Question
Here is shell.c: #define _GNU_SOURCE //this is needed to be able to use execvpe #include #include #include #include #include #include #include #include typedef struct {
Here is shell.c:
#define _GNU_SOURCE //this is needed to be able to use execvpe #include
typedef struct { char* binary_path; char* stdin; char* stdout; char* stderr; char* arguments; char* extra_environment; short use_path; short copy_environment; short niceness; short wait; unsigned int timeout; } command;
//function prototypes void print_parsed_command(command); short parse_command(command*, char*);
//global variables here
short getlinedup(FILE* fp, char** value){ char* line = NULL; size_t n = 0; //get one line int ret = getline(&line, &n, fp);
if(ret == -1){ //the file ended return 0; } //remove at the end line[strcspn(line, " ")] = '\0'; //duplicate the string and set value *value = strdup(line); free(line);
return 1; }
//parse a command_file and set a corresponding command data structure short parse_command(command* parsed_command, char* cmdfile){ FILE* fp = fopen(cmdfile, "rb"); if(!fp){ //the file does not exist return 0; }
char* value; short ret; int intvalue;
ret = getlinedup(fp,&value); if(!ret){ fclose(fp); return 0; } parsed_command->binary_path = value;
ret = getlinedup(fp,&value); if(!ret){ fclose(fp); return 0; } parsed_command->stdin = value;
ret = getlinedup(fp,&value); if(!ret){ fclose(fp); return 0; } parsed_command->stdout = value;
ret = getlinedup(fp,&value); if(!ret){ fclose(fp); return 0; } parsed_command->stderr = value;
ret = getlinedup(fp,&value); if(!ret){ fclose(fp); return 0; } parsed_command->arguments = value;
ret = getlinedup(fp,&value); if(!ret){ fclose(fp); return 0; } parsed_command->extra_environment = value;
ret = getlinedup(fp,&value); if(!ret){ fclose(fp); return 0; } intvalue = atoi(value); if(intvalue != 0 && intvalue != 1){ fclose(fp); return 0; } parsed_command->use_path = (short)intvalue;
ret = getlinedup(fp,&value); if(!ret){ fclose(fp); return 0; } intvalue = atoi(value); if(intvalue != 0 && intvalue != 1){ fclose(fp); return 0; } parsed_command->copy_environment = (short)intvalue;
ret = getlinedup(fp,&value); if(!ret){ fclose(fp); return 0; } intvalue = atoi(value); if(intvalue 19){ fclose(fp); return 0; } parsed_command->niceness = (short)intvalue;
ret = getlinedup(fp,&value); if(!ret){ fclose(fp); return 0; } intvalue = atoi(value); if(intvalue != 0 && intvalue != 1){ fclose(fp); return 0; } parsed_command->wait = (short)intvalue;
ret = getlinedup(fp,&value); if(!ret){ fclose(fp); return 0; } intvalue = atoi(value); if(intvalue timeout = (unsigned int)intvalue;
return 1; }
//useful for debugging void print_parsed_command(command parsed_command){ printf("---------- "); printf("binary_path: %s ", parsed_command.binary_path); printf("stdin: %s ", parsed_command.stdin); printf("stdout: %s ", parsed_command.stdout); printf("stderr: %s ", parsed_command.stderr); printf("arguments: %s ", parsed_command.arguments); printf("extra_environment: %s ", parsed_command.extra_environment); printf("use_path: %d ", parsed_command.use_path); printf("copy_environment: %d ", parsed_command.copy_environment); printf("niceness: %d ", parsed_command.niceness); printf("wait: %d ", parsed_command.wait); printf("timeout: %d ", parsed_command.timeout); }
void free_command(command cmd){ free(cmd.binary_path); free(cmd.stdin); free(cmd.stdout); free(cmd.stderr); free(cmd.arguments); free(cmd.extra_environment); }
int main(int argc, char *argv[], char* env[]) {
for(int ncommand=1; ncommand //may be useful for debugging //print_parsed_command(parsed_command); //process_command(...); /* process_command will: - get a parsed_command variable - create a child process - set file redirection, niceness, arguments, envirionment variables, ... - call a proper variant of execv - print when a child process is created and when any child process is terminated - if necessary, wait for the termination of the program */ free_command(parsed_command); } //remember to wait for the termination of all the child processes, regardless of the value of parsed_command.wait }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
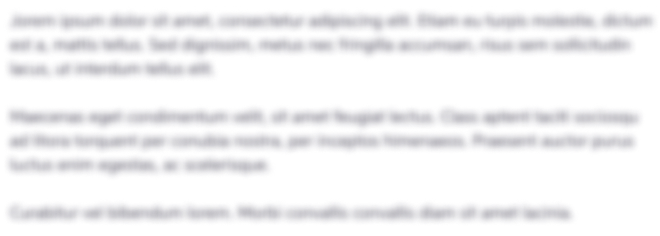
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started