Here is my code and instructions: I am getting an error, please let me know how to
Question:
Here is my code and instructions: I am getting an error, please let me know how to fix it:
class Bear {
String name; //iniatiation
int height;
double speed;
public Bear( String name, int height, double speed ) {
this.name = name; // name assignment
this.height = height; // height assignment
this.speed = speed; // speed assignment
}
public void climbTree() {
System.out.println("Bear "+name+" climbs a tree"); // ui
}
public String toString() {
return name+ height+speed;
}
}
____________________________
import java.util.Scanner;
public class Bears {
private Bear[] myBears; //array of Bear
public Bears(int length) {
this.myBears = new Bear[length];
}
public void makeBears() {
String name;
int height;
double speed;
Scanner input = new Scanner(System.in);
System.out.println("Enter number of bears:"); // user input
int n = Integer.parseInt(input.nextLine());
for (int i = 0; i
System.out.println("Enter name of the bear:"); // user input
name = input.nextLine();
System.out.println("Enter height of the bear (integer):"); // user input
height = Integer.parseInt(input.nextLine());
System.out.println("Enter speed of the bear (double):"); // user input
speed = Double.parseDouble(input.nextLine());
Bear b = new Bear(name, height, speed); // setting
myBears[i] = b;
}
input.close();
}
public void allClimbTree() {
for (Bear b : myBears) {
b.climbTree();
}
}
}
____________________________
public class TestBears { //test class
public static void main(String[] args){
Bears bears = new Bears(5);
bears.makeBears();
bears.allClimbTree();
}
_____________
Output:
Exception in thread "main" java.lang.NullPointerException: Cannot invoke "Bear.climbTree()" because "b" is null
at Bears.allClimbTree(Bears.java:62)
at TestBears.main(TestBears.java:12)
________
Lab Instructions:
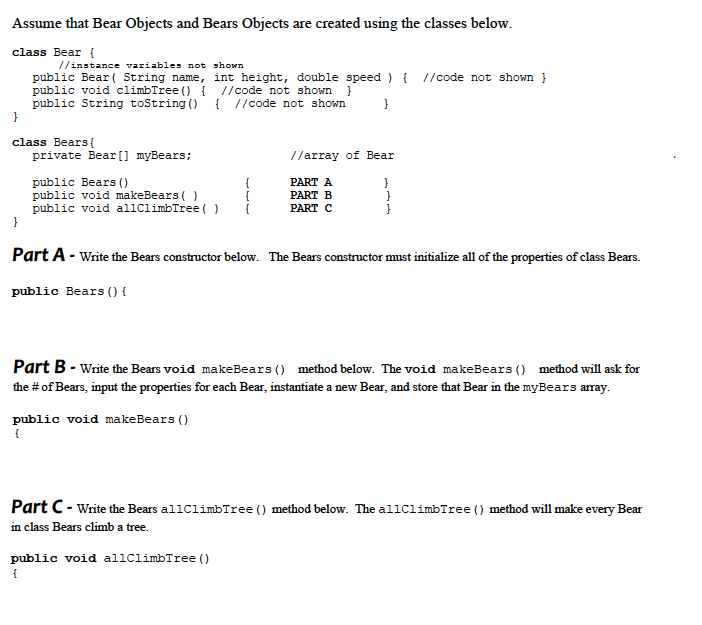
Applying Communication Theory For Professional Life A Practical Introduction
ISBN: 9781506315478
4th Edition
Authors: Marianne Dainton, Elaine D. Zelley