Question
Here is my iOS bluetooth smartphone application code for the main view controller: import UIKit import CoreBluetooth let heartRateServiceCBUUID = CBUUID(string: 0x180D) let heartRateMeasurementCharacteristicCBUUID =
Here is my iOS bluetooth smartphone application code for the main view controller:
import UIKit
import CoreBluetooth
let heartRateServiceCBUUID = CBUUID(string: "0x180D")
let heartRateMeasurementCharacteristicCBUUID = CBUUID(string: "2A37")
let bodySensorLocationCharacteristicCBUUID = CBUUID(string: "2A38")
extension ViewController: CBCentralManagerDelegate {
func centralManagerDidUpdateState(_ central: CBCentralManager) {
switch central.state {
case .unknown:
print("central.state is .unknown")
case .resetting:
print("central.state is .resetting")
case .unsupported:
print("central.state is .unsupported")
case .unauthorized:
print("central.state is .unauthorized")
case .poweredOff:
print("central.state is .poweredOff")
case .poweredOn:
print("central.state is .poweredOn")
centralManager.scanForPeripherals(withServices: [heartRateServiceCBUUID])
}
}
func centralManager(_ central: CBCentralManager, didDiscover peripheral: CBPeripheral, advertisementData: [String : Any], rssi RSSI: NSNumber) {
print(peripheral)
heartRatePeripheral = peripheral
///heartRatePeripheral.delegate = self
centralManager.stopScan()
centralManager.connect(heartRatePeripheral)
}
func centralManager(_ central: CBCentralManager, didConnect peripheral: CBPeripheral) {
func centralManager(_ central: CBCentralManager, didConnect peripheral: CBPeripheral) {
print("Connected!")
heartRatePeripheral.discoverServices([heartRateServiceCBUUID])
}
}
}
class ViewController: UIViewController {
var centralManager: CBCentralManager!
var heartRatePeripheral: CBPeripheral!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
centralManager = CBCentralManager(delegate: self, queue: nil)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
I have another view controller page that sends values when buttons are pushed to the bluetooth chip. Here is the code for that:
import UIKit
import CoreBluetooth
class TemperatureViewController: UIViewController {
var _peripheral: CBPeripheral?
var _characteristics: [CBCharacteristic]?
func peripheral(peripheral: CBPeripheral, didDiscoverCharacteristicsForService service: CBService, error:NSError?)
{
print("Found \(service.characteristics!.count) characteristics!: \(String(describing: service.characteristics))")
_peripheral = peripheral
_characteristics = service.characteristics
let string = "0"
let data = string.data(using: String.Encoding.utf8)
for characteristic in service.characteristics as [CBCharacteristic]!
{
if(characteristic.uuid.uuidString == "2A38")
{
print("sending data")
peripheral.writeValue(data!, for: characteristic,type: CBCharacteristicWriteType.withoutResponse)
}
}
}
@IBAction func offButtonTouch(_ sender: UIButton) {
var value: UInt8 = 0
let data = NSData(bytes: &value, length: MemoryLayout
for characteristic in _characteristics as [CBCharacteristic]!
{
if(characteristic.uuid.uuidString == "2A38")
{
func writeValue(_ data: Data, for characteristic: CBCharacteristic,type:CBCharacteristicWriteType){
}
}
}
}
@IBAction func lowButtonTouch(_ sender: UIButton) {
var value: UInt8 = 1
let data = NSData(bytes: &value, length: MemoryLayout
for characteristic in _characteristics as [CBCharacteristic]!
{
if(characteristic.uuid.uuidString == "2A38")
{
func writeValue(_ data: Data, for characteristic: CBCharacteristic,type:CBCharacteristicWriteType){
}
}
}
}
@IBAction func highButtonTouch(_ sender: UIButton) {
var value: UInt8 = 2
let data = NSData(bytes: &value, length: MemoryLayout
for characteristic in _characteristics as [CBCharacteristic]!
{
if(characteristic.uuid.uuidString == "2A38")
{
func writeValue(_ data: Data, for characteristic: CBCharacteristic,type:CBCharacteristicWriteType){
}
}
}
}
}
Why does the app automatically send a 2 when the connection is made?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
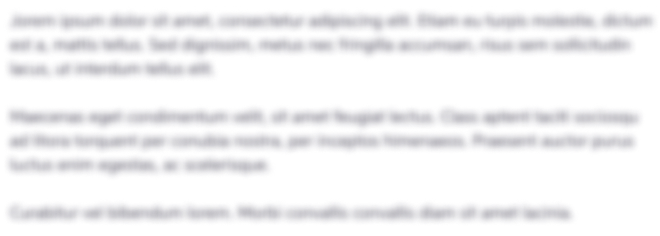
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started