Question
Help with part A) of this question 5.a) If the light is blocked by 50% or more, LED 1 will turn on (think of it
Help with part A) of this question
5.a) If the light is blocked by 50% or more, LED 1 will turn on (think of it as the Room Light), and once the light in not blocked LED1 will turn back off. LED should not flicker as it alternates between on and off. To prevent that from happening, apply the hysteresis function.
5.b) If the room temperature reading increases by 2% over the room value, LED 2 turns on (think of it as the Air Conditioner). If the temperature decreases to the original room temperature or below it, LED 2 will turn off. LED should not flicker!
5.c) Every time you touch the touch switch, LED 3 will toggle between on and off (think of it as your Alarm System). LED 3 should not flicker!
#include
int value=0, i=0 ; int light = 0, lightroom = 0, dimled=50; int temp = 0, temproom = 0; int touch =0, touchroom =0; int flag =0; int ADCReading [3];
// Function Prototypes void fadeLED(int valuePWM); void ConfigureAdc(void); void getanalogvalues();
int main(void) { WDTCTL = WDTPW + WDTHOLD; // Stop WDT P1OUT = 0; P2OUT = 0; P1DIR = 0; P1REN = 0; P2REN = 0; P2DIR = 0; P1DIR |= ( BIT4 | BIT5 | BIT6); // set bits 4, 5, 6 as outputs P2DIR |= BIT0; // set bit 0 as outputs
ConfigureAdc();
// reading the initial room values, lightroom, touchroom, temproom __delay_cycles(250); getanalogvalues(); lightroom = light; touchroom = touch; temproom = temp; __delay_cycles(250);
for (;;) { // reading light, touch, and temp repeatedly at the beginning of the main loop getanalogvalues();
//light controlling LED2 on launchpad (P1.6) via variable dimled dimled = light; //use the light reading range limits 50-900, and conver them to 0-100% dimled = ((dimled- 50)*100)/(900- 50); if(dimled <= 5)dimled = 0; else if (dimled >=99)dimled = 100; fadeLED(dimled);
//light Controlling LED1 //Create dead zone of no action to avoid flickering (switching on/off over a a small fluctuating value on light //I chose the range 1.1 to 1.5 of the value, that is no action if (1.1 lightroom < light < 1.5 lightroom) if(light < lightroom * 1.50 && light > lightroom * 1.10) {} else { if(light >= lightroom * 1.50) {P1OUT |= BIT4; __delay_cycles(200);} // on if dark if(light <= lightroom * 1.10) {P1OUT &= ~BIT4; __delay_cycles(200);} // off if light }
// ******************************************* // beginning of area for all changes
//Temperature Controlling LED2 //use a dead zone for no action between 1.01-1.03 of the temoroom value if(temp < temproom * 1.03 && temp > temproom * 1.01) {} else { //+++ enter student here code for temerature //if temp is higher than 1.03 * temproom, turn LED2 on //if temp is lower than 1.01 * temproom, turn LED2 off
}
//Touch Controlling LED3 //use a dead zone for no action between 0.07-0.09 of the value temp if(touch > touchroom * 0.7 && touch < touchroom * 0.9) {} else { //+++ enter student code for toggle //the two lines below make a simple turn-on while still touching, off when not touching //Chnage the code so that with every touch, LED3 toggles and stay if(touch >= touchroom * 0.9) {P2OUT &= ~BIT0; __delay_cycles(200);} // off if(touch <= touchroom * 0.7) {P2OUT |= BIT0; __delay_cycles(200);} // on }
// end of area for all changes // *******************************************
} }
void ConfigureAdc(void) { ADC10CTL1 = INCH_2 | CONSEQ_1; // A2 + A1 + A0, single sequence ADC10CTL0 = ADC10SHT_2 | MSC | ADC10ON; while (ADC10CTL1 & BUSY); ADC10DTC1 = 0x03; // 3 conversions ADC10AE0 |= (BIT0 | BIT1 | BIT2); // ADC10 option select }
void fadeLED(int valuePWM) { P1SEL |= (BIT6); // P1.0 and P1.6 TA1/2 options CCR0 = 100 - 0; // PWM Period CCTL1 = OUTMOD_3; // CCR1 reset/set CCR1 = valuePWM; // CCR1 PWM duty cycle TACTL = TASSEL_2 + MC_1; // SMCLK, up mode }
void getanalogvalues() { i = 0; temp = 0; light = 0; touch =0; // set all analog values to zero for(i=1; i<=5 ; i++) // read all three analog values 5 times each and average { ADC10CTL0 &= ~ENC; while (ADC10CTL1 & BUSY); //Wait while ADC is busy ADC10SA = (unsigned)&ADCReading[0]; //RAM Address of ADC Data, must be reset every conversion ADC10CTL0 |= (ENC | ADC10SC); //Start ADC Conversion while (ADC10CTL1 & BUSY); //Wait while ADC is busy light += ADCReading[0]; // sum all 5 reading for the three variables touch += ADCReading[1]; temp += ADCReading[2]; } light = light/5; touch = touch/5; temp = temp/5; // Average the 5 reading for the three variables }
#pragma vector=ADC10_VECTOR __interrupt void ADC10_ISR(void) { __bic_SR_register_on_exit(CPUOFF); }
P.S the set up is as such
The temperature sensor MCP9700 or MCP9701 is connected to Port1.0 (A0). It is linear and it outputs a 0.1 V for every degree, for example a room temperature of 78 F degrees, would show as a .78 V signal, which is translated into 10-b digital value as .68 x (1023/3.3V) = 210. The exact value will fluctuate due to the imprecision of the sensor and the noise around it.
The touch switch is connected to Port1.1 (A1) and a 100K ohm pull-up resistor. When you touch the transistor base, your body acts like a ground sinking a very little current form the PNP transistor, which is enough to trigger it due to its high gain (1000). So when you touch the base and the transistor is turned on, the collector voltage will drop form VCC level (#1000) to almost ground (#50). To help your body to serve as a better GND, touch the GND as well.
The photo cell is connected to Port1.2 (A2). With the 10K resistor in series working a voltage divider, you read about .97 VDC on A2 at room light. When you block the light completely from the photo sensor, the reading goes higher to 3.1 V. When you apply direct light onto the sensor, the reading goes lower to 0.3 V.
LEDs 123 are connected to Port pins 1.4, 1.5, 2.0. LEDs must have current limiting resistors in series with value between 100-470 ohms.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
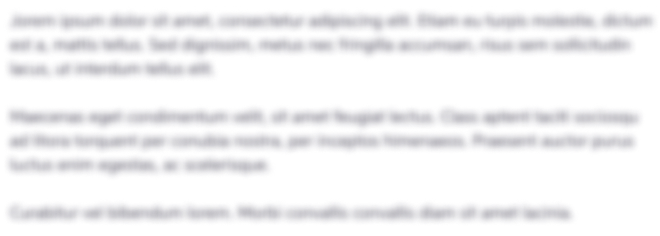
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started