Question
here is my class Fraction public class Fraction { private int num; private int denom; public Fraction() { num = 0; denom = 1; }
here is my class Fraction
public class Fraction { private int num; private int denom;
public Fraction() { num = 0; denom = 1;
}
public Fraction(int n, int d) { int gcd; if (n != 0) { num = n; denom = d; gcd = getGCD(); if (d < 0) { num = -n / gcd; denom = -d / gcd; } else { num = n / gcd; denom = d / gcd; } } else { num = 0; denom = 1; } }
public Fraction add(Fraction f) { return new Fraction(num * f.denom + denom * f.num, denom * f.denom); }
public Fraction subtract(Fraction f) { return new Fraction(num * f.denom - denom * f.num, denom * f.denom);
}
public Fraction multiply(Fraction f) { return new Fraction(num * f.num, denom * f.denom);
}
public Fraction divide(Fraction f) { return new Fraction(num * f.denom, denom * f.num);
}
public int getNum() { return num;
}
public int getDenom() { return denom;
}
public void setNum(int n) { int gcd; if (n != 0) { num = n; gcd = getGCD(); num /= gcd; denom /= gcd; } else { num = 0; denom = 1; } }
public void setDenom(int d) { int gcd; if (num != 0) { denom = d; gcd = getGCD(); if (denom < 0) { num = -num / gcd; denom = -denom / gcd; } else { num = num / gcd; denom = denom / gcd; } } else { denom = 1; } }
public void print() { System.out.println(" num: " + num + " denom: " + denom);
}
public void update(int n, int d) { int gcd; if (n != 0) { num = n; denom = d; gcd = getGCD(); if (d < 0) { num = -n / gcd; denom = -d / gcd; } else { num = n / gcd; denom = d / gcd; } } else { num = 0; denom = 1; } }
public int[] getCommonDigit() { int[] returnAry; int[] numDigitAry; int[] denomDigitAry; int tmp; int size = 0; tmp = num < 0 ? -num : num; numDigitAry = new int[10]; do { numDigitAry[tmp % 10] = 1; tmp /= 10; } while (tmp != 0); tmp = denom; denomDigitAry = new int[10]; do { denomDigitAry[tmp % 10] = 1; tmp /= 10; } while (tmp != 0); for (int i = 0; i < 10; i++) { if (numDigitAry[i] == 1 && denomDigitAry[i] == 1) { size++; } } returnAry = new int[size]; if (size != 0) { for (int i = 0, j = 0; i < 10; i++) { if (numDigitAry[i] == 1 && denomDigitAry[i] == 1) { returnAry[j] = i; j++; } } } return returnAry; }
protected final int getGCD(int a, int b) { if (b != 0) return getGCD(b, a % b); else return a; }
protected final int getGCD() { int a = num < 0 ? -num : num; int b = denom < 0 ? -denom : denom; int tmp; while (b != 0) { tmp = a % b; a = b; b = tmp; } return a; } -------------------------
A. Update the Fraction class given in the Lecture notes as needed.
B. Create the Point class as required below and update as needed
/**
* Program Name: Point.java
* Discussion: Points and operations
* Date:
*/
class Point {
private Fraction fx;
private Fraction fy;
// Constructors
// Operations
// Displaying
}
D. Write a menu program to first display the exercise information and output as below,
*******************************
* MENU Hw #5 *
* 1. Initializing (2 Points) *
* 2. Placement *
* 3. Moving *
* 4. Flipping *
* 5. Displaying *
* 6. Quit *
*******************************
Select an option (use integer value only): 2
Not a proper call as no Points are available!
*******************************
* MENU Hw #5 *
* 1. Initializing (2 Points) *
* 2. Placement *
* 3. Moving *
* 4. Flipping *
* 5. Displaying *
* 6. Quit *
*******************************
Select an option (use integer value only): 1
Initializing Option --
***************************
* Sub MENU INITIALIZING *
* 1. Creating *
* 2. Updating *
* 3. Returning *
***************************
Select an option (integer only): 2
Not a proper call as no Points are available!
***************************
* Sub MENU INITIALIZING *
* 1. Creating *
* 2. Updating *
* 3. Returning *
***************************
Select an option (integer only): 1
//Enter proper data to build Point objects
***************************
* Sub MENU INITIALIZING *
* 1. Creating *
* 2. Updating *
* 3. Returning *
***************************
Select an option (integer only): 3
Returning to MENU Hw#5
*******************************
* MENU Hw #5 *
* 1. Initializing (2 Points) *
* 2. Placement *
* 3. Moving *
* 4. Flipping *
* 5. Displaying *
* 6. Quit *
*******************************
Select an option (use integer value only): 5
Printing Option --
// Displaying proper values & formats!
*******************************
* MENU Hw #5 *
* 1. Initializing (2 Points) *
* 2. Placement *
* 3. Moving *
* 4. Flipping *
* 5. Displaying *
* 6. Quit *
*******************************
Select an option (use integer value only): 2
Placement Option -
The given Point objects are as follows,
// Assuming the Point objects are as below
Point #1: (1/2, 2/1)
Point #2: (4/1, 1/1)
Which Point to be checked against (1 or 2)? 3
Wrong option!
Which Point to be checked against (1 or 2)? 1
With respective to Point #1, Point #2 is in Quadrant #4!
*******************************
* MENU Hw #5 *
* 1. Initializing (2 Points) *
* 2. Placement *
* 3. Moving *
* 4. Flipping *
* 5. Displaying *
* 6. Quit *
*******************************
Select an option (use integer value only): 2
Placement Option -
The given Point objects are as follows,
// Assuming the Point objects are as below
Point #1: (1/2, 2/1)
Point #2: (4/1, 1/1)
Which Point to be checked against (1 or 2)? 3
Wrong option!
Which Point to be checked against (1 or 2)? 2
With respective to Point #2, Point #1 is in Quadrant #2!
*******************************
* MENU Hw #5 *
* 1. Initializing (2 Points) *
* 2. Placement *
* 3. Moving *
* 4. Flipping *
* 5. Displaying *
* 6. Quit *
*******************************
Select an option (use integer value only): 3
Moving Option -
Which Point to be moved (1 or 2)? 3
Wrong option!
Which Point to be moved (1 or 2)? 1
*****************************
* Sub MENU -- MovingPoint *
* 1. By (frX, frY) *
* 2. By fr *
* 3. Printing *
* 4. Returning *
*****************************
Select an option (use integer value only): 1
// Providing proper values & steps to move!
*****************************
* Sub MENU -- MovingPoint *
* 1. By (frX, frY) *
* 2. By fr *
* 3. Printing *
* 4. Returning *
*****************************
Select an option (use integer value only): 3
// Displaying proper values & formats!
*****************************
* Sub MENU -- MovingPoint *
* 1. By (frX, frY) *
* 2. By fr *
* 3. Printing *
* 4. Returning *
*****************************
Select an option (use integer value only): 2
// Providing proper values & steps to move!
*****************************
* Sub MENU -- MovingPoint *
* 1. By (frX, frY) *
* 2. By fr *
* 3. Printing *
* 4. Returning *
*****************************
Select an option (use integer value only): 3
// Displaying proper values & formats!
*****************************
* Sub MENU -- MovingPoint *
* 1. By (frX, frY) *
* 2. By fr *
* 3. Printing *
* 4. Returning *
*****************************
Select an option (use integer value only): 4
Returning to MENU Hw#5
*******************************
* MENU Hw #5 *
* 1. Initializing (2 Points) *
* 2. Placement *
* 3. Moving *
* 4. Flipping *
* 5. Displaying *
* 6. Quit *
*******************************
Select an option (use integer value only): 4
Flipping Option -
Which Point to be flipped (1 or 2)? 3
Wrong option!
Which Point to be flipped (1 or 2)? 2
****************************
* Sub MENU - FlippingPoint *
* 1. By Y *
* 2. By X *
* 3. By Origin *
* 4. Printing *
* 5. Returning *
****************************
Select an option (use integer value only): 1
// Providing proper values & steps!
****************************
* Sub MENU - FlippingPoint *
* 1. By Y *
* 2. By X *
* 3. By Origin *
* 4. Printing *
* 5. Returning *
****************************
Select an option (use integer value only): 4
// Displaying proper values & formats!
****************************
* Sub MENU - FlippingPoint *
* 1. By Y *
* 2. By X *
* 3. By Origin *
* 4. Printing *
* 5. Returning *
****************************
Select an option (use integer value only): 2
// Providing proper values & steps!
****************************
* Sub MENU - FlippingPoint *
* 1. By Y *
* 2. By X *
* 3. By Origin *
* 4. Printing *
* 5. Returning *
****************************
Select an option (use integer value only): 3
// Providing proper values & steps!
****************************
* Sub MENU - FlippingPoint *
* 1. By Y *
* 2. By X *
* 3. By Origin *
* 4. Printing *
* 5. Returning *
****************************
Select an option (use integer value only): 4
// Displaying proper values & formats!
****************************
* Sub MENU - FlippingPoint *
* 1. By Y *
* 2. By X *
* 3. By Origin *
* 4. Printing *
* 5. Returning *
****************************
Select an option (use integer value only): 5
Returning to MENU Hw #5
*******************************
* MENU Hw #5 *
* 1. Initializing (2 Points) *
* 2. Placement *
* 3. Moving *
* 4. Flipping *
* 5. Displaying *
* 6. Quit *
*******************************
Select an option (use integer value only): 6
Having Fun ...
Hint!
You should at least test your program with the information given below (used as pairs).
Point #1: (1/2, 2/1)
Point #2: (4/1, 1/1)
Point #3: (-1/1, -1/2)
Point #4: (2/1, -2/1)
1. Reminder!
In your program, no GLOBAL DATA are allowed, and you must write all needed functions (no package methods are allowed Except for input/ouput methods).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
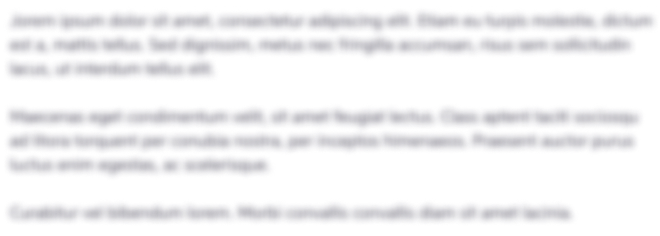
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started