Question
Help in JAVA: Use the Node class and the Circular Queue class you created. In your main program input the words from words.txt into a
Help in JAVA:
Use the Node class and the Circular Queue class you created. In your main program input the words from words.txt into a Queue (a circular queue) - do this by using enqueue .Then input an integer (n). Then starting at the head of the queue delete every nth node until only one node remains. Output the String in that last node. Make NO changes to the Node class. In the Queue class you will need a method to delete every nth node. You will need to print your queue, so make a print method in your Queue class. This should print the ENTIRE queue, not just the head. It is true that at the end only the head should remain, but by printing the entire queue and finding there is only one node printed you are verifying to me (and to yourself) that only the head remains.
Everything I have:
public static void main(String[] args) { //file input File inFile = new File("words.txt"); // try/catch Scanner fileInput = null; try { fileInput = new Scanner(inFile); } catch (FileNotFoundException ex) { }
//queue Queue q = new Queue(); //node head Node head = null; //string //String words=""; //file input while (fileInput.hasNextLine()) { String words = fileInput.next(); q.enqueue(words); //System.out.println(words); } int num = fileInput.nextInt();
q.delete(num, q); q.print(q.size()); }
}
public class Queue
private Node head, tail = null; int size = 0;
public Queue() { head = tail = null; size = 0;
}
//enqueue public void enqueue(T item) { Node temp = new Node(item); if (head == null) { head = temp; tail = temp; } else { temp.next = head; tail.next = temp; tail = temp;
} size++;
}
//dequeue public T dequeue(T item) { Node temp = head; head = head.next; tail.next = head; size--; return (T) temp.item; }
//size public int size() { if (size == 0) { } return size; }
//peek public T peek() { return (T) head.item; //return item }
//isEmpty public boolean isEmpty() {
return (size == 0); }
//print in queue class public void print(int i) { Node curr = head; while (curr != null) { System.out.println(curr.item); curr = curr.next; }
}
public void delete(int num, Queue q) { Node prev = null; int count=0; if (num > count || isEmpty()) { prev = head; } int deleteIndex = 1;
if (num == deleteIndex) { if (head.next == null) { tail = null; } head = head.next; count--; return; }
for (Node n = head; n != null; n = n.next, deleteIndex++) { if (count == deleteIndex) { prev.next = n.next; if (prev.next == null) { tail = prev; count--; return; } prev = n; } } } }
//node class public class Node {
Object item; Node next;
Node(Object newItem) { item = newItem; next = null; }
Node(Object newItem, Node nextNode) { item = newItem; next = nextNode; } }
PLEASE HELP!!!!!!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
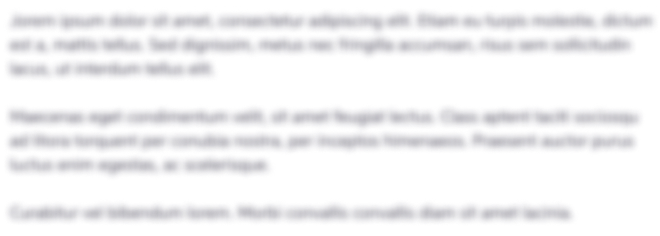
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started