Question
Help writing java program that checks the validity of credit card numbers stored within text files. I am currently struggling with a lab activity for
Help writing java program that checks the validity of credit card numbers stored within text files.
I am currently struggling with a lab activity for my Intro to Computer Science class. I've been learning java for about 2 months now, so my programming skills are still very basic, so bear with me please!
For the sake of saving time, below are screenshots of the assignment prompt. There are two activities in total:
Some clarifications:
We were given 3 separate text files to work from here. For the first activity, we need to read from a text file that contains a list of only credit card numbers that vary in validity. In our main method, we are to call the Luhn check method from activity one to check the validity of the credit card numbers provided in the text file. However, I have already written the code for activity 1 and my code runs fine. For activity 2 though, we are given a text file with a list of customer names and their credit card numbers. As seen in the assignment prompt, we have to populate a 2-D array with the information given in the text file, then call the first method from activity 1 within the second method that will check the 2-D array for valid and invalid credit card numbers. If there are any invalid credit card numbers within the 2-D array of customer names and CC numbers, the program must then write to a separate text file the name of the customer and their respective invalid credit card number. This second activity is what has me absolutely stumped. Here is a screenshot of the text file with customer names and CC numbers:
Lastly, here is my code so far:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class ComprehensiveLab2 {
public static boolean Luhn(String creditCard) {
int sum = 0;
int[] cardArray = new int[creditCard.length()];
for (int i = 0; i
cardArray[i] = Integer.parseInt(creditCard.substring(i, i + 1));
}
for (int i = 0; i
int j = cardArray[i];
j = j * 2;
if (j > 9) {
j = j % 10 + 1;
}
cardArray[i] = j;
}
for (int i = 0; i
sum += cardArray[i];
}
if (sum % 10 == 0) {
return true;
}
else {
return false;
}
}
//Iterative version
public static void checkCustomersIter(String[][] customersInfo) {
}
//Recursive version
public static void checkCustomersRec(String[][] customersInfo) {
}
private static final String creditCardFile = "ccexamples.txt";
private static final String customersFile = "ccandnamesExamples.txt";
public static void main(String[] args) {
try (BufferedReader br = new BufferedReader(new FileReader(creditCardFile))) {
String sCurrentLine;
System.out.println("Credit Card \t Validity");
System.out.println("----------------------------");
while ((sCurrentLine = br.readLine()) != null) {
boolean validNum = Luhn(sCurrentLine);
if (validNum) {
System.out.println(sCurrentLine + " Valid");
}
else {
System.out.println(sCurrentLine + " Invalid");
}
}
}
catch (IOException e) {
e.printStackTrace();
}
}
}
Here is a screenshot of my code after running it:
So as I said before, activity 1 was not a problem for me. However, activity 2 has me beyond confused. How do I go about doing these last 2 methods? Any help is appreciated. I apologize if this post is hard to understand or too long. I'm new to Chegg and this is my first question ever posted! I'm also new to programming and coding, so I still struggle with a lot of the concepts.
18-Fall-CS1101-ComprehensiveLab2-final(8).pdf 1 /4 Use the syntax and semantics of a high-level language to represent: basic variable assignment and arithmetic operations and basic control structures (if-then, for-loop while-loop) Luhn Method Activity 1 Method Luhn. You will have to implement the Luhn Checksum Validation algorithm to check whether a credit card number is valid or fake. This is a widely used algorithm that checks that you enter a valid credit card number when you are trying to make a purchase. Short description of the algorithm [Think Like a Programmer an Introduction to Creative Problem Solving by V. A. Spraul]: Using the original number, double the value of every other digit, starting with the leftmost one, as shown below. Then add the values of the individual digits together (if a doubled value now has two digits, add the digits together - see below). The identification number is valid if the sum is divisible by 10 (i.e., the sum has to be a multiple of 10) 3.1. Testing Example of execution of the algorithm: 8273 1232 7351 0569. Let's see how to check it: Suppose you want to check that your credit card number is valid. You credit card number is First, you are going to double every other number, starting with the first number: 18-Fall-CS1101-ComprehensiveLab2-final(8).pdf 1 /4 Use the syntax and semantics of a high-level language to represent: basic variable assignment and arithmetic operations and basic control structures (if-then, for-loop while-loop) Luhn Method Activity 1 Method Luhn. You will have to implement the Luhn Checksum Validation algorithm to check whether a credit card number is valid or fake. This is a widely used algorithm that checks that you enter a valid credit card number when you are trying to make a purchase. Short description of the algorithm [Think Like a Programmer an Introduction to Creative Problem Solving by V. A. Spraul]: Using the original number, double the value of every other digit, starting with the leftmost one, as shown below. Then add the values of the individual digits together (if a doubled value now has two digits, add the digits together - see below). The identification number is valid if the sum is divisible by 10 (i.e., the sum has to be a multiple of 10) 3.1. Testing Example of execution of the algorithm: 8273 1232 7351 0569. Let's see how to check it: Suppose you want to check that your credit card number is valid. You credit card number is First, you are going to double every other number, starting with the first numberStep by Step Solution
There are 3 Steps involved in it
Step: 1
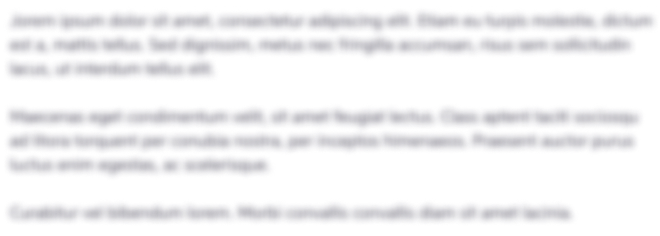
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started