Answered step by step
Verified Expert Solution
Question
1 Approved Answer
help with PHP problem set. #!/usr/bin/php -d display_errors
help with PHP problem set. #!/usr/bin/php -d display_errors 5) { return 1; } } } loopInFunction(); //calls the loop in a function code //@TODO How many times will that loop run? Try to guess before running the code. // Write your answer here: // //example of break $i = 0; while(true){ print($i++ . " "); if ($i > 10) { break; } } // The Division Algorithm */ /** * This method implements the division algorithm. * * It takes as input $a as an integer * and d as a positive integers, computes a/d, * and outputs an array contatining the quotient q and the remainder r. */ function divisionAlg(int $a, int $d) { if ($d < 1) { throw new InvalidArgumentException('divisionAlg requires divisor be positive. Input was: ' . $d); } //@TODO write what this thing does // Your Answer: $r = $a; //this will become the remainder $q = 0; //this will become the quotient /* * This handles negatives. We did not do this part of the algorithm in class. */ while ($r < 0) { $r = $r + $d; $q--; print( "r: " . $r . "\tq: " . $q . " " ); //debug code } /** * @TODO Comment the above loop, so that someone who did not quite understand * could read the code and understand what it does. */ while ($d <= $r) { $r = $r - $d; $q++; print( "r: " . $r . "\tq: " . $q . " " ); //debug code } /** * @TODO Comment the above loop, so that someone who did not quite understand * could read the code and understand what it does. */ return array( "q" => $q, "r" => $r ); } print_r(divisionAlg(28, 5)); print_r(divisionAlg(-26, 5)); /** * Naive GCD Calculator * * The following will compute the gcd of two numbers, using the "definition" of gcd we did in class. */ function getGCD(int $a, int $b) { if ($a < 1 || $b < 1) { throw new InvalidArgumentException('getGCD requires non-negative integers. Input was: ' . $a . 'and' . $b); } if($a > $b) { $maxtocheck = $b; } else { $maxtocheck = $a; } $k = 1; $currentGCD = 1; while($k <= $maxtocheck) { if(($a % $k == 0) && ($b % $k == 0)) { //@TODO Write this condition in english: $currentGCD = $k; } print_r(array( "k" => $k, "currentGCD" => $currentGCD)); //@TODO what does this line do? $k++; } return $currentGCD; } print (" The GCD of 9 and 495 is ... " . getGCD(9,495)); //print (" The GCD of 105280 and 495 is ... " . getGCD(105280,495)); //@TODO How many times would the program loop to compute the above line? // Write your answer here: /** * @TODO * Write the function implementing the Euclidean algorithm * You must * * Use a while(true) loop * * Have a break statement to exit the loop. * * Use the algorithm I gave in class * * Use the variable names I used in class * * Each time your loop runs, it must print your current $smaller, $bigger, $newsmaller * * A piece of code that should work is proveded * You may NOT use any of the solutions online (I purposely made the above instructions to thwart you) */ function getGCDEuclid(int $a, int $b) { if ($a < 1 || $b < 1) { throw new InvalidArgumentException('getGCD requires non-negative integers. Input was: ' . $a . 'and' . $b); } //// YOUR CODE STARTS HERE (the bellow snippits have been provided) //while(true) { //print_r(array("smaller" => $smaller, // "bigger" => $bigger, // "newsmaller" => $newsmaller)); //} } print (" The GCD of 105280 and 495 is ... " . getGCDEuclid(105280,495));
.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
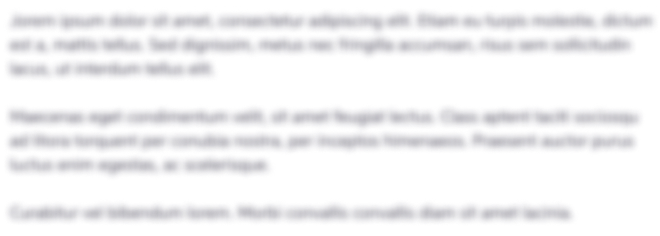
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started