Question
Help, I am having issues removing the name in my remove method public static void Remove (String n) in java.. I can add just
Help, I am having issues removing the name in my remove method "public static void Remove (String n) " in java.. I can add just fine but when i go to the remove method i get an error.
- - - -
public class LinkedQueue
{
private class Node
{
String value;
Node next;
Node(String val, Node n)
{
value = val;
next = n;
}
}
private Node front = null;
private Node rear = null;
/** Enqueue Employee **/
public void enqueue(String s)
{
if(rear != null)
{
rear.next = new Node(s,null);
rear = rear.next;
}
else
{
rear = new Node(s,null);
front = rear;
}
}
public boolean empty()
{
return front == null;
}
/** Return the Employee in the front **/
public String peek()
{
if(empty())
throw new EmptyQueueException();
else
return front.value;
}
/** Dequeue Employee **/
public String dequeue()
{
if(empty())
throw new EmptyQueueException();
else
{
String value = front.value;
front = front.next;
if(front==null) rear = null;
return value;
}
}
public String toString()
{
StringBuilder sBuilder = new StringBuilder();
Node p = front;
while (p != null)
{
sBuilder.append(p.value + " ");
p = p.next;
}
return sBuilder.toString();
}
public void add(String employee) {
// TODO Auto-generated method stub
}
}
- - - - - -
public class EmptyQueueException extends RuntimeException {
}
- - - - - - -
import java.util.LinkedList;
import java.util.Scanner;
public class Main
{
private static LinkedList
public static void main(String[] args)
{
Scanner scan = new Scanner(System.in);
System.out.println("1. Add");
System.out.println("2. Remove");
System.out.println("0. Quit");
String choice = scan.nextLine();
String name = null;
while (true) {
switch (choice) {
case "":
showMainMenu();
break;
case "0":
System.exit(0);
break;
case "1":
Add(name);
break;
case "2":
Remove(name);
break;
}
}
}
public static void showMainMenu() {
Scanner scan = new Scanner(System.in);
System.out.println("1. Add ");
System.out.println("2. Remove ");
System.out.println("0. Quit");
String choice = scan.nextLine();
while (true) {
switch (choice) {
case "":
showMainMenu();
break;
case "0":
System.exit(0);
break;
case "1":
Add(choice);
break;
case "2":
Remove(choice);
break;
}
}
}
public static void Add (String n)
{
String name;
Scanner scan = new Scanner(System.in); // creates scanner
LinkedQueue queue = new LinkedQueue(); // declares linkedlist
/** Add names **/
System.out.println("Add a name:");
name = scan.next();
queue.add(name);
nameList.add(name);
name = scan.next();
queue.add(name);
nameList.add(name);
name = scan.next();
queue.add(name);
nameList.add(name);
System.out.println("Adding names:");
for (String s : nameList)
{
System.out.print(s + " ");
queue.enqueue(s);
}
System.out.println(" State of queue is: ");
System.out.println(queue);
showMainMenu();
}
public static void Remove (String n)
{
/** Remove names **/
LinkedQueue queue = new LinkedQueue(); // declares linkedlist
System.out.println("Removing 2 names.");
queue.dequeue();
queue.dequeue();
System.out.println("State of queue is: ");
System.out.println(queue);
showMainMenu();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
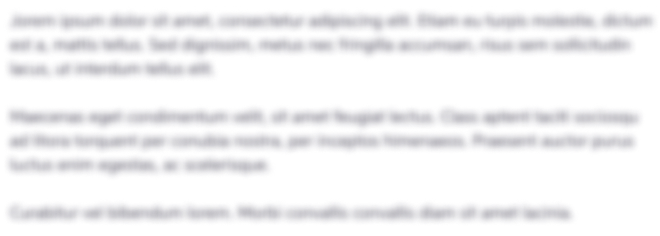
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started