Question
Here is the description: Your program first reads some inventory data from a file named p1data.txt, creates a dynamically allocated part record object for each
Here is the description:
Your program first reads some inventory data from a file named p1data.txt, creates a dynamically allocated part record object for each part, with its address stored in the next position (starting from index 0) in a dynamically allocated pointer array data structure. Since you do not know how many parts to expect, you have to assume a large enough size initially (e.g., 50 or whatever). The program then prints out the data in the same order in which it was entered, which we call the original/chronological order.
So pretty much all I need is to make it so I can read the data from the file and put it into a dynamic array of part record pointers. I have exhausted all other option sin regards of researching this so here I am.
THIS IS WHAT A SAMPLE OF THE INPUT FILE LOOKS LIKE:
40 32.99 30000
30 17.99 70000
15 4.95 20000
20 28.99 60000
25 39.79 10000
VECTORS CANNOT BE USED
HERE IS THE CODE THAT IS PROVIDED
From what I have gathered the only real things that need adjusting is readdata. Just needs to gathe rthe input into the proper dynaic array that I guess has to be made then outputs that data in chronological order as it was gathered.
Any help would be extremely appreciated.
#include
// Type delcarations - NO MEMORY ALLOCATED (EVEN STATICALLY) struct Part /*A Part record*/ { int ID; float Price; int Quantity; }; typedef Part * Partptr; // Part record pointer type; The type Partptr becomes synonymous with Part * typedef Partptr* Index; // The type Index becomes synonymous with Partptr *
int main () { /* Rudimentary scope control in C/C++ */ void readdata (Index &, int &); void printdata (Index, int); void copyindex (Index, Index &, int); void sortbyID (Index, int); void sortbyPrice (Index, int); void deallocate (Index &, int &); Index DBindex, IDindex, Priceindex; int dbsize;
readdata (DBindex, dbsize); cout << "Original data:" << endl; printdata (DBindex, dbsize); copyindex (DBindex, IDindex, dbsize); sortbyID (IDindex, dbsize); cout << "Data sorted by the ID:" << endl; printdata (IDindex, dbsize); copyindex (DBindex, Priceindex, dbsize); sortbyPrice (Priceindex, dbsize); cout << "Data sorted by the price:" << endl; printdata (Priceindex, dbsize); cout << "Data in the original chronological order:" << endl; printdata (DBindex, dbsize); cout << "Data sorted by the ID:" << endl; printdata (IDindex, dbsize); deallocate (DBindex, dbsize); if (IDindex != nullptr) delete IDindex; if (Priceindex != nullptr) delete Priceindex; };
void eat_white (ifstream & in) /* Pre: "in" is a valid input file stream. Post: Any white space skipped over to EOF or the next non-white space character.*/ { while ((in.peek()!=EOF) && isspace(in.peek())) in.ignore(); };
void resize (Index & Base, int OLDSIZE, int NEWSIZE) /* Pre: Base is a (dynamic) Index of size OLDSIZE. Post: Base is "EXPANDED" OR "SHRUNK" into a new (dynamic) Index of size NEWSIZE, with data copied appropriately. IMPORTANT NOTE: If being shrunk, it is assumed that there are only NEWSIZE valid elements in the original array, and only that many are copied.*/ { // STUDENT IS TO FILL THIS IN (NEEDED ONLY FOR BONUS CREDIT). }
void readdata (Index & ptrs, int & size) /*Pre: ptrs is a pointer that could point to a dynamic array of Part record pointers. Post: ptrs points to a dynamic pointer array of the right size based on the input read in from the data file named "p1data". All the elements of the dynamic array are filled with pointers to dynamically allocated Part records, which in turn are filled with input data.*/ { void resize (Index &, int, int);
// STUDENT IS TO FILL THIS IN
};
void printrec (Part rec) /*Pre: rec has data. Post: The data in rec printed out.*/ { cout << rec.ID << ' ' << rec.Price << ' ' << rec.Quantity << endl; };
void printdata (Index ptrs, int size) /*Pre: The first "size" elements of the array pointed at by ptrs are Part records containing data. Post: They are all printed out in the order pointed at.*/ { void printrec (Part); for (int i=0; i void copyindex (Index orig, Index & copy, int size) /*Pre: The first "size" elements of the array pointed at by orig are Part records containing data. Post: copy points to a newly allocated dynamic array of pointers (with size elements), and the elements of this array are copies of the first size elements of orig.*/ { // STUDENT IS TO FILL THIS IN }; int selectsmallestID (Index ptrs, int first, int last) /*Pre: "first" through "last" elements of the ptrs array are pointers to Part records containing data. Post: A value k is returned such that, for i ranging from first to last, the relation ptrs[k]->ID <= ptrs[i]->ID holds.*/ { // STUDENT IS TO FILL THIS IN; YOU'D NEED TO CHANGE THE FOLLOWING return STATEMENT, TOO. return 0; }; void sortbyID (Index ptrs, int size) /*Pre: The first "size" elements of the ptrs array are pointers to Part records containing data. Post: for i ranging from 1 to size-2, the relation ptrs[i]->ID <= ptrs[i+1]->ID holds. ONLY POINTERS ARE MOVED. The Part records THEMSELVES ARE NOT DISTURBED OR MOVED AROUND.*/ { int selectsmallestID (Index, int, int); // STUDENT IS TO FILL THIS IN }; int selectsmallestPrice (Index ptrs, int first, int last) /*Pre: "first" through "last" elements of the ptrs array are pointers to Part records containing data. Post: A value k is returned such that, for i ranging from first to last, the relation ptrs[k]->Price <= ptrs[i]->Price holds.*/ { // STUDENT IS TO FILL THIS IN; YOU'D NEED TO CHANGE THE FOLLOWING return STATEMENT, TOO. return 0; }; void sortbyPrice (Index ptrs, int size) /*Pre: The first "size" elements of the ptrs array are pointers to Part records containing data. Post: for i ranging from 1 to size-2, the relation ptrs[i]->Price <= ptrs[i+1]->Price holds. ONLY POINTERS ARE MOVED. The Part records THEMSELVES ARE NOT DISTURBED OR MOVED AROUND.*/ { int selectsmallestPrice (Index, int, int); // STUDENT IS TO FILL THIS IN }; void deallocate (Index & ptrs, int & size) /*Pre: ptrs is a pointer pointing to an allocated dynamic array of "size" Part record pointers. Post: ptrs points to a dynamic pointer array. The "size" elements of the dynamic array, filled with pointers to dynamically allocated Part records, are now deallocated, and then the array of pointers that ptrs points to is itself deallocated, with ptrs reset to nullptr and size reset to 0.*/ { for (int i=0; i
Step by Step Solution
There are 3 Steps involved in it
Step: 1
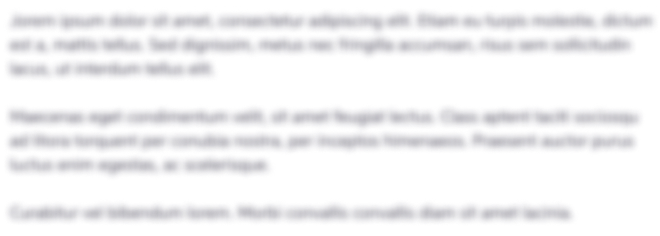
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started