Question
Help with this Java project please. I listed the departmentstore, departmentstoretest, staffmember, staffmemberio, hourly, exective and the file employees.txt below. DEPARTMENT STORE: package departmentstorepkg; public
Help with this Java project please. I listed the departmentstore, departmentstoretest, staffmember, staffmemberio, hourly, exective and the file employees.txt below.
DEPARTMENT STORE:
package departmentstorepkg;
public class DepartmentStore
{
private static final int DEFAULT_SIZE = 10;
private StaffMember [] myEmployees;
private int myNumberEmployees;
private String myFileName;
public DepartmentStore(String filename)
{
myFileName = filename;
initialize( );
}
public void awardBonus(double amount){
}
public Employee findEmployee(String SSN){
for (int i = 0; i
Employee sm = (Employee) myEmployees[i];
if (sm.getSocialSecurityNumber().equals (SSN));
return sm;
}
return null;
}
public void updateHours(String SSN, int hours){
}
public void print(){
for (int i = 0; i
StaffMember sm = myEmployees[i];
System.out.println(sm);
System.out.println();
}
}
public void printEmployees(){
System.out.println(myEmployees);
}
public void printExecutives(){
}
public void printExecutivesPay(){
}
public void printHourly(){
}
private void initialize( )
{
myEmployees = new StaffMember[DEFAULT_SIZE];
myNumberEmployees = 0;
StaffMemberIO empIO = new StaffMemberIO(myFileName);
StaffMember t;
while(( t = empIO.getNext()) != null){
if(myNumberEmployees >= myEmployees.length)
resize();
myEmployees[myNumberEmployees] = t;
myNumberEmployees++;
}
}
private void resize( )
{
StaffMember [] temp = new StaffMember[2*myEmployees.length];
for (int i = 0; i
temp[i] = myEmployees[i];
myEmployees = temp;
}
}
DEPARTMENTSTORE TEST:
package departmentstorepkg;
public class DepartmentStoreTest {
public static void main(String[] args) {
// StaffMemberIO empIn = new StaffMemberIO ("./employees.txt");
// StaffMember sm = empIn.getNext();
// while (sm!=null) {
//
// System.out.println(sm);
//
// if(sm instanceof Executive)
// {
// System.out.println(" Executive");
// System.out.println(sm);
// }
// else if(sm instanceof Hourly)
// {
// System.out.println(" Hourly");
// System.out.println(sm);
// }
// else if(sm instanceof Employee)
// {
// System.out.println(" Employee");
// System.out.println(sm);
// }
// System.out.println();
// sm = empIn.getNext();
}
{
DepartmentStore ds= new DepartmentStore("./employee.txt.");
ds.print();
StaffMember sm = ds.findEmployee("123-45-6789");
if (sm != null) {
System.out.println("found");
System.out.println(sm);
}
else {
System.out.println("employee not found");
}
}
}
EMPLOYEE:
package departmentstorepkg;
class Employee extends StaffMember
{
protected String socialSecurityNumber;
protected double payRate;
//-----------------------------------------------------------------
// Sets up an employee with the specified information.
//-----------------------------------------------------------------
public Employee (String name, String address, String phone,
String socialSecurityNumber, double payRate)
{
super (name, address, phone);
this.socialSecurityNumber = socialSecurityNumber;
this.payRate = payRate;
}
//-----------------------------------------------------------------
// Returns information about an employee as a string.
//-----------------------------------------------------------------
public String toString ()
{
String result = super.toString();
result += " Social Security Number: " + socialSecurityNumber;
return result;
}
//-----------------------------------------------------------------
// Returns the pay rate for this employee.
//-----------------------------------------------------------------
public double pay ()
{
return payRate;
}
// getter and setter for local private data member
public String getSocialSecurityNumber() {
return socialSecurityNumber;
}
public void setSocialSecurityNumber(String socialSecurityNumber) {
this.socialSecurityNumber = socialSecurityNumber;
}
}
StaffMember:
package departmentstorepkg;
abstract class StaffMember
{
private String name;
private String address;
private String phone;
//-----------------------------------------------------------------
// Sets up a staff member using the specified information.
//-----------------------------------------------------------------
public StaffMember (String name, String address, String phone)
{
this.name = name;
this.address = address;
this.phone = phone;
}
//-----------------------------------------------------------------
// Returns a string including the basic employee information.
//-----------------------------------------------------------------
public String toString ()
{
String result = "Name: " + name + " ";
result += "Address: " + address + " ";
result += "Phone: " + phone;
return result;
}
//-----------------------------------------------------------------
// Derived classes must define the pay method for each employee
// type.
//-----------------------------------------------------------------
public abstract double pay();
// getters and setters (mutators) for the private data members of this abstract class
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
}
StaffMemberIO:
package departmentstorepkg;
import java.io.*;
import java.util.*;
public class StaffMemberIO
{
private String myFileName;
private BufferedReader myInfile;
private Scanner scan;
public StaffMemberIO(String filename)
{
myFileName = filename;
openFile();
scan = new Scanner (myInfile);
}
public String toString( )
{
return "[" + myFileName + "]";
}
public StaffMember getNext( )
{
if (scan == null)
return null;
try
{
String typeStr = scan.nextLine();
char type = typeStr.charAt(0);
String name = scan.nextLine();
String address = scan.nextLine();
String phone = scan.nextLine();
String socialSecurity = scan.nextLine();
double pay = scan.nextDouble();
scan.nextLine();
switch (type)
{
case 'e': case 'E':
return new Employee(name, address, phone, socialSecurity, pay);
case 'x': case 'X':
return new Executive(name, address, phone, socialSecurity, pay);
case 'h': case 'H':
return new Hourly(name, address, phone, socialSecurity, pay);
}
return null;
}
catch (Exception e){
closeFile( );
return null;
}
}
private void closeFile( )
{
if (myInfile == null)
return;
try
{
myInfile.close( );
}
catch (IOException e) { }
}
private void openFile( )
{
myInfile = null;
try
{
FileReader fr = new FileReader(myFileName);
myInfile = new BufferedReader(fr);
}
catch (IOException e) {System.err.println("Didn't open " + myFileName);}
}
}
Hourly:
package departmentstorepkg;
class Hourly extends Employee
{
private int hoursWorked;
//-----------------------------------------------------------------
// Sets up this hourly employee using the specified information.
//-----------------------------------------------------------------
public Hourly (String name, String address, String phone,
String socialSecurityNumber, double payRate)
{
super (name, address, phone, socialSecurityNumber, payRate);
hoursWorked = 0;
}
//-----------------------------------------------------------------
// Adds the specified number of hours to this employee's
// accumulated hours.
//-----------------------------------------------------------------
public void addHours (int moreHours)
{
hoursWorked += moreHours;
}
//-----------------------------------------------------------------
// Computes and returns the pay for this hourly employee.
//-----------------------------------------------------------------
public double pay ()
{
double payment = payRate * hoursWorked;
hoursWorked = 0;
return payment;
}
//-----------------------------------------------------------------
// Returns information about this hourly employee as a string.
//-----------------------------------------------------------------
public String toString ()
{
String result = super.toString();
result += " Current hours: " + hoursWorked;
return result;
}
}
Executive:
package departmentstorepkg;
class Executive extends Employee
{
private double bonus;
//-----------------------------------------------------------------
// Sets up an executive with the specified information.
//-----------------------------------------------------------------
public Executive (String name, String address, String phone,
String socialSecurityNumber, double payRate)
{
super (name, address, phone, socialSecurityNumber, payRate);
bonus = 0; // bonus has yet to be awarded
}
//-----------------------------------------------------------------
// Awards the specified bonus to this executive.
//-----------------------------------------------------------------
public void awardBonus (double execBonus)
{
bonus = execBonus;
}
//-----------------------------------------------------------------
// Computes and returns the pay for an executive, which is the
// regular employee payment plus a one-time bonus.
//-----------------------------------------------------------------
public double pay ()
{
double payment = super.pay() + bonus;
bonus = 0;
return payment;
}
}
EMPLOYEES.TXT
x
Mary Jones
123 Main Line
555-0469
123-45-6789
1923.07
E
Joe Smith
3423 UCA Blvd
593-3243
323-23-5666
846.15
h
Alven Chipmonk
32 Hollywood Lane
233-3343
212-33-6978
7.40
x
John Dirk
2334 Hello Way
980-3323
623-34-2399
1732.32
x
Holly Henley
233 IH 10 West
877-7632
345-67-8934
1500.00
h
Doris Day
432 Blanco Road
766-3324
658-45-9234
8.85
.Write the initialize method that instantiates a staffMemberIo object for nyFileName and reads the information into myEmployees. SetmyNumbe rEmp loyees appropriately. Call resize if necessary .Write the following print methods of Departmentstore and add client code in DepartmentStoreTest to test them: print - prints the information about every employee. Include their classification in the printout. printEmployees - prints all the information about each employee who is an employee, but not an executive or hourly. (You will need to use the instanceof operator here.) printExecutives - prints all the information about each executive employee. (You will need to use the instanceof operator here.) printHourly prints all the information about each hourly employee. (You will need to use the instanceof operator here.) o o o Write a findEmployee (String ssN) method in Departmentstore that returns a Employee object corresponding to the specified employee or null if the employee is not found. Add client code to pepartmentstoreTest to test this method. (Notice that staffMember, doesn't have a field corresponding to the social security number. This is an example of the use of a protected field among subclasses.) . Write an updateHours (String sSN, int hours) method in Departmentstore that adds the specified hours to the specified hourly emplovee. Ifthe employee is not an Hourly print out a warning message. Use findEmployee . Write a method wardBonus (double amount) that sets the bonus for each executive to amount. Add client code to DepartmentStoreTest to test this methodStep by Step Solution
There are 3 Steps involved in it
Step: 1
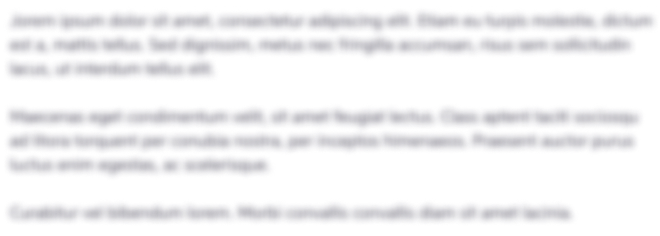
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started