Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Help needed: University of Washington Bothell CSS 5 0 3 : System Programming Program 3 C + + Standard I / O Library 1 .
Help needed: University of Washington Bothell CSS: System Programming Program C Standard IO Library Purpose In this programming assignment you will design and implement your own core input and output functions of the CC standard IO library: stdio.h Linux IO Unix provide system calls for file IO such as open read write and lseek However, these system calls are not supported on nonUnix based systems such as Window. Therefore, the CC standard IO library was created so that applications which require these functions can be easily ported through recompilation across these systems. CC Standard IO Library Overview The standard IO library is an architecture independent library that allows CC programs to read and write files instead of directly calling the underlying OS system calls. The library's functions add buffering and provide a userfriendly file stream interface FILE The file stream interface is implemented by the standard IO library utilizing the underlying system calls. When reading andor writing small bytecounts eg reading one line at a time from a file buffered functions are faster. The read and write system calls operate on file descriptors and readwrite fromto buffers not strings. A file descriptor is just an integer referring to a currently open file. The OS uses that number as an index into the file descriptor table of files currently in use to access the actual device eg disk, network, terminal On the other hand, file streams operators freadfwrite interact directly with file streams: FILE File streams are dynamically allocated and allow readingwriting raw data. File stream operators, fread and fwrite use the type void since there are no dataspecific requirements. The core input and output functions defined in include: Function name Description fopen opens a file fflush synchronizes an output stream with the actual file setbuf, setvbuf sets the size of an inputoutput stream buffer fpurge clears an inputoutput stream buffer fread reads from a file fwrite writes to a file fgetc reads a character from a file stream fputc writes a character to a file stream fgets reads a character string from a file stream fputs writes a character string to a file stream fseek moves the file position to a specific location in a file feof checks for the endoffile fclose closes a file printf prints formatted output to stdout Figure below shows how the standard IO library utilizes a buffer to reduce the number of read and write system calls with filestreams. Figure This figure shows Standard IO functions using FILE instead of file descriptor. FILE Data Structure and fopen Upon a file open, fopen returns a pointer to a FILE object that maintains the attributes of the opened file. On canvas is posted a version of the header file we will use for the project. The following shows the class FILE definition: #ifndef MYSTDIOH #define MYSTDIOH #define BUFSIZ default buffer size #define IONBF unbuffered #define IOLBF line buffered. Do not need to implement this mode. #define IOFBF fully buffered #define EOF end of file class FILE public: FILE : fd pos bufferchar size actualsize modeIONBF flag bufownfalse lastop eoffalse int fd; a Unix file descriptor of an opened file int pos; the current file position in the buffer char buffer; an input or output file stream buffer int size; the buffer size int actualsize; actual buffer size when read returns # bytes smaller than size int mode; IONBF, IOLBF, IOFBF. You do not need to implement IOLBF. int flag; ORDONLY ORDWR OWRONLY OCREAT OTRUNC OWRONLY OCREAT OAPPEND ORDWR OCREAT OTRUNC ORDWR OCREAT OAPPEND bool bufown; true if allocated by stdio.h or false by a user char lastop; r or w bool eof; true if EOF is reached ; #include "stdio.cpp #endif When opening a file, the fopen function receives not only the file name to open but also various file access modes: r Open text file for reading. r Open for reading and writing. w Truncate file to zero length or create text file for writing. w Open for reading and writing. The file is created if it does not exist, otherwise truncated. a Open for appending writing at end of file The file is created if it does not exist. a Open for reading and appending writing at end of file The file is created if it does not exist. The initial file position for reading is at the beginning of the file, but output is alway appended to the end of the file. The fopen function
Help needed: University of Washington Bothell
CSS: System Programming
Program
C Standard IO Library
Purpose
In this programming assignment you will design and implement your own core input and output functions of the CC standard IO library: stdio.h
Linux IO
Unix provide system calls for file IO such as open read write and lseek However, these system calls are not supported on nonUnix based systems such as Window. Therefore, the CC standard IO library was created so that applications which require these functions can be easily ported through recompilation across these systems.
CC Standard IO Library Overview
The standard IO library is an architecture independent library that allows CC programs to read and write files instead of directly calling the underlying OS system calls. The library's functions add buffering and provide a userfriendly file stream interface FILE The file stream interface is implemented by the standard IO library utilizing the underlying system calls. When reading andor writing small bytecounts eg reading one line at a time from a file buffered functions are faster.
The read and write system calls operate on file descriptors and readwrite fromto buffers not strings. A file descriptor is just an integer referring to a currently open file. The OS uses that number as an index into the file descriptor table of files currently in use to access the actual device eg disk, network, terminal
On the other hand, file streams operators freadfwrite interact directly with file streams: FILE File streams are dynamically allocated and allow readingwriting raw data. File stream operators, fread and fwrite use the type void since there are no dataspecific requirements.
The core input and output functions defined in include:
Function name Description
fopen opens a file
fflush synchronizes an output stream with the actual file
setbuf, setvbuf sets the size of an inputoutput stream buffer
fpurge clears an inputoutput stream buffer
fread reads from a file
fwrite writes to a file
fgetc reads a character from a file stream
fputc writes a character to a file stream
fgets reads a character string from a file stream
fputs writes a character string to a file stream
fseek moves the file position to a specific location in a file
feof checks for the endoffile
fclose closes a file
printf prints formatted output to stdout
Figure below shows how the standard IO library utilizes a buffer to reduce the number of read and write system calls with filestreams.
Figure This figure shows Standard IO functions using FILE instead of file descriptor.
FILE Data Structure and fopen
Upon a file open, fopen returns a pointer to a FILE object that maintains the attributes of the opened file.
On canvas is posted a version of the header file we will use for the project. The following shows the class FILE definition:
#ifndef MYSTDIOH
#define MYSTDIOH
#define BUFSIZ default buffer size
#define IONBF unbuffered
#define IOLBF line buffered. Do not need to implement this mode.
#define IOFBF fully buffered
#define EOF end of file
class FILE
public:
FILE :
fd pos bufferchar size actualsize
modeIONBF flag bufownfalse lastop eoffalse
int fd; a Unix file descriptor of an opened file
int pos; the current file position in the buffer
char buffer; an input or output file stream buffer
int size; the buffer size
int actualsize; actual buffer size when read returns # bytes smaller than size
int mode; IONBF, IOLBF, IOFBF. You do not need to implement IOLBF.
int flag; ORDONLY
ORDWR
OWRONLY OCREAT OTRUNC
OWRONLY OCREAT OAPPEND
ORDWR OCREAT OTRUNC
ORDWR OCREAT OAPPEND
bool bufown; true if allocated by stdio.h or false by a user
char lastop; r or w
bool eof; true if EOF is reached
;
#include "stdio.cpp
#endif
When opening a file, the fopen function receives not only the file name to open but also various file access modes:
r Open text file for reading.
r Open for reading and writing.
w Truncate file to zero length or create text file for writing.
w Open for reading and writing. The file is created if it does not exist, otherwise truncated.
a Open for appending writing at end of file The file is created if it does not exist.
a Open for reading and appending writing at end of file The file is created if it does not exist. The initial file position for reading is at the
beginning of the file, but output is alway appended to the end of the file.
The fopen function
Step by Step Solution
There are 3 Steps involved in it
Step: 1
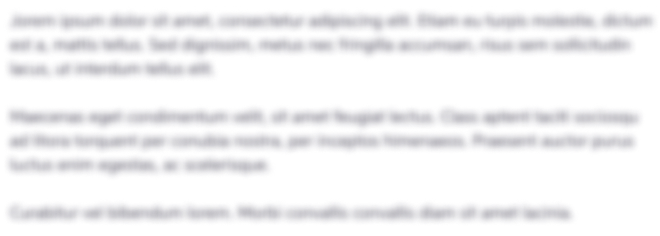
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started