Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Here is the code from the previous assignment and this should remove any confusion about node: LinkedList.java: public class LinkedList { Node head; Node tail;
Here is the code from the previous assignment and this should remove any confusion about node:
LinkedList.java:
public class LinkedList{ Node head; Node tail; public class Node { E data; Node next; public Node(E element) { data = element; next = null; } @Override public String toString() { return String.valueOf(this.data); } } public void add(int index, E element) { Node temp1 = head; while (--index > 0) temp1 = temp1.next; Node temp = new Node(element); temp.next = temp1.next; temp1.next = temp; } public void addFirst(E element) { Node temp = new Node(element); if (head == null) { tail = temp; } temp.next = head; head = temp; } public void addLast(E element) { Node temp = new Node(element); if (tail == null) { tail = temp; head = temp; } else { tail.next = temp; tail = temp; } } public E getFirst() { return head.data; } public E getLast() { return tail.data; } public void remove(int index) { Node temp1 = head; while (--index > 0) temp1 = temp1.next; temp1.next = temp1.next.next; } public E removeFirst() { E data = head.data; head = head.next; return data; } public E removeLast() { E data = tail.data; Node temp = head; while (temp.next != tail) { temp = temp.next; } temp.next = null; tail = temp; return data; } public int size() { int count = 0; Node temp = head; while (temp != null) { temp = temp.next; count++; } return count; } @Override public String toString() { Node temp = head; String str = "["; while (temp.next != null) { str = str + temp.data + ","; temp = temp.next; } str = str + temp.data; return str + "]"; } }
ListTestYourName.java:
import java.util.Scanner; public class ListTestYourName { public static void main(String[] args) { int option; Scanner keyboardInput = new Scanner(System.in); // declare and initialize LinkedList object LinkedListlist = new LinkedList(); // continue to get user choice until the option is 0 do { // display menu System.out.println("----- Linked List Test Program -----"); System.out.println("0 - Exit Program"); System.out.println("1 - Add First Node"); System.out.println("2 - Add Last Node"); System.out.println("3 - Add at Index"); System.out.println("4 - Remove First Node"); System.out.println("5 - Remove Last Node"); System.out.println("6 - Remove at Index"); System.out.println("7 - Print List Size"); System.out.print("Select option: "); // get user option option = keyboardInput.nextInt(); // option check switch (option) { // check addFirstNode method case 1: System.out.println("Testing method addFirstNode()"); // get the element to be inserted System.out.print("Enter the first node element: "); int nodeFirstElement = keyboardInput.nextInt(); // call addFirst method list.addFirst(nodeFirstElement); // print the list after insertion System.out.println("List contents after adding first node is : " + list.toString()); break; // check addLast method case 2: System.out.println("Testing method addLastNode()"); // get the element to be inserted System.out.print("Enter the last node element: "); int nodeLastElement = keyboardInput.nextInt(); // call addLast method list.addLast(nodeLastElement); // print the list after insertion System.out.println("List contents after adding last node is : " + list.toString()); break; // check add method case 3: System.out.println("Testing method add()"); // get the index at which it should be inserted System.out.print("Enter the index in which element to be inserted: "); int index = keyboardInput.nextInt(); // get the element to be inserted System.out.print("Enter the node element to be inserted: "); int nodeElement = keyboardInput.nextInt(); // call add method with index and element list.add(index, nodeElement); // print the list after insertion System.out.println("List contents after adding node at given index is : " + list.toString()); break; // check removeFirst method case 4: // when there is no element in the list if (list.size() == 0) { System.out.println("List is Empty now."); } else { // if there is element in list System.out.println("List contents before removing first node is: " + list.toString()); list.removeFirst(); System.out.println("List contents after removing first node is : " + list.toString()); } break; // check removeLast method case 5: // when there is no element in the list if (list.size() == 0) { System.out.println("List is Empty now."); } else { // if there is element in list System.out.println("List contents before removing last node is: " + list.toString()); list.removeLast(); System.out.println("List contents after removing last node is : " + list.toString()); } break; // check remove method case 6: if (list.size() == 0) { System.out.println("List is Empty now."); } else { // if there is element in list get index at which the removed System.out.println("Enter the index of the node to be removed: "); int indexRemove = keyboardInput.nextInt(); // print the list contents before removal System.out.println( "List contents before removing node at index " + indexRemove + " is: " + list.toString()); // call remove method list.remove(indexRemove); // print the list contents after removal System.out.println( "List contents after removing node at index " + indexRemove + " is: " + list.toString()); } break; // display the size of the list case 7: System.out.println("Size of the list is: " + list.size()); break; // terminate the program case 0: System.out.println("Good Bye!!!"); break; default: System.out.println("Invalid choice!!!"); break; } } while (option != 0); keyboardInput.close(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
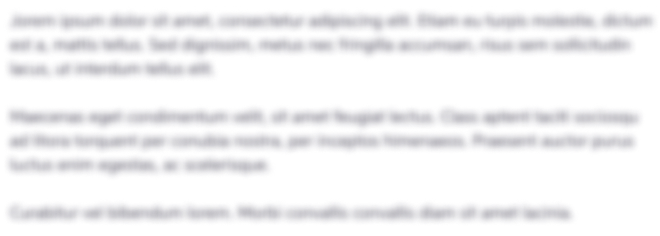
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started