Question
Here is my assignment: I have all of the assignment completed except for the second part of #3: the get accessor should check that the
Here is my assignment:
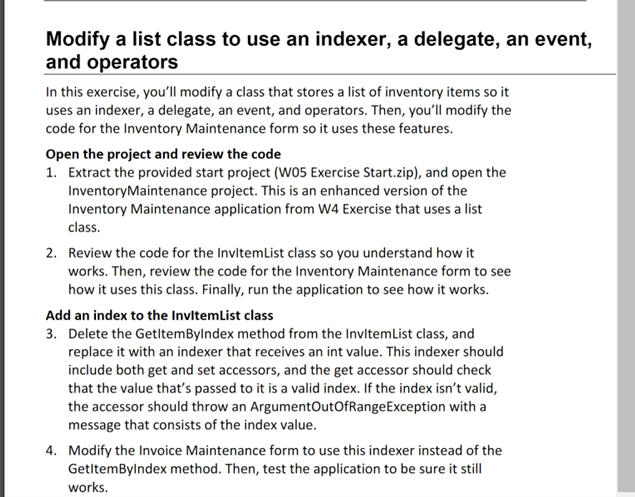
I have all of the assignment completed except for the second part of #3: "the get accessor should check that the value that is passed to it is a valid index. If the index isn't valid, the accessor should throw an ArgumentOutofRangeException with a message that consists of the index value. I would appreciate any tips.
Here is my code - the first part is where the get-set accessor resides for the GetItemByIndex method.
using System;
using System.CodeDom;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
namespace InventoryMaintenance
{
public class InvItemList
{
private List
public delegate void ChangeHandler(InvItemList invItems);//delegate for event specifies what kind of info will be sent along with the event
public event ChangeHandler Changed;// event must use name of delegate
public InvItemList()
{
invItems = new List
}
public int Count => invItems.Count;
// public InvItem GetItemByIndex(int i) => invItems[i];
public InvItem this[int i]
{
get => invItems[i];
set => invItems[i] = value;
}
// throw new throwArgumentOutOfRangeException()
// public void Add(InvItem invItem) => invItems.Add(invItem);
//this where a new item is added, a changed event should be raised and involves invitem object
public void Add(InvItem invItem)
{
invItems.Add(invItem);
Changed(this);
}
public void Add(int itemNo, string description, decimal price)
{
InvItem i = new InvItem(itemNo, description, price);
invItems.Add(i);
}
// public void Remove(InvItem invItem) => invItems.Remove(invItem);
public void Remove(InvItem invItem)
{
invItems.Remove(invItem);
Changed(this);
}
public void Fill() => invItems = InvItemDB.GetItems();
public void Save() => InvItemDB.SaveItems(invItems);
public static InvItemList operator + (InvItemList il, InvItem ii)
{
il.Add(ii);
return il;
}
public static InvItemList operator - (InvItemList il, InvItem ii)
{
il.Remove(ii);
return il;
}
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace InventoryMaintenance
{
public partial class frmInvMaint : Form
{
public frmInvMaint()
{
InitializeComponent();
}
private InvItemList invItems = new InvItemList();
private void frmInvMaint_Load(object sender, EventArgs e)
{
invItems.Changed += new InvItemList.ChangeHandler(InvItemListChanged); //wire the event handler (our delegate), needs to be first before start filling
invItems.Fill();
FillItemListBox();//need for load event
}
private void FillItemListBox()
{
// InvItem item;
lstItems.Items.Clear();
for (int i = 0; i < invItems.Count; i++)
{
//item = invItems.GetItemByIndex(i);
lstItems.Items.Add(invItems[i].GetDisplayText());//use indexer
// lstItems.Items.Add(item.GetDisplayText());
}
}
private void btnAdd_Click(object sender, EventArgs e)
{
frmNewItem newItemForm = new frmNewItem();
InvItem invItem = newItemForm.GetNewItem();
if (invItem != null)
{
//invItems.Add(invItem);
invItems += invItem;//add item to the list
//invItems.Save();//no longer need these two lines because of our new event handler
//FillItemListBox();
}
}
private void btnDelete_Click(object sender, EventArgs e)
{
int i = lstItems.SelectedIndex;
if (i != -1)
{
//InvItem invItem = invItems.GetItemByIndex(i);
InvItem invItem = invItems[i];//use indexer
string message = "Are you sure you want to delete "
+ invItem.Description + "?";
DialogResult button =
MessageBox.Show(message, "Confirm Delete",
MessageBoxButtons.YesNo);
if (button == DialogResult.Yes)
{
//invItems.Remove(invItem);
invItems -= invItem;
// invItems.Save();//no longer need these two because of the new event handler
//FillItemListBox();
}
}
}
private void btnExit_Click(object sender, EventArgs e)
{
this.Close();
}
private void InvItemListChanged(InvItemList invItem)//must have same void typetypes delegate, not a regular method but will be used in a changed event
{
invItem.Save();
FillItemListBox();
}
}
}
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace InventoryMaintenance
{
public partial class frmNewItem : Form
{
public frmNewItem()
{
InitializeComponent();
}
private InvItem invItem = null;
public InvItem GetNewItem()
{
this.ShowDialog();
return invItem;
}
private void btnSave_Click(object sender, EventArgs e)
{
if (IsValidData())
{
invItem = new InvItem(Convert.ToInt32(txtItemNo.Text),
txtDescription.Text, Convert.ToDecimal(txtPrice.Text));
this.Close();
}
}
private bool IsValidData()
{
return Validator.IsPresent(txtItemNo) &&
Validator.IsInt32(txtItemNo) &&
Validator.IsPresent(txtDescription) &&
Validator.IsPresent(txtPrice) &&
Validator.IsDecimal(txtPrice);
}
private void btnCancel_Click(object sender, EventArgs e)
{
this.Close();
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace InventoryMaintenance
{
public static class Validator
{
private static string title = "Entry Error";
public static string Title
{
get
{
return title;
}
set
{
title = value;
}
}
public static bool IsPresent(TextBox textBox)
{
if (textBox.Text == "")
{
MessageBox.Show(textBox.Tag + " is a required field.", Title);
textBox.Focus();
return false;
}
return true;
}
public static bool IsDecimal(TextBox textBox)
{
decimal number = 0m;
if (Decimal.TryParse(textBox.Text, out number))
{
return true;
}
else
{
MessageBox.Show(textBox.Tag + " must be a decimal value.", Title);
textBox.Focus();
return false;
}
}
public static bool IsInt32(TextBox textBox)
{
int number = 0;
if (Int32.TryParse(textBox.Text, out number))
{
return true;
}
else
{
MessageBox.Show(textBox.Tag + " must be an integer.", Title);
textBox.Focus();
return false;
}
}
public static bool IsWithinRange(TextBox textBox, decimal min, decimal max)
{
decimal number = Convert.ToDecimal(textBox.Text);
if (number < min || number > max)
{
MessageBox.Show(textBox.Tag + " must be between " + min
+ " and " + max + ".", Title);
textBox.Focus();
return false;
}
return true;
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace InventoryMaintenance
{
public class InvItem
{
private int itemNo;
private string description;
private decimal price;
public InvItem()
{
}
public InvItem(int itemNo, string description, decimal price)
{
this.itemNo = itemNo;
this.description = description;
this.price = price;
}
public int ItemNo
{
get
{
return itemNo;
}
set
{
itemNo = value;
}
}
public string Description
{
get
{
return description;
}
set
{
description = value;
}
}
public decimal Price
{
get
{
return price;
}
set
{
price = value;
}
}
public string GetDisplayText() => itemNo + " " + description + " (" + price.ToString("c") + ")";
}
}
Modify a list class to use an indexer, a delegate, an event, and operators In this exercise, you'll modify a class that stores a list of inventory items so it uses an indexer, a delegate, an event, and operators. Then, you'll modify the code for the Inventory Maintenance form so it uses these features. Open the project and review the code 1. Extract the provided start project (W05 Exercise Start.zip), and open the InventoryMaintenance project. This is an enhanced version of the Inventory Maintenance application from W4 Exercise that uses a list class. 2. Review the code for the InvitemList class so you understand how it works. Then, review the code for the Inventory Maintenance form to see how it uses this class. Finally, run the application to see how it works. Add an index to the InvitemList class 3. Delete the GetItemByIndex method from the InvitemList class, and replace it with an indexer that receives an int value. This indexer should include both get and set accessors, and the get accessor should check that the value that's passed to it is a valid index. If the index isn't valid, the accessor should throw an Argument OutOfRange Exception with a message that consists of the index value. 4. Modify the Invoice Maintenance form to use this indexer instead of the GetItemByIndex method. Then, test the application to be sure it still works.
Step by Step Solution
3.35 Rating (158 Votes )
There are 3 Steps involved in it
Step: 1
using System using SystemCollectionsGeneric public class InvItem public string Name get set pub...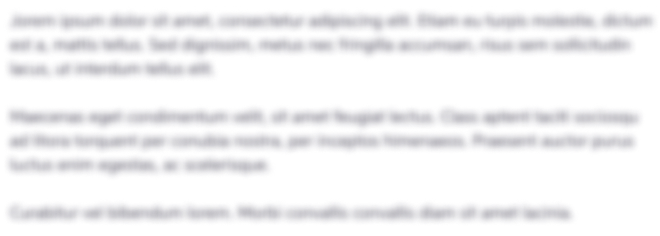
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started