Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Here is a Python program that reads customer data from a list of dictionaries, processes it , and writes the results to a new file.
Here is a Python program that reads customer data from a list of dictionaries, processes it and writes the results to a new file. The program uses control flow, loops, functions, and classes to manipulate data stored in lists and dictionaries. It also utilizes modules to handle file input and output:
class Customer:
def initself name, age, email, phone:
self.name name
self.age age
self.email email
self.phone phone
def readcustomerdatafilename:
Read customer data from a file and return a list of Customer objects.
customers
try:
with openfilenamer as file:
for line in file:
data line.stripsplit
if lendata:
name, age, email, phone data
customer Customername intage email, phone
customers.appendcustomer
except FileNotFoundError:
printfError: File filename not found."
return customers
def writecustomerdatacustomers filename:
Write customer data to a file.
try:
with openfilenamew as file:
for customer in customers:
file.writefcustomernamecustomeragecustomeremailcustomerphone
printfCustomer data has been written to filename successfully."
except Exception as e:
printfError writing to file filename: e
def processcustomerdatacustomerdata:
Process customer data for example, Sort the customer data based on age
sortedcustomers sortedcustomerdata, keylambda x: xage
return sortedcustomers
def main:
inputfilename 'customerinput.txt
outputfilename 'customeroutput.txt
# Read customer data from file
customers readcustomerdatainputfilename
# Process customer data if necessary
customers processcustomerdatacustomers
# Write processed customer data to file
writecustomerdatacustomers outputfilename
if namemain:
main
Explanation:
Customer class: Represents a customer with attributes name, age, email, and phone number.
readcustomerdata: Reads customer data from a file provided as input and returns a list of Customer objects.
writecustomerdata: Writes customer data to a file provided as output.
processcustomerdata: Processes customer data, in this case, sorts the customer data based on age.
main function: Coordinates the entire process. It reads customer data from the input file, processes it if necessary, and then writes the processed data to the output file.Write a pseudocode and control flow chart for the above solution.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
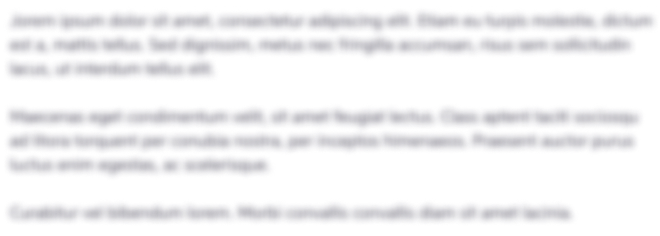
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started