Question
HELP IN JAVA PLEASE !!!!! 1 Whats my GPA? For the first part of this lab, youre going to be writing a Java program that
HELP IN JAVA PLEASE !!!!!
1 Whats my GPA?
For the first part of this lab, youre going to be writing a Java program that computes GPA based on input from a user. Specifically, wed like a user to be able to enter input like the example below, which consists of pairs of letter grades and course credit hours:
a 4 b 4 a 2 b- 4
and your program should return a response in the format below, which reflects the weighted GPA:
The GPA is: 3.33 To help you get started, lets briefly review the Java built-in Scanner class we discussed in lecture.
Working with Scanner
First off, create a file named GPA.java and add the appropriate class syntax. Next, youll need to import the Scanner class. This is what allows you to use the class in your program. To do this, at the very top of your file, even above your class definition, you should add this line:
import java.util.Scanner;
1
CSCI 1933 LAB 2 1. WHATS MY GPA?
This tells your computer to add the Scanner class to the resources available in your program. Then, you can use it in your program. Inside your main method, add this basic code to test out how the Scanner class works:
System.out.println("Type something, then press enter"); Scanner myScanner = new Scanner(System.in); String input = myScanner.nextLine(); Donotcopyandpastecode} System.out.println("You input " + input);
This might not make a lot of sense, initially, so lets go through the two new lines. The first new line is the instantiation of our Scanner class.
Scanner myScanner = new Scanner(System.in);
Thats standard for an instantiation. You declare your variable to be a Scanner, then, using the new keyword, you create a new Scanner object. The System.in argument is telling your new Scanner object to read from the users input. Remember from lecture that the Scanner class also knows how to interact with other types of objects with input data (e.g. File objects).
String input = myScanner.nextLine();
This is where you finally get user input. The program will wait on the nextLine() method until something is entered as input, at which point the Scanner will return the input as a String. Note that the nextLine() method will always return a String.
The Scanner class can also be used to parse individual elements of a string. Update the code in your main method to the following and run it:
System.out.println("Type a sentence, then press enter"); Scanner myScanner = new Scanner(System.in);
String input = myScanner.nextLine(); Scanner stringScanner = new Scanner(input); int count = 0;
while(stringScanner.hasNext()) { String inputText = stringScanner.next(); System.out.println("Input " + count + ":" + inputText); count++;
}
Note that in this case, youve taken a single line of input text from the user and used the Scanner class to break it up into individual elements (called tokens), separating it at the whitespace characters. Here, you used the next() method, which will always return a String for each token, but there are other methods you can use if you expect other types of input data, such as nextInt() or nextDouble().
2
CSCI 1933 LAB 2 1. WHATS MY GPA?
Calculating GPA
Ok, now youre ready to write a GPA calculator! Students and advisors commonly need to find the GPA for specific groups of courses taken. Examples include finding the technical GPA or the GPA for prerequisite courses for a specific major.
Your Java program should take in letter grades and credit hour pairs from the command line and compute the GPA. In your main method, you need to prompt the user for their input, parse their input, and calculate their GPA.
Use the following chart for grade point values:
Grade A A- B+ B B- C+ C C- D+ D F
Grade Points 4.0 3.667 3.333 3.0 2.667 2.333 2.0 1.667 1.333 1.0 0.0
You can assume that credit hours are always whole integers.
Some examples of how your program should run follow:
> java GPA > Enter grades: a 4 b 4 a 2 b- 4 > The GPA is: 3.33
> java GPA > Enter grades: a4 > The GPA is: 4
> java GPA > Enter grades:
Hint: Writing a helper method to convert from letter to numeric grades may be helpful (this can be called from your main method).
3
CSCI 1933 LAB 2
2. WRITE A RATIONAL NUMBER CLASS
c- 4 b+ 4 a 3 > The GPA is: 2.91
> java GPA > Enter grades: c 4 d 1 a 3 b+ 4 a 4 > The GPA is: 3.145
To calculate GPA, use the following method: (gradei crediti)
i
gpa =
crediti i
For example, if you enter java GPA a 4 b- 3 c+ 2 your calculation would be: gpa = (4.04)+(2.6673)+(2.3332) = 3.185
9
If the user enters input in an incorrect format or using an incorrect grade symbol, your code should exit with the following error message: Error: Incorrect input format!
> java GPA > Enter grades: c4dd2 > Error: Incorrect input format!
> java GPA > Enter grades: g4a3b2 > Error: Incorrect input format!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
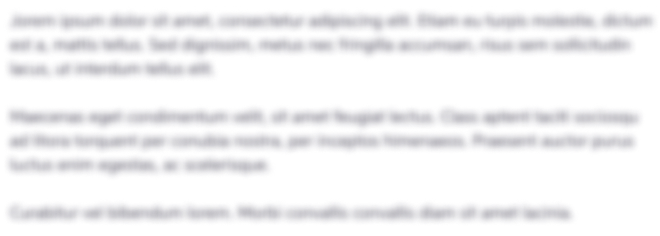
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started