Question
HELP WITH PLAIT CODING LANGUAGE (DrRacket): The interpreter with function values plait code that needs to be updated according to part 1, 2 and 3:
HELP WITH PLAIT CODING LANGUAGE (DrRacket):
The "interpreter with function values" plait code that needs to be updated according to part 1, 2 and 3:
#lang plait
(define-type Value (numV [n : Number]) (closV [arg : Symbol] [body : Exp] [env : Env]))
(define-type Exp (numE [n : Number]) (idE [s : Symbol]) (plusE [l : Exp] [r : Exp]) (multE [l : Exp] [r : Exp]) (letE [n : Symbol] [rhs : Exp] [body : Exp]) (lamE [n : Symbol] [body : Exp]) (appE [fun : Exp] [arg : Exp]))
(define-type Binding (bind [name : Symbol] [val : Value]))
(define-type-alias Env (Listof Binding))
(define mt-env empty) (define extend-env cons)
(module+ test (print-only-errors #t))
;; parse ---------------------------------------- (define (parse [s : S-Exp]) : Exp (cond [(s-exp-match? `NUMBER s) (numE (s-exp->number s))] [(s-exp-match? `SYMBOL s) (idE (s-exp->symbol s))] [(s-exp-match? `{+ ANY ANY} s) (plusE (parse (second (s-exp->list s))) (parse (third (s-exp->list s))))] [(s-exp-match? `{* ANY ANY} s) (multE (parse (second (s-exp->list s))) (parse (third (s-exp->list s))))] [(s-exp-match? `{let {[SYMBOL ANY]} ANY} s) (let ([bs (s-exp->list (first (s-exp->list (second (s-exp->list s)))))]) (letE (s-exp->symbol (first bs)) (parse (second bs)) (parse (third (s-exp->list s)))))] [(s-exp-match? `{lambda {SYMBOL} ANY} s) (lamE (s-exp->symbol (first (s-exp->list (second (s-exp->list s))))) (parse (third (s-exp->list s))))] [(s-exp-match? `{ANY ANY} s) (appE (parse (first (s-exp->list s))) (parse (second (s-exp->list s))))] [else (error 'parse "invalid input")]))
(module+ test (test (parse `2) (numE 2)) (test (parse `x) (idE 'x)) (test (parse `{+ 2 1}) (plusE (numE 2) (numE 1))) (test (parse `{* 3 4}) (multE (numE 3) (numE 4))) (test (parse `{+ {* 3 4} 8}) (plusE (multE (numE 3) (numE 4)) (numE 8))) (test (parse `{let {[x {+ 1 2}]} y}) (letE 'x (plusE (numE 1) (numE 2)) (idE 'y))) (test (parse `{lambda {x} 9}) (lamE 'x (numE 9))) (test (parse `{double 9}) (appE (idE 'double) (numE 9))) (test/exn (parse `{{+ 1 2}}) "invalid input"))
;; interp ---------------------------------------- (define (interp [a : Exp] [env : Env]) : Value (type-case Exp a [(numE n) (numV n)] [(idE s) (lookup s env)] [(plusE l r) (num+ (interp l env) (interp r env))] [(multE l r) (num* (interp l env) (interp r env))] [(letE n rhs body) (interp body (extend-env (bind n (interp rhs env)) env))] [(lamE n body) (closV n body env)] [(appE fun arg) (type-case Value (interp fun env) [(closV n body c-env) (interp body (extend-env (bind n (interp arg env)) c-env))] [else (error 'interp "not a function")])]))
(module+ test (test (interp (parse `2) mt-env) (numV 2)) (test/exn (interp (parse `x) mt-env) "free variable") (test (interp (parse `x) (extend-env (bind 'x (numV 9)) mt-env)) (numV 9)) (test (interp (parse `{+ 2 1}) mt-env) (numV 3)) (test (interp (parse `{* 2 1}) mt-env) (numV 2)) (test (interp (parse `{+ {* 2 3} {+ 5 8}}) mt-env) (numV 19)) (test (interp (parse `{lambda {x} {+ x x}}) mt-env) (closV 'x (plusE (idE 'x) (idE 'x)) mt-env)) (test (interp (parse `{let {[x 5]} {+ x x}}) mt-env) (numV 10)) (test (interp (parse `{let {[x 5]} {let {[x {+ 1 x}]} {+ x x}}}) mt-env) (numV 12)) (test (interp (parse `{let {[x 5]} {let {[y 6]} x}}) mt-env) (numV 5)) (test (interp (parse `{{lambda {x} {+ x x}} 8}) mt-env) (numV 16))
(test/exn (interp (parse `{1 2}) mt-env) "not a function") (test/exn (interp (parse `{+ 1 {lambda {x} x}}) mt-env) "not a number") (test/exn (interp (parse `{let {[bad {lambda {x} {+ x y}}]} {let {[y 5]} {bad 2}}}) mt-env) "free variable")
#; (time (interp (parse '{let {[x2 {lambda {n} {+ n n}}]} {let {[x4 {lambda {n} {x2 {x2 n}}}]} {let {[x16 {lambda {n} {x4 {x4 n}}}]} {let {[x256 {lambda {n} {x16 {x16 n}}}]} {let {[x65536 {lambda {n} {x256 {x256 n}}}]} {x65536 1}}}}}}) mt-env)))
;; num+ and num* ---------------------------------------- (define (num-op [op : (Number Number -> Number)] [l : Value] [r : Value]) : Value (cond [(and (numV? l) (numV? r)) (numV (op (numV-n l) (numV-n r)))] [else (error 'interp "not a number")])) (define (num+ [l : Value] [r : Value]) : Value (num-op + l r)) (define (num* [l : Value] [r : Value]) : Value (num-op * l r))
(module+ test (test (num+ (numV 1) (numV 2)) (numV 3)) (test (num* (numV 2) (numV 3)) (numV 6)))
;; lookup ---------------------------------------- (define (lookup [n : Symbol] [env : Env]) : Value (type-case (Listof Binding) env [empty (error 'lookup "free variable")] [(cons b rst-env) (cond [(symbol=? n (bind-name b)) (bind-val b)] [else (lookup n rst-env)])]))
(module+ test (test/exn (lookup 'x mt-env) "free variable") (test (lookup 'x (extend-env (bind 'x (numV 8)) mt-env)) (numV 8)) (test (lookup 'x (extend-env (bind 'x (numV 9)) (extend-env (bind 'x (numV 8)) mt-env))) (numV 9)) (test (lookup 'y (extend-env (bind 'x (numV 9)) (extend-env (bind 'y (numV 8)) mt-env))) (numV 8)))
Honework 3 - Bools, Scope Elinination, and Thunks Code s.bnitted without conplete test coverage will result in an a-tanatic 205 penalty. This assignnent is an individ.al sssigrent. Part 1 - Booleans Start with the interpreter with function values, and extend the irplenentation to support boolean literals, an equality test, and a conditional forn: The - operator should only work on nunber values, and if should only work when the value of the first subexpression is a boolean. Feport a "not a nunber" error if a subexpression - produces a non-nutber value and report a "not a boolean" error when the first subexpression of if does not produce a boolean. The if forn should evaluate its second subexpression only when the first subexpression's value is true, and it should evaluate its third subexpression only when the first subexpression's value is false. True and false are always spelled true and false, not =t or f. Note that you not only need to extend Exp with new kinds of expressions, but you will also need to add booleans to value. For example, true should produce a true value, while \{if true {12} s } should produce 3 , and {1f23} should report a "not a boolean" error. As usual, update parse to support the extended language. More examples: (test (interp (parse { if {=2{+11}}7 s } should produce 1 . As before, you rust update the parse function. Sone examples: Part 3 - Thunks A thuak is like a function of zero argunents, whose purpose is to delay a conputation. Extend your interpreter with a delay forn that creates a thunk, and a force forn that causes a thunk's expression to be evaluated: A thunk is a new kind of value, like a nurber, function, or boolean. For example, {delay}1+1{ lanbda {x}x}}} produces a thunk value without complaining that a function is not a nutber, whle { force { delay {1 tanbda {x}x}}}} triggers a "not a nutber" erroz. As another example, \{Let { Cok { delay {+12}}1} \{let {[ bed { delay {+1 false }}]} \{force ok\}\}\} produces 3, while \{let { Cok { delay {+12}}]}. { let {[ bad { delay {+1 false }}} { force bad }}} triggers a "not a nutber" erroz. More exanplesStep by Step Solution
There are 3 Steps involved in it
Step: 1
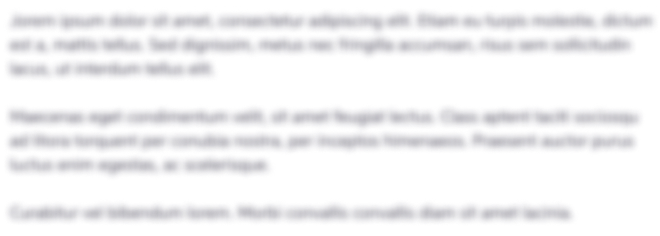
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started