Question
HELP PLEASE! You'll find that it contains several methods that have not yet been finished. For now, the methods contain only a placeholder return value
HELP PLEASE! You'll find that it contains several methods that have not yet been finished.
For now, the methods contain only a placeholder return value so that the code will compile. Fill in your own implementation.
The uniques() method is extra credit. If you plan to implement it, uncomment it and fill in your implementation.
You'll also want to uncomment the corresponding unit test already written for you. The test method for counts() is also missing.
Please implement this. So far, you've been using the JUnit methods:
assertTrue()
assertFalse()
assertEquals()
We now have assertArrayEquals(A, B) which tests if the contents of the arrays that A and B reference are the same.
You can see its use in some of the other test methods. You'll find it useful for the test you're writing. You may not use the Arrays class in your methods for this assignment.
package arraypractice;
import org.junit.After; import org.junit.Before; import org.junit.Test; import static org.junit.Assert.*;
public class ArrayPracticeTest { /** * Test of initialize method, of class ArrayPractice. */ @Test public void testInitialize() { System.out.println("initialize"); int[] A = new int[5]; int initialValue = 5; ArrayPractice.initialize(A, initialValue); assertArrayEquals(new int[]{5,5,5,5,5}, A); }
/** * Test of average method, of class ArrayPractice. */ @Test public void testAverage() { System.out.println("average"); int[] A = {10,20,30,40,50}; double expResult = 30.0; double result = ArrayPractice.average(A); assertEquals(expResult, result, 0.1); int B[] = {31}; expResult = 31.0; result = ArrayPractice.average(B); assertEquals(expResult, result, 0.1); }
/** * Test of numOccurrences method, of class ArrayPractice. */ @Test public void testNumOccurrences() { System.out.println("numOccurrences"); int[] A = {21,16,5,31,8,1,9,1,16,4,2,16}; int x = 16; int expResult = 3; int result = ArrayPractice.numOccurrences(A, x); assertEquals(expResult, result); x=37; expResult=0; result = ArrayPractice.numOccurrences(A, x); assertEquals(expResult, result); x=21; expResult=1; result = ArrayPractice.numOccurrences(A, x); assertEquals(expResult, result); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
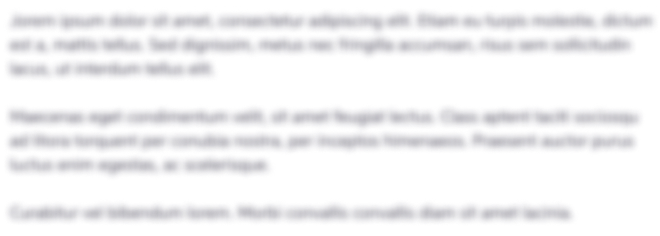
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started