Question
Help! Why isn't my code working properly? It only finds 185 duplicates in the list, but it should find 199. How the program should work:
Help! Why isn't my code working properly? It only finds 185 duplicates in the list, but it should find 199.
How the program should work:
Write DvcSchedule4.cpp to read and parse the 70,000 line dvc-schedule.txt (Links to an external site.)Links to an external site. text file, and find each subject code in the file. Output each code to the console screen, in alphabetical order, with the number of classes offered under that code. Use your own DynamicArray template from Lab Assignment 3. Do NOT use any STL containers, and do NOT modify your H except to make corrections. Submit the H file, even if there are no corrections since lab 3. Canvas will add it's version tracking to the file's name -- that's okay.
Parse. Start with the simple parsing code and see if you can read and parse the TXT file.
Count. Then add your code to count how many subject codes and how many sections of each.
Duplicate Check. Then add your code to track and exclude duplicates from counting.
Link to .txt file: https://drive.google.com/file/d/0B14YTZL55whCSGE0VjcyajZxSmc/view
Code DvcSchedule4.cpp:
#include
#include
#include
#include
#include "StaticArray.h"
#include
using namespace std;
struct SubjectCode {
string name;
int count;
inline bool operator<(SubjectCode arg) {
if (name.compare(arg.name) < 0) {
return true;
}
return false;
}
};
struct Node {
SubjectCode data;
Node* next;
};
struct termAndSection {
string semester;
string sectionNumber;
};
bool foundData(SubjectCode);
int main() {
cout << " Casey Bender" << endl;
cout << "Lab 4, The \"DvcSchedule4\" Program " << endl;
cout << endl;
cout << endl;
char* token;
char buf[1000];
Node* start = 0;
Node* p;
Node *prev;
Node* q;
array
string line;
int dupliCount =0;
bool dupliCourse;
bool found;
clock_t startTime = clock();
double elapsedSeconds;
ifstream fin;
fin.open("dvc-schedule.txt");
if (!fin.good()) {
cout << "Unable to open file" << endl;
}
while (!fin.eof()) {
dupliCourse = false;
found = false;
getline(fin, line);
strcpy(buf, line.c_str());
if (buf[0] == 0) continue;
const string term(token = strtok(buf, "\t"));
const string section(token = strtok(NULL, "\t"));
const string course(token = strtok(NULL, "\t, -"));
for (int i = 1; i < 1000; i++) {
if (term.compare(data1[i].semester) == 0 &&
section.compare(data1[i].sectionNumber) == 0) {
dupliCourse = true;
}
}
if (dupliCourse == true) {
dupliCount++;
} else {
for (int i = 1; i < 1000; i++) {
data1[i].semester = term;
data1[i].sectionNumber = section;
}
p = start;
while (p != NULL && !found) {
if (p->data.name.compare(course) == 0) {
found = true;
}
if (!found) {
p = p->next;
}
}
if (found) {
p->data.count++;
} else {
Node* node = new Node;
node->data.name = course;
node->data.count = 1;
node->next = start;
start = node;
}
}
}
fin.close();
for (p = start; p; p = p->next) {
for (q = p->next; q; q = q->next) {
if (q->data < p->data) {
SubjectCode temp = p->data;
p->data = q->data;
q->data = temp;
}
}
}
elapsedSeconds = (double)(clock() - startTime) / CLOCKS_PER_SEC;
for (p = start;p ;p = p->next) {
if (p -> data.name != "course")
cout << p->data.name << ", " << p->data.count << " Classes" << endl;
}
cout << " The duplicates entries are " << dupliCount << endl;
cout << "The processing time is: " << elapsedSeconds << " seconds." << endl;
cout << endl;
cout << endl;
return 0;
}
Header: StaticArray.h
#ifndef StaticArray_H
#define StaticArray_H
template
class Array{
public:
Array();
inline DataType& operator[](int);
int getCapacity() const;
int lsearch(const DataType&) const;
private:
DataType data[CAPACITY];
int index;
DataType dummy;
};
template
Array
}
template
inline DataType& Array
if (index < 0)
return dummy;
if (index >= CAPACITY)
return dummy;
return data[index];
}
template
int Array
return CAPACITY;
}
template
int Array
for (int i = 0; i < CAPACITY; i++) {
if(arg == data[i]) {
return i;
}
}
return -1;
}
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
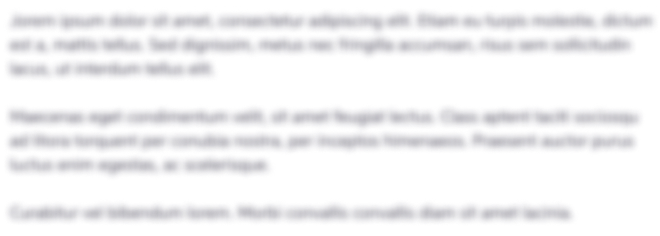
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started