Question
help me to write programm that use class inherits Polymorphism, and Virtual Functions from Design a ProgrammingProject class that is derived from the GradedActivity class
help me to write programm that use class inherits Polymorphism, and Virtual Functions from
Design a ProgrammingProject class that is derived from the GradedActivity class presented in your book. The ProgrammingProject class should determine the grade a student receives on a programming project. The student's total scores can be up to 100, and is determined in the following manner:
Use of classes: 15 points
Code Execution: 35 points
Proper Choice of Code: 21 points
Correct Pseudocode: 10 points
Use of comments: 11 points
Input/Output/professionalism: 8 points
Your ProgrammingProject class should define these point categories as member variables. After entering the scores, a total should be returned, as well as the letter grade, which is obtained from the parent class, GradedActivity.
Make sure that your member data is protected (with low level validation that creates an Exit_Failure when necessary). Validate user's input (high level validation) for all scores >=0 so that your program doesn't cause an Exit_Failure down in the object.
Create a user-interface program to demonstrate your class. Make your input and output professional. Break your code down into functions as necessary.
Programming Notes:
The ProgrammingProject class is based on (inherits from) the GradedActivity.
The ProgrammingProject class will contain members for the scores and the associated mutator/accessor functions, BUT it will call the setScore function and the getLetterGrade function from GradedActivity.
The main program will prompt and input and do high-level validation and store the results and then create the output display.
This isn't a particularly taxing assignment, so spend some time really doing nice work. Show me how you can shine!
Submit to me: main .cpp and .h and all files
I need something like that one this example of :
Contents of GradedActivity.h (Version 3) 1 #ifndef GRADEDACTIVITY_H 2 #define GRADEDACTIVITY_H 3 4 // GradedActivity class declaration 5 6 class GradedActivity 7 { 8 protected: 9 double score; // To hold the numeric score 10 public: 11 // Default constructor 12 GradedActivity() 13 { score = 0.0; } 14 15 // Constructor 16 GradedActivity(double s) 17 { score = s; } 18 19 // Mutator function 20 void setScore(double s) 21 { score = s; } 22 23 // Accessor functions 24 double getScore() const 25 { return score; } 26 27 virtual char getLetterGrade() const; 28 }; 29 #endif
Contents of PassFailActivity.h 1 #ifndef PASSFAILACTIVITY_H 2 #define PASSFAILACTIVITY_H 3 #include "GradedActivity.h" 4 5 class PassFailActivity : public GradedActivity
6 { 7 protected: 8 double minPassingScore; // Minimum passing score 9 public: 10 // Default constructor 11 PassFailActivity() : GradedActivity() 12 { minPassingScore = 0.0; } 13 14 // Constructor 15 PassFailActivity(double mps) : GradedActivity() 16 { minPassingScore = mps; } 17 18 // Mutator 19 void setMinPassingScore(double mps) 20 { minPassingScore = mps; } 21 22 // Accessors 23 double getMinPassingScore() const 24 { return minPassingScore; } 25 26 virtual char getLetterGrade() const; 27 }; 28 #endif
#include #include #include "PassFailActivity.h" using namespace std; // Function prototype void displayGrade(const GradedActivity &); int main() { // Create a PassFailActivity object. Minimum passing // score is 70. PassFailActivity test(70); // Set the score to 72. test.setScore(72); // Display the object's grade data. The letter grade // should be 'P'. What will be displayed? displayGrade(test); return 0; }
//*************************************************************** // The displayGrade function displays a GradedActivity object's * // numeric score and letter grade. * //*************************************************************** void displayGrade(const GradedActivity &activity) { cout << setprecision(1) << fixed; cout << "The activity's numeric score is " << activity.getScore() << endl; cout << "The activity's letter grade is " << activity.getLetterGrade() << endl; }
//*************************************************************** // The displayGrade function displays a GradedActivity object's * // numeric score and letter grade. * //*************************************************************** void displayGrade(const GradedActivity &activity) { cout << setprecision(1) << fixed; cout << "The activity's numeric score is " << activity.getScore() << endl; cout << "The activity's letter grade is " << activity.getLetterGrade() << endl; }
displayGrade(test2); // PassFailExam object return 0; } //*************************************************************** // The displayGrade function displays a GradedActivity object's * // numeric score and letter grade. * //*************************************************************** void displayGrade(const GradedActivity &activity) { cout << setprecision(1) << fixed; cout << "The activity's numeric score is " << activity.getScore() << endl; cout << "The activity's letter grade is " << activity.getLetterGrade() << endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
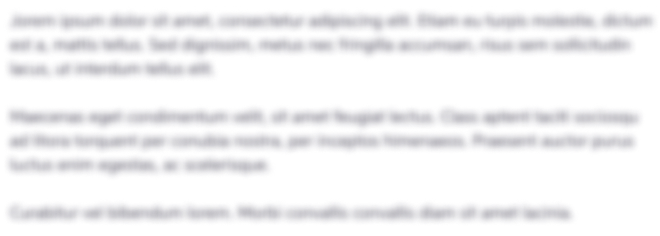
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started