Help creating these class in Java
Inputs are formated such as:
USA
20
30
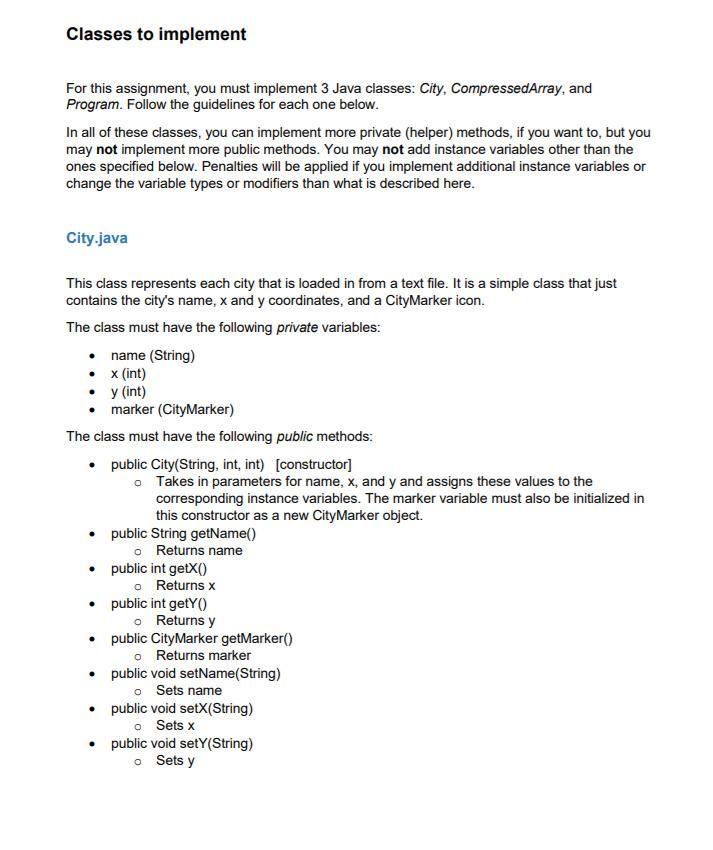
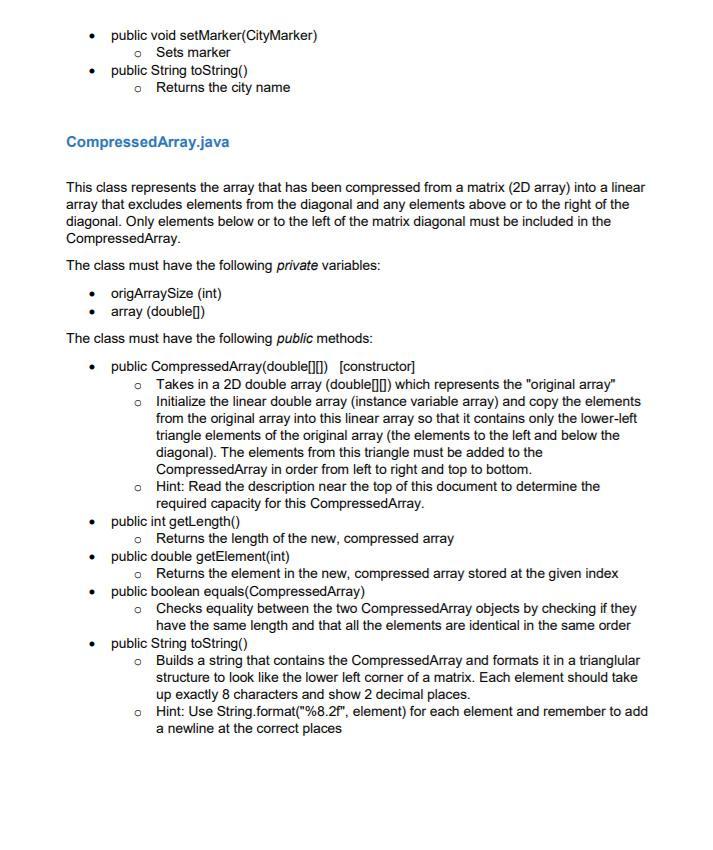
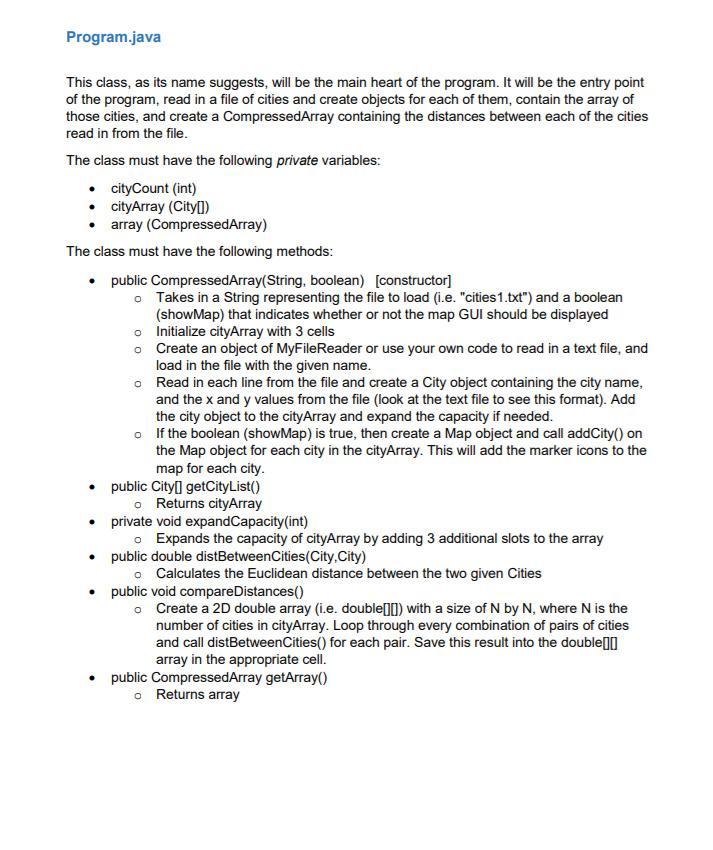
Classes to implement For this assignment, you must implement 3 Java classes: City, CompressedArray, and Program. Follow the guidelines for each one below. In all of these classes, you can implement more private (helper) methods, if you want to, but you may not implement more public methods. You may not add instance variables other than the ones specified below. Penalties will be applied if you implement additional instance variables or change the variable types or modifiers than what is described here. City.java This class represents each city that is loaded in from a text file. It is a simple class that just contains the city's name, x and y coordinates, and a CityMarker icon. The class must have the following private variables: name (String) x (int) y(int) marker (CityMarker) The class must have the following public methods: public City(String, int, int) [constructor] o Takes in parameters for name, x, and y and assigns these values to the corresponding instance variables. The marker variable must also be initialized in this constructor as a new City Marker object. public String getName() o Returns name public int getX() o Returns x public int getY0 o Returns y public City Marker getMarker() o Returns marker public void setName(String) o Sets name public void setX(String) o Sets x public void setY(String) o Sets y public void setMarker(CityMarker) o Sets marker public String toString() o Returns the city name CompressedArray.java This class represents the array that has been compressed from a matrix (2D array) into a linear array that excludes elements from the diagonal and any elements above or to the right of the diagonal. Only elements below or to the left of the matrix diagonal must be included in the CompressedArray. The class must have the following private variables: origArray Size (int) array (double() The class must have the following public methods: public CompressedArray(double[]0) [constructor] o Takes in a 2D double array (double(0) which represents the original array" o Initialize the linear double array (instance variable array) and copy the elements from the original array into this linear array so that it contains only the lower-left triangle elements of the original array (the elements to the left and below the diagonal). The elements from this triangle must be added to the CompressedArray in order from left to right and top to bottom. o Hint: Read the description near the top of this document to determine the required capacity for this CompressedArray. public int getLength() o Returns the length of the new, compressed array public double getElement(int) o Returns the element in the new, compressed array stored at the given index public boolean equals(CompressedArray) o Checks equality between the two CompressedArray objects by checking if they have the same length and that all the elements are identical in the same order public String toString() o Builds a string that contains the CompressedArray and formats it in a trianglular structure to look like the lower left corner of a matrix. Each element should take up exactly 8 characters and show 2 decimal places. o Hint: Use String.format("%8.2f", element) for each element and remember to add a newline at the correct places Program.java This class, as its name suggests, will be the main heart of the program. It will be the entry point of the program, read in a file of cities and create objects for each of them, contain the array of those cities, and create a CompressedArray containing the distances between each of the cities read in from the file. The class must have the following private variables: cityCount (int) cityArray (City) array (CompressedArray) The class must have the following methods: public CompressedArray(String, boolean) [constructor] o Takes in a String representing the file to load (i.e. "cities 1.txt") and a boolean (showMap) that indicates whether or not the map GUI should be displayed o Initialize cityArray with 3 cells o Create an object of MyFileReader or use your own code to read in a text file, and load in the file with the given name. o Read in each line from the file and create a City object containing the city name, and the x and y values from the file (look at the text file to see this format). Add the city object to the cityArray and expand the capacity if needed. o If the boolean (showMap) is true, then create a Map object and call addCity() on the Map object for each city in the cityArray. This will add the marker icons to the map for each city public City() getCityList() o Returns cityArray private void expandCapacity (int) o Expands the capacity of cityArray by adding 3 additional slots to the array public double distBetweenCities(City City) o Calculates the Euclidean distance between the two given Cities public void compare Distances() o Create a 2D double array (i.e. double(0) with a size of N by N, where N is the number of cities in cityArray. Loop through every combination of pairs of cities and call distBetweenCit ) for each pair. Save this result into the double | array in the appropriate cell. public CompressedArray getArray() o Returns array Classes to implement For this assignment, you must implement 3 Java classes: City, CompressedArray, and Program. Follow the guidelines for each one below. In all of these classes, you can implement more private (helper) methods, if you want to, but you may not implement more public methods. You may not add instance variables other than the ones specified below. Penalties will be applied if you implement additional instance variables or change the variable types or modifiers than what is described here. City.java This class represents each city that is loaded in from a text file. It is a simple class that just contains the city's name, x and y coordinates, and a CityMarker icon. The class must have the following private variables: name (String) x (int) y(int) marker (CityMarker) The class must have the following public methods: public City(String, int, int) [constructor] o Takes in parameters for name, x, and y and assigns these values to the corresponding instance variables. The marker variable must also be initialized in this constructor as a new City Marker object. public String getName() o Returns name public int getX() o Returns x public int getY0 o Returns y public City Marker getMarker() o Returns marker public void setName(String) o Sets name public void setX(String) o Sets x public void setY(String) o Sets y public void setMarker(CityMarker) o Sets marker public String toString() o Returns the city name CompressedArray.java This class represents the array that has been compressed from a matrix (2D array) into a linear array that excludes elements from the diagonal and any elements above or to the right of the diagonal. Only elements below or to the left of the matrix diagonal must be included in the CompressedArray. The class must have the following private variables: origArray Size (int) array (double() The class must have the following public methods: public CompressedArray(double[]0) [constructor] o Takes in a 2D double array (double(0) which represents the original array" o Initialize the linear double array (instance variable array) and copy the elements from the original array into this linear array so that it contains only the lower-left triangle elements of the original array (the elements to the left and below the diagonal). The elements from this triangle must be added to the CompressedArray in order from left to right and top to bottom. o Hint: Read the description near the top of this document to determine the required capacity for this CompressedArray. public int getLength() o Returns the length of the new, compressed array public double getElement(int) o Returns the element in the new, compressed array stored at the given index public boolean equals(CompressedArray) o Checks equality between the two CompressedArray objects by checking if they have the same length and that all the elements are identical in the same order public String toString() o Builds a string that contains the CompressedArray and formats it in a trianglular structure to look like the lower left corner of a matrix. Each element should take up exactly 8 characters and show 2 decimal places. o Hint: Use String.format("%8.2f", element) for each element and remember to add a newline at the correct places Program.java This class, as its name suggests, will be the main heart of the program. It will be the entry point of the program, read in a file of cities and create objects for each of them, contain the array of those cities, and create a CompressedArray containing the distances between each of the cities read in from the file. The class must have the following private variables: cityCount (int) cityArray (City) array (CompressedArray) The class must have the following methods: public CompressedArray(String, boolean) [constructor] o Takes in a String representing the file to load (i.e. "cities 1.txt") and a boolean (showMap) that indicates whether or not the map GUI should be displayed o Initialize cityArray with 3 cells o Create an object of MyFileReader or use your own code to read in a text file, and load in the file with the given name. o Read in each line from the file and create a City object containing the city name, and the x and y values from the file (look at the text file to see this format). Add the city object to the cityArray and expand the capacity if needed. o If the boolean (showMap) is true, then create a Map object and call addCity() on the Map object for each city in the cityArray. This will add the marker icons to the map for each city public City() getCityList() o Returns cityArray private void expandCapacity (int) o Expands the capacity of cityArray by adding 3 additional slots to the array public double distBetweenCities(City City) o Calculates the Euclidean distance between the two given Cities public void compare Distances() o Create a 2D double array (i.e. double(0) with a size of N by N, where N is the number of cities in cityArray. Loop through every combination of pairs of cities and call distBetweenCit ) for each pair. Save this result into the double | array in the appropriate cell. public CompressedArray getArray() o Returns array