Question
Help Bug Fixing my C++ Code Node.h #ifndef NODE_H #define NODE_H #include #include using namespace std ; template < class T > class node {
Help Bug Fixing my C++ Code
Node.h
#ifndef NODE_H
#define NODE_H
#include
#include
using namespace std;
template<class T>
class node {
public:
node();
node(T);
node(T, node<T> *);
node(const node<T>& orig);
~node();
void printAll();
node<T>* GetNextNode() const {
return nextNode;
}
void SetNextNode(node<T>* _nextNode) {
nextNode = _nextNode;
}
T GetValue() const {
return value;
}
void SetValue(T _value) {
value = _value;
}
private:
T value;
node<T> *nextNode;
};
#endif /* NODE_H */
Node.cpp
#include "node.h"
template<class T>
node<T>::node() : value(0), nextNode(NULL){
}
template<class T>
node<T>::node(T _value): value(_value), nextNode(NULL) {
}
template<class T>
node<T>::node(T _value, node *_nextNode) : value(_value), nextNode(_nextNode) {
}
template<class T>
node<T>::node(const node<T>& orig) : value(orig.GetValue()), nextNode(orig.GetNextNode()) {
}
template<class T>
node<T>::~node() {
}
template<class T>
void node<T>::printAll(){
cout << "Node content:" << endl;
cout << value << endl;
cout << nextNode << endl;
}
LinkedList.h
#ifndef LINKEDLIST_H
#define LINKEDLIST_H
#include "Node.h"
template
class LinkedList
{
int size;
node
node
public:
LinkedList();
~LinkedList();
int getSize() const;
node
node
void setSize(int s);
void setHead(node
void setTail(node
// insert the node n at t-th position(0...size)
void insertNode(int n, int pos);
// deletes the element at position pos from the list
void deleteNode(int pos);
//searches the node and returns its position
int search(T n);
void print();
};
#endif
LinkedList.cpp
#include "linkedlist.h"
#include
using namespace std;
template
LinkedList
{
size=0;
head=tail=NULL;
}
template
LinkedList
{
node
while(temp)
{
temp=head->GetNextNode();
delete head;
head=temp;
}
}
template
void LinkedList
{
cout << "List contents:" << endl;
node
while(temp)
{
temp->printAll();
}
}
template
int LinkedList
{
return size;
}
template
node
{
return head;
}
template
node
{
return tail;
}
template
void LinkedList
{
size=s;
}
template
void LinkedList
{
head=h;
}
template
void LinkedList
{
tail=t;
}
template
void LinkedList
{
node
if((pos==0) && (head==NULL))
{
head=tail=newnode;
}
else if(pos==0)
{
newnode->SetNextNode(head);
head=newnode;
}
else if(pos==size)
{
tail->GetNextNode()=newnode;
tail=newnode;
}
else
{
int i;
node
for(i=0;i { temp=temp->GetNextNode(); } newnode->GetNextNode()=temp->GetNextNode(); temp->GetNextNode()=newnode; } size++; } template void LinkedList { if(head == tail) { head=tail=NULL; } else if(pos==0) { head=head->GetNextNode(); } else { int i; node for(i=0;i { temp=temp->GetNextNode(); } temp->GetNextNode()=temp->GetNextNode()->GetNextNode(); size--; } } template int LinkedList { int pos=0,flag=0; node while(temp) { if(el==temp->GetValue()) { pos++; flag=1; } temp=temp->GetNextNode(); } if(flag) return pos; else return 0; } TestProgram.cpp #include "LinkedList.h" #include "Node.h" #include using namespace std; int main() { LinkedList int ch; char choice='y'; int el,pos; cout<<"This program performs operations on linked list"; do { cout<<" 1. Insert"; cout<<" 2. Delete"; cout<<" 3. Search"; cout<<" 4. Show all elements"; cout<<" 5. Exit"; cout<<" Enter your choice: "; cin>>ch; switch(ch) { case 1: cout<<"Enter a value to be inserted: "; cin>>el; cout<<"Enter a position where you want to enter an element: "; cin>>pos; if(pos<0 || pos>(list.getSize())) cout<<" Please enter a valid position (0-"< else { list.insertNode(pos-1,el); list.print(); } break; case 2: printf("Enter a position where you want to delete: "); cin>>pos; if(pos<0 || pos> (list.getSize()-1)) cout<<" Please enter a valid position(0-"< else { list.deleteNode(pos-1); list.print(); } break; case 3: if(list.getHead()==NULL) { cout<<"List is empty. "; } else { cout<<"Enter an element you want to search: "; cin>>el; pos=list.search(el); if(pos) cout<<"Element you want to serach is at position: %d ",pos; else cout<<" Element not found in the list. "; list.print(); } break; case 4: cout<<"Elements in the list are: "; list.print(); break; case 5: cout<<"Exiting..."; exit(0); break; default: cout<<"Invalid choice!"; break; } }while(choice=='y'||choice=='Y'); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
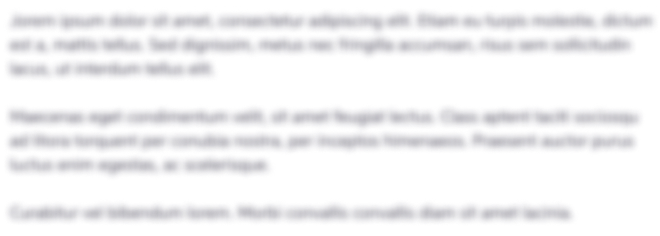
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started