x, int y, String direction, int width, int height){ this.x=x; this.y=y; this.direction = direction; this.width = width; this.height = height; } //if isCaught is true the player caught the pod public boolean isCaught(){ return caught; } //this method moves the pod in the direction of its current motion //if it hits a wall the pod bounces off in a new direction public void move(){ //instances where pod does not bounce off any walls if(direction.equals("SW") && y > 0 && x > 0){ x--; y--; } else if(direction.equals("NW") && y < height - 1 && x> 0){ x--; y++; } else if(direction.equals("NE") && y < height-1 && x< width-1){ x++; y++; } else if(direction.equals("SE") && y > 0 && x < width-1){ x++; y--; } //Instances where pod bounces off the top or bottom wall else if(direction.equals("SW") && x>0 && y==0){ x--; y++; direction = "NW"; } else if(direction.equals("NW") && x > 0 && y == height - 1){ x--; y--; direction = "SW"; } else if(direction.equals("NE") && x < width-1 && y == height - 1){ x++; y--; direction = "SE"; } else if(direction.equals("SE") && x < width - 1 && y == 0){ x++; y++; direction = "NE"; } //Instances where pod bounces off the left or right wall else if(direction.equals("SW") && x == 0 && y > 0){ x++; y--; direction = "SE"; } else if(direction.equals("NW") && x == 0 && y < height - 1){ x++; y++; direction = "NE"; } else if(direction.equals("NE") && x == width - 1 && y< height - 1){ x--; y++; direction = "NW"; } else if(direction.equals("SE") && x == width - 1 && y> 0){ x--; y--; direction = "SW"; } //Instances where pod hits a corner and bounces diagonally else if(direction.equals("SW") && x == 0 && y == 0){ x++; y++; direction = "NE"; } else if(direction.equals("NW") && x == 0 && y == height - 1){ x++; y--; direction = "SE"; } else if(direction.equals("NE") && x == width - 1 && y == height - 1){ x--; y--; direction = "SW"; } else if(direction.equals("SE") && x == width - 1 && y == 0){ x--; y++; direction = "NW"; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
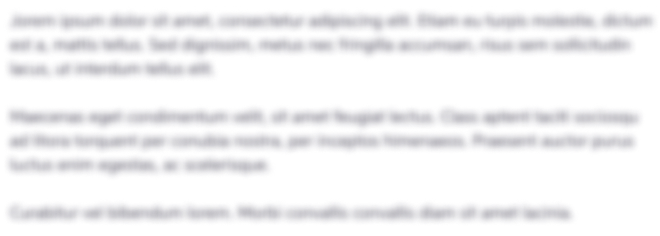
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started