Answered step by step
Verified Expert Solution
Question
1 Approved Answer
HELP ME FIX THE ERRORS PLEASE package lab 1 ; / * * * The counter class implements a counter that will roll over to
HELP ME FIX THE ERRORS PLEASE
package lab;
The counter class implements a counter that will roll over to the initial
value when it hits the maximum value.
@author Charles Hoot
@version
public class Counter
private int minimum;
private int maximum;
private int currValue;
PUT PRIVATE DATA FIELDS HERE
The default constructor for objects of class Counter. Minimum is and the maximum
is the largest possible integer.
public Counter
this.minimum ;
this.maximum Integer.MAXVALUE;
this.currValue minimum;
ADD CODE FOR THE CONSTRUCTOR
The alternate constructor for objects of class Counter. The minimum and maximum values are given as parameters.
The counter starts at the minimum value.
@param min The minimum value that the counter can have
@param max The maximum value that the counter can have
public Counterint min, int max
this.minimum min;
this.maximum max;
this.currValue minimum;
ADD CODE FOR THE ALTERNATE CONSTRUCTOR
Determine if two counters are in the same state
@param otherObject the object to test against for equality
@return true if the objects are in the same state
public boolean equalsObject otherObject
boolean result true;
if otherObject instanceof Counter
Counter othercounter Counter otherObject;
result this.currValue othercounter.currValue;
YOUR CODE GOES HERE
return result;
Increases the counter by one
public void increase
ifcurrValue maximum
currValue;
else
currValue minimum;
ADD CODE TO INCREASE THE VALUE OF THE COUNTER
Decreases the counter by one
public void decrease
ifcurrValue minimum
currValue; ADD CODE TO INCREASE THE VALUE OF THE COUNTER
else
currValue maximum;
Get the value of the counter
@return the current value of the counter
public int value
CHANGE THE RETURN TO GIVE THE CURRENT VALUE OF THE COUNTER
return currValue;
Accessor that allows the client to determine if the counter
rolled over on the last count
@return true if the counter rolled over
public boolean rolledOver
CHANGE THE RETURN TO THE ROLLOVER STATUS OF THE COUNTER
return currValue minimum;
Override the toString method to provide a more informative
description of the counter
@return a descriptive string about the object
public String toString
CHANGE THE RETURN TO A DESCRIPTION OF THE COUNTER
return "Counter" currValue Min: minimum Max: maximum ;
TESTING the constructor
Trying min max
Passes
Trying minmax
Fails no exception thrown
Trying min max
Fails no exception thrown
Finished constructor testing
TESTING the toString method
Displaying the counter using tostring:
CounterMin: Max:
The counter should have the value
the minimum should be the maximum should be
it should not have rolled over
Finished tostring testing
TESTING the equals method
trying two counters that should be in the same state
passes
trying two counters that should be in a different state
passes
trying two counters that should be in a different state
fails
Finished equals testing
TESTING the increase method
Increasing counter once
passes
passes
Increasing counter again
passes
passes
Increasing counter a third time
passes
passes
Increasing counter until it rolls over
passes
fails wrong number of increases; count was
Increasing counter until it rolls over
passes
fails wrong number of increases; count was
Finished increase testing
TESTING the decrase method
Decreasing the counter once
passes
fails should roll over
Decreasing the counter again
passes
fails should not roll over
Decreasing the counter a third time
passes
fails should roll over
Decrease counter twice. then decrease counter until it rolls over again
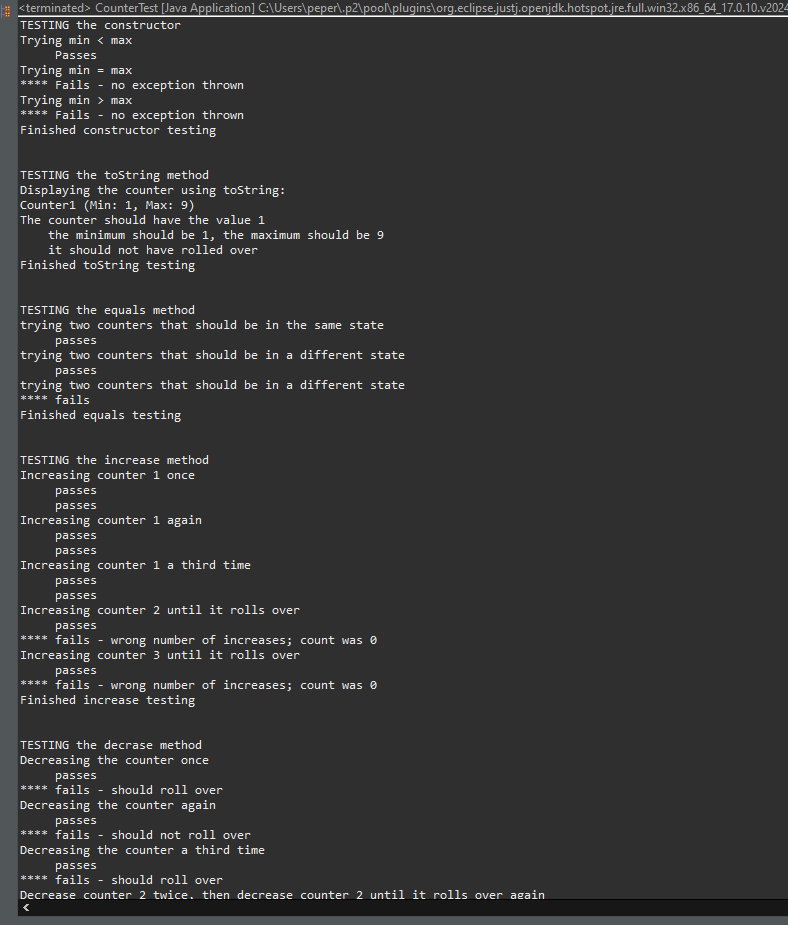
Step by Step Solution
There are 3 Steps involved in it
Step: 1
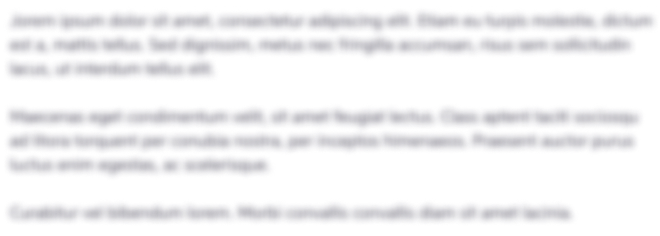
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started